Responsive or adaptable web design is a design methodology in which the appearance of the web page adapts to the resolution of the device with which it is being viewed, so we can have a unique design for computers, tablets or mobiles.
To implement a responsive design we can make use of the media queries, which is a module introduced in CSS3 that allows you to specify different CSS rules depending on whether some or other conditions are met, although the most common is to use them to apply rules according to the size of the screen. With the following code, for example, we would establish a blue background color for the page in widths less than 576px and red in larger sizes:
body { background-color:blue } @media (min-width: 768px) { body { background-color: red } }
Bootstrap uses media queries to define 5 breakpoints that depend on the width of the device's screen.
We have screens with extra small size, like phones in vertical orientation, whose classes use the suffix xs of extra small (less than 576px); small, like phones in horizontal orientation, with suffix sm of small (less than 768px); medium, like tablets, with suffix md, of medium (less than 992px); big, like PC screens, with suffix lg, of large (less than 1200px) and extra large, with suffix xl of extra large (more than 1200px).
Bootstrap Installation
If we only want to use Boostrap to create a layout, we can simply include the css, which only includes the grid system and the Flex utilities. But normally we want to use other styles, so we include bootstrap.min.css inside the <head>, either by downloading the files from the Bootstrap page, or from the Bootstrap CDN:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">
Containers
The first thing we have to define is a container using the classes container or container-fluid. With container-fluid we can create containers with a width of 100%. This is the CSS that defines this class in Bootstrap 4:
.container-fluid { width: 100%; padding-right: 15px; padding-left: 15px; margin-right: auto; margin-left: auto; }
On the other hand, with container we create centered containers, with a fixed width. The maximum width of this container depends on the width of the screen. For example, if the window is at least 576px wide but less than 768px, the maximum width of the container will be 540px, because it would apply the first media query, in addition to the main rule:
.container { width: 100%; padding-right: 15px; padding-left: 15px; margin-right: auto; margin-left: auto; } @media (min-width: 576px) { .container { max-width: 540px; } } @media (min-width: 768px) { .container { max-width: 720px; } } @media (min-width: 992px) { .container { max-width: 960px; } } @media (min-width: 1200px) { .container { max-width: 1140px; } }
Rows
Within the containers we will have rows, which are represented by the row class, and which are really containers of the CSS or flexbox Flexible Box Model, a specification that greatly facilitates creating designs that change dynamically when the screen resolution changes.
<div class="container"> <div class="row"> ... </div> </div>
The use of flexbox is one of the great novelties of Bootstrap 4 with respect to the previous version, in which div containers and the float property were used to define the design. A disadvantage of flexbox, as always, is the support in older versions of browsers, so if you need to support Internet Explorer 8 or Internet Explorer 9, and although there are hacks to add support, it may be advisable to continue using Bootstrap 3.
If we consult the CSS of Bootstrap we will see that these rows are defined as boxes or flexible containers using the property display: flex. The flex-wrap: wrap property also indicates that your children can flow on several lines if they don't have enough space, instead of having to stay on the same line, which is the default value (flex-wrap: nowrap):
.row { display: -ms-flexbox; display: flex; -ms-flex-wrap: wrap; flex-wrap: wrap; margin-right: -15px; margin-left: -15px; }
Columns
Within the rows we have columns, for which we have a multitude of classes depending on the size we want to give them. These columns become flexible elements by the simple fact of being daughters of a flexible box.
To define our columns the simplest thing is to use the class col, which will do that all the columns have the same weight at the time of dividing the width. If we look at its CSS, we will see that it is thanks to the property flex-grow: 1, which indicates that all the elements with class col will have a weight of 1 unit:
.col { -ms-flex-preferred-size: 0; flex-basis: 0; -ms-flex-positive: 1; flex-grow: 1; max-width: 100%; }
In this example we have, centered, thanks to the class container, three rows of elements. These rows are divided into 1, 2 and 3 columns respectively, each of the same size as its sisters:
<div class="container text-white"> <div class="row"> <div class="col bg-primary">col</div> </div> <div class="row"> <div class="col bg-primary">col</div> <div class="col bg-danger">col</div> </div> <div class="row"> <div class="col bg-primary">col</div> <div class="col bg-danger">col</div> <div class="col bg-warning text-dark">col</div> </div> </div>

We can also use classes of the .col-num type to indicate how many parts (of the 12 total into which the row is divided) the element should occupy. For example, .col-1 indicates that we want a maximum width of 1/12 (8.333333%). Bootstrap, like many other frameworks, uses rows based on a weight of 12 units for the flexibility provided by the number of divisors it has: 12 can be divided exactly between 12, 6, 4, 3 and 2 columns.
.col-1 { -ms-flex: 0 0 8.333333%; flex: 0 0 8.333333%; max-width: 8.333333%; } .col-2 { -ms-flex: 0 0 16.666667%; flex: 0 0 16.666667%; max-width: 16.666667%; } .col-3 { -ms-flex: 0 0 25%; flex: 0 0 25%; max-width: 25%; } ....
The following example uses these numbered col classes to assign more space to some columns than to others:
<div class="container text-white"> <div class="row"> <div class="col-4 bg-primary">col-4</div> <div class="col-4 bg-danger">col-4</div> <div class="col-4 bg-warning text-dark">col-4</div> </div> <div class="row"> <div class="col-6 bg-primary">col-6</div> <div class="col-6 bg-danger">col-6</div> </div> <div class="row"> <div class="col-8 bg-primary">col-8</div> <div class="col-2 bg-danger">col-2</div> <div class="col-2 bg-warning text-dark">col-2</div> </div> </div>

If the total sum of the weights is more than 12, as the row class has the flex-wrap: wrap property, the columns will be shown in a new row. If they add less than 12, they simply will not fill the total width:
<div class="container text-white bg-dark"> <div class="row"> <div class="col-4 bg-primary">col-4</div> <div class="col-4 bg-danger">col-4</div> <div class="col-8 bg-warning text-dark">col-8</div> </div> <div class="row"> <div class="col-6 bg-primary">col-6</div> <div class="col-3 bg-danger">col-3</div> </div> </div>

Columns adaptable to resolution
If we want the weight of the columns to depend on the width of the device screen, we can use the breakpoints defined by Bootstrap. In this way, if we have a col-mum class, this will apply for extra small and upper screens; col-sm-mum for small and upper screens, col-md-mum for medium and upper screens, col-lg-mum for large and upper screens, and col-xl-mum for extra large screens.
For example, the following code defines that each column has a width of 25% (3/12) in extra small and small screens, 50% in medium screens and 100% in large or extra large screens:
<div class="container text-white"> <div class="row"> <div class="col-3 col-md-6 col-lg-12 bg-primary"> col-3 col-md-6 col-lg-12 </div> <div class="col-3 col-md-6 col-lg-12 bg-danger"> col-3 col-md-6 col-lg-12 </div> </div> </div>
If we only define the size of the columns for one of the breakpoints, the columns will occupy 100% before that breakpoint (so each column will appear in a subsequent line) and the size indicated from the breakpoint. In this example, both columns will be shown one below the other until they reach the average screen size, at which point they will be shown side by side, each occupying half of the screen:
<div class="container text-white"> <div class="row"> <div class="col-md-6 bg-primary">col-md-6</div> <div class="col-md-6 bg-danger">col-md-6</div> </div> </div>
We can also combine columns with weight (columns with col-num class) with columns without weight (columns with col class). In this case what would happen is that the indicated width would be given to the columns with weight, and the rest of the width would be distributed equitably between the columns:
<div class="container text-white"> <div class="row"> <div class="col bg-primary">col</div> <div class="col-2 bg-danger">col-2</div> <div class="col bg-warning text-dark">col</div> </div> </div>

Finally, if we want a column to fit its content, we can use the col-auto or col-breakpoint-auto classes.
<div class="container text-white"> <div class="row"> <div class="col-auto bg-primary">col-auto</div> <div class="col bg-danger">col</div> <div class="col bg-warning text-dark">col</div> </div> </div>

Margen
Para añadir margen izquierdo entre columnas, en lugar de crear columnas sin contenido, podemos añadir a una columna una clase del tipo offset-peso u offset-breakpoint-peso, cuyos sufijos de breakpoint funcionan de forma similar a los sufijos de las clases col-. En este caso el peso puede ir de 0 (ningún espacio, sólo en las clases con sufijo de breakpoint) a 11.
.offset-1 { margin-left: 8.333333%; } .offset-2 { margin-left: 16.666667%; } .offset-3 { margin-left: 25%; } ...
In this example we show an option to align two columns of equal width to each side of the screen, with an equal space in the middle:
<div class="container text-white"> <div class="row"> <div class="col-4 bg-primary">col-4</div> <div class="col-4 offset-4 bg-danger">col-4 offset-4</div> </div> </div>

Vertical alignment
We can align the columns vertically within the rows by means of classes that take advantage again of the model of flexible boxes of CSS, with classes of the style align-items-value.
align-items-start: aligns the columns to the top edge of the row (equivalent to using the property align-items: flex-start)
align-items-end: aligns the columns to the bottom edge of the row
align-items-center: aligns the columns to the center of the row
align-items-baseline: aligns the columns by the base lines of the texts they contain
align-items-stretch: default value; stretches columns to match row height
.align-items-start { -ms-flex-align: start !important; align-items: flex-start !important; } .align-items-end { -ms-flex-align: end !important; align-items: flex-end !important; } .align-items-center { -ms-flex-align: center !important; align-items: center !important; } .align-items-baseline { -ms-flex-align: baseline !important; align-items: baseline !important; } .align-items-stretch { -ms-flex-align: stretch !important; align-items: stretch !important; }
In this example we assign a height of 25% of the screen to the row to see how the columns align to the top when using align-items-start:
<div class="container text-white bg-dark"> <div class="row align-items-start h-25"> <div class="col bg-primary">col</div> <div class="col bg-danger">col</div> <div class="col bg-warning text-dark">col</div> </div> </div>
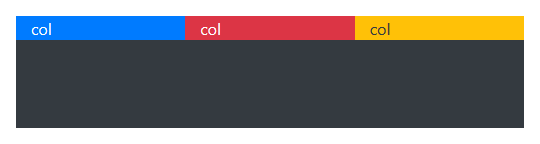
In this example the columns are aligned to the bottom of the row by the use of align-items-end.
<div class="container text-white bg-dark"> <div class="row align-items-end h-25"> <div class="col bg-primary">col</div> <div class="col bg-danger">col</div> <div class="col bg-warning text-dark">col</div> </div> </div>
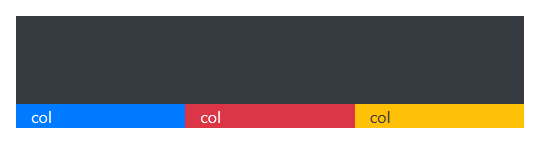
In this example the columns are aligned in the center when using align-items-center:
<div class="container text-white bg-dark"> <div class="row align-items-center h-25"> <div class="col bg-primary">col</div> <div class="col bg-danger">col</div> <div class="col bg-warning text-dark">col</div> </div> </div>
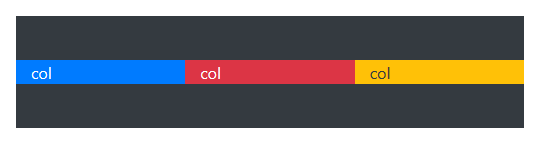
In this example the columns are aligned by the base lines of the texts they contain, according to the align-items-baseline class:
<div class="container text-white bg-dark"> <div class="row align-items-baseline h-25"> <div class="col bg-primary h1">col</div> <div class="col bg-danger h2">col</div> <div class="col bg-warning text-dark h3">col</div> </div> </div>
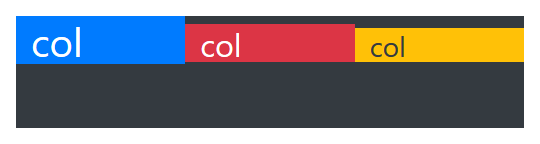
In this example the columns are stretched to adjust to the height defined for the row, thanks to align-items-stretch:
<div class="container text-white bg-dark"> <div class="row align-items-stretch h-25"> <div class="col bg-primary h1">col</div> <div class="col bg-danger h2">col</div> <div class="col bg-warning text-dark h3">col</div> </div> </div>
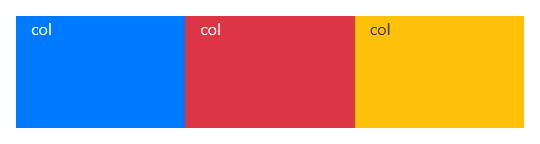
In the next post we will talk about the horizontal alignment and the nesting of the rows.