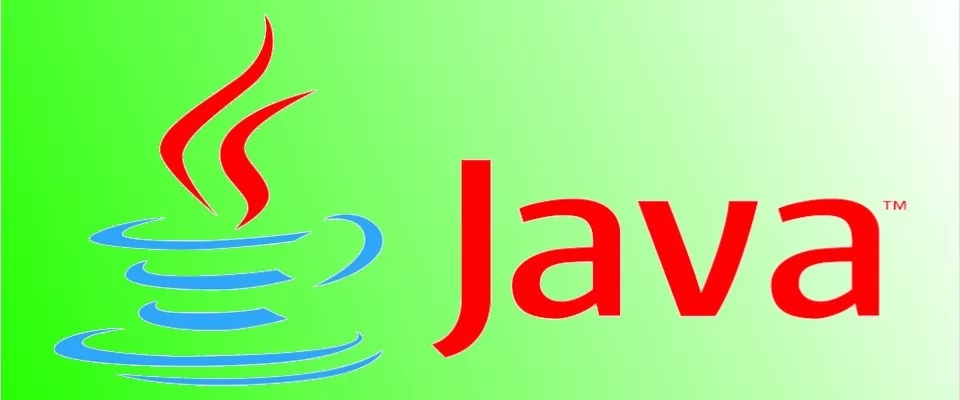
Hello everyone and welcome back! Up to now we have been concerned to make as complete an overview as possible of the fundamental concepts we need to approach the use of conditional structures. We have therefore introduced the concept of conditional structures, outlining the various situations that could arise. We then went on to outline the main features of Boolean algebra, analysing the use of Boolean variables and Boolean operators.
One concept that is worth repeating is that conditional structures, also called flow control structures, serve to modify the normal flow of program execution.
Since learning to program is not enough to read, let's go and analyse some practical examples so that we can see in action the concepts we have analysed so far. It must be said that, in examining some examples, I will take for granted the theoretical and practical aspects concerning the variables, focusing on the aspects concerning the selection structures.
Case 1: Nested selection structures
A first question that could be asked is the case of the nested selection structures. One might wonder whether a second if can be grafted within an if.
The answer is yes.
Let's see how.
// check that n1 is greater than or equal to n2 if (n1 >= n2) { // check that n1 is greater than or equal to n3 if (n1 >= n3) { largest = n1; } else { largest = n3; } } else { // check that n2 is greater than or equal to n3 if (n2 >= n3) { largest = n2; } else { largest = n3; } } System.out.println("Largest Number: " + largest);
This example may seem complex on the surface, but if we read it carefully, we realize that the task it performs is quite simple: it is concerned with finding the variable that has the maximum value within a set of three variables.
To do this, the reasoning is as follows: to be the maximum, one value must be greater than the other two. So what happens is that you check if n1 is greater than n2. If this is true, then you check that n1 is also greater than n3. If two conditions are also true, then you value the variable largest with the value contained in n1.
If n1 is less than n2, we know that n1 cannot be the maximum. The check with n2 and n3 therefore remains to be done. If n2 is greater than n3, then n2 is the maximum of the three variables. Otherwise, the maximum is n3.
We can easily see that it is possible to engage more if one inside the other. This is allowed, though not always encouraged.
Syntax note: the curly brackets
Technically, Java allows us to omit the curly brackets from both the if blocks and the other blocks, as long as they contain only one instruction and no more.
Often this practice is used, and I admit that I do it myself: it has to be said that, in my opinion, we are losing legibility and clarity. Obviously, we are going to gain in compactness of the code. In the case of nested ifs, I recommend using them all the time.
Case 2: Selection structures with composite conditions
Another interesting case to analyse is the one where Boolean operators are used within the conditions. The classic example that can be analysed is one where you want to check that a given number falls within a range. Think, for example, of a software to make statistical evaluations of the school performance of a class.
Suppose we want to count how many grades are sufficient on a scale from 1 to 10, i.e. with a value greater or equal to six.
So let's see how we can do this, assuming we have already declared and initialised a variable called grade.
int counter = 0; if( grade >= 6 && grade <= 10){ counter = counter + 1; }
The idea is therefore that a grade should be considered sufficient if and only if it is greater than or equal to 6 and at the same time less than or equal to 10. On a first attempt, someone might argue that a simple grade check >= 6 is sufficient. The problem arises, however, if there is an insertion error and a grade greater than ten is inserted. Our application would consider it sufficient, even if it is not a valid grade. One would then find oneself considering legitimate a situation that should be handled as a problem.
Case 3: condition with negation
Often, it may be convenient to use conditions that contain a denial within them. This may be for different reasons. It may be developmental convenience or mere readability.
Let's see an example. As a case of use we can always keep the case of grade analysis. Let's assume that we want to check that a grade is valid. A solution might be similar to the one produced before. A far more readable and simple solution, in my opinion, is the following.
boolean voteValid = vote >= 6 && vote <= 10; if(!voteValid){ //Manage error }
So we notice that the code here is very easy to understand. In natural language, the condition can be read as "if not votoValido", which translated into Italian natural language, can be "if the vote is not valid". So we understand that the if will be executed only if the vote is not valid. This is the case when using a Boolean variable greatly simplifies code development.
Conclusions
This brief examination leaves us with some salient aspects. In particular, let us see how operators can be combined to form complex expressions.
It is important, in these situations as in the rest of programming, to pay attention to code indentation. The risk is to write a code that is very complicated to read, if you don't insert the necessary spacing at the beginning of the line.
We will see later on that there is a valid alternative to the massive use of if constructs: the switch statement.
It should be pointed out and reiterated that the analysis made is by no means exhaustive. The idea is to first give the fundamental tools to start writing code, and then to deepen some peculiar aspects.
To practice, it may be useful to solve the following exercise:
Write a SmallEven program that asks the user to enter a whole number and displays the message "Even and Small" if the number is even and is between 0 and 100, otherwise it prints the message "Not even and small". (Note: this program can be done using nested if-else or Boolean expressions. Try both versions).
The exercise comes from the following document.
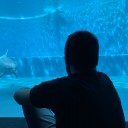
Alessio Mungelli
Computer Science student at UniTo (University of Turin), Network specializtion, blogger and writer. I am a kind of expert in Java desktop developement with interests in AI and web developement. Unix lover (but not Windows hater). I am interested in Linux scripting. I am very inquisitive and I love learning new stuffs.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…