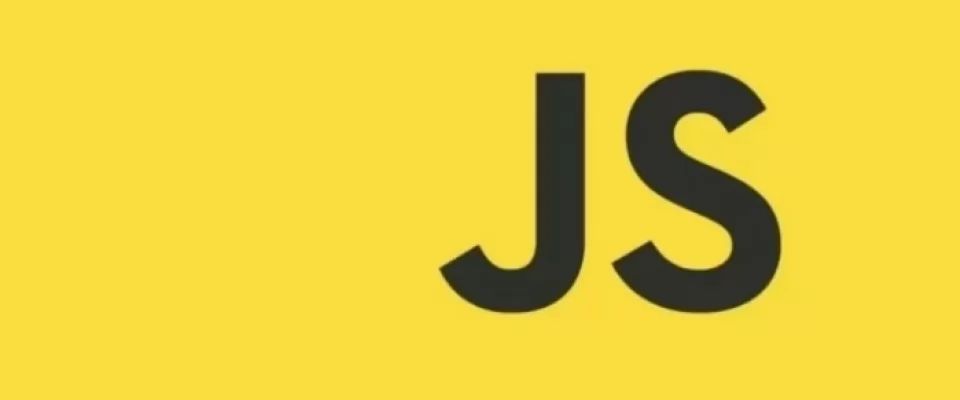
A sleep()
function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for many reasons: from waiting for some condition to be met before continuing with the code, to simulating during development an asynchronous connection that takes a long time to return something.
Almost all languages and platforms have some way of doing this, but JavaScript does not have this functionality natively. In this article we are going to see how to implement a sleep() function in JavaScript using two different methods: the classic and the modern, explaining how to achieve this and what their advantages and disadvantages are.
sleep()
with "classic" JavaScript (ECMASCript 5)
Back in the days of ECMAScript 5 (i.e. before 2015) the only way to simulate a sleep()
function was to run a crazy loop for as long as we were interested in "stopping" the execution of the code. The implementation of a sleep()
function would look something like this:
var sleepES5 = function(ms){ var waitUntil = new Date().getTime() + ms; while(new Date().getTime() < waitUntil) continue; };
As you can see, what it does is to add up the number of milliseconds passed as a parameter and run a loop without doing anything until the stipulated time has elapsed. It couldn't be simpler.
Then you could put it in a code fragment to stop it for a while by writing something like this:
function testES5(){ console.log(>'we start the test.'); sleepES5(3000); //We sleep the execution for 3 seconds console.log(>'End of test function.'); };
This does the job, and can be useful for development testing and the like, but it has several drawbacks:
1. The execution does not really stop, since what is being done is to execute a loop thousands of times while we wait. So it is using CPU and, in fact, if you set a longer or shorter time (a few seconds, depending on the browser), you will end up getting a warning message to stop the execution.
2. The user interface crashes. Since JavaScript has only a single thread, it cannot execute two tasks at the same time (and the code is not in a Web Worker or a Service Worker), so during the wait the UI stops responding. In reality, the clicks and actions you perform are queued and executed when the execution of the "runaway" code finishes.
If in our example we place a button that you can press during the wait to do something else, it will ignore it until the execution is finished.
Note that the execution stops for 3 seconds, but the button presses are only executed when the wait is over. In other words, the wait is not a wait, but a blocking of execution for the indicated time.
Moreover, if we look at the browser's task manager and look at the CPU usage of the page during timeout, we will see that it reaches very high peaks of usage (which depend on the power of your computer).
As I say, it's a "trick" that works, but it's not a real sleep() and presents problems. But well, in "classic" JavaScript, before ES6, it was the solution we had.
sleep()
with modern browsers (ES6)
One of the big and long-awaited new features when ECMASCript 6 came out was the addition of the promise
feature to the language.
A promise is an object representing a task that "promises" to be executed at some point in time (in the future or even in the past, although it may seem counterintuitive), and they are ECMAScript's native mechanism for executing code asynchronously.
As promises have their complexity, the async
and await
keywords were also incorporated into the language to simplify the handling of asynchronous functions with promises.
Let's take advantage of this functionality to create a sleep()
function that actually works as expected.
Doing so is actually quite simple, as we only need to return a promise and have it automatically resolved after the specified time. In other words, the code is the following:
var sleep = function(ms){ return new Promise(resolve => setTimeout(resolve, ms)); };
The promise
is created and a timeout
is specified as a resolution function, which is executed at the end of the specified time. The timeout
function has access to the value of the variable ms thanks to the function closure.
It couldn't be easier!
OK, let's see how this would run in our code now:
async function pruebaES6(){ console.log('we start the test'); await sleep(3000); //We sleep the execution for 3 seconds console.log('End of test.'); };
Note that the function calling our sleep()
function, like any function calling an asynchronous method, must have an async
modifier in front of it. And the call must be preceded by an await
.
During the 3-second wait, we have pressed the send console message button 3 times, as in the previous example.
If you look closely and compare it with the execution of "traditional" code you will see that, apart from executing the wait, now the user interface does not block and when we press the other button the messages arrive at the console at the same time we do it. That is, this sleep()
really stops the execution of our code and also does not block the main thread of the browser and therefore everything is interactive.
And what about processor usage?
Well, during the whole time the execution is stopped, the CPU usage is 0%:
So, perfect! We've got a real, working sleep() with no disadvantages for doing so.
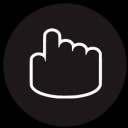
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
What are javascript symbols and how can they help you?
Symbols are a new primitive value introduced by ES6. Their purpose is to provide us unique identifiers. In this article, we tell you how they work, in which way they…