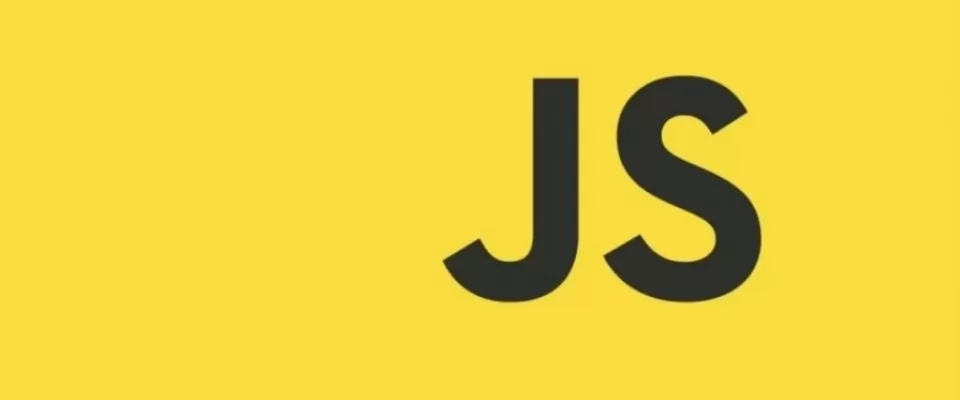
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities.
About template literals
Template literals were introduced in the ES6 version of JavaScript, allowing strings to be declared using inverted-quote syntax:
const chain = `text of the chain`;
This method offers new possibilities compared to strings declared with standard single or double quotes. For example, using this syntax it is possible to deifnir strings on several lines, and you can also insert variables anywhere in the strings without having to concatenate several strings.
In addition, you can also create DSLs (Domain Specific Language) with embedded tags, just like in React or Styled Components. Don't worry if you don't understand what I'm talking about, we'll look at each of these features in detail below.
Inserting Variables
Inserting variables or expressions in the middle of strings is very easy with template literals. This is also called variable interpolation. To do this, simply use the syntax ${varaible} o ${expresión}
In this example we insert a variable into a string:
const web = 'mano'; const mychain = `Welcome to ${web}`;
You can also insert any expression, be it a function or an operation. In this example we insert a mathematical expression:
const operation = `The sum of 2 + 2 is ${2 + 2}`;
In this example we call a function and display one thing or another based on the result of the function:
const result = `The result is ${check() ? 'correct' : 'false'}`
Strings on multiple lines
With the old JavaScript strings, as they were before ES6, it is also possible to define multi-line strings at the editor level by using
at the end of the line:
const chain = 'This is a multi-line string';
This allows you to define strings on multiple lines, but when rendered, you will only see them on one line. In the case of the example above, you will see the string This is a multi-line string
on the screen, on a single line.
However, it is also possible to define them on two real lines using the line break character:
const chain = 'This is a n multi-line string';
In case you want what you type on the screen to be transferred to your output, you will have no choice but to combine the line break n
with the character :
const chain = 'This is a n multi-line string';
However, template literals make it much easier to define multi-line strings, because once you start the string, just press ENTER to create a new line, which will be reflected both in your editor and in the final output:
const chain = 'This is a multi-line string';
You can define as many lines as you want:
const chain = `This is the first line of the chain, this is the second and this is the third.`;
However, you must be careful with the spaces and indentations in your editor, because if you define the following string:
const chain = `This is the first line of the chain, this is the second`;
What you will see on the screen is the following:
This is the first line of the chain, this is the second
What you can do to fix this is to leave the first line blank so you don't have to add spaces in the second line if you want to format the code:
const chain = ` This is the first line of the chain, this is the second`.trim();
As you can see we have also used the trim()
function to remove the first line break.
Template tags
This is a feature that at first glance seems unimportant, but in reality it is used by lots of famous libraries such as GraphQL, Apollo or Styled Components. Let's see how it works. Actually I've always worked with JavaScript in another language, so I have no idea what to call them in English, so I'll say template tags, although it doesn't sound too good, to be honest.
Template tags are simply functions that are placed before the template literal, like this:
templatetag`literaltemplate ${2 + 2}`;
As you can see, we have added the templatetag function, which should correspond to a real function that we have defined:
function templatetag(literals, ...expressions)
{
// Function code
}
This function accepts two parameters; literals and expressions. Let's see what each of these parameters represents:
Literals: An array containing the different parts of the template literals; that is, the parts of the text string that separate the interpolated elements.
Expressions: Contains all the expressions, variables or elements that we have interpolated.
Let's propose the following example.
const chain = `the result of ${2 + 2} it's four`;
The literals in our example will be an array with two elements. The first element of the array will be the string the result of and the second element will be the string is four, including spaces. That is, the text strings that are delimited by the interpolated elements are included.
On the other hand, the expressions in our example will contain only one element, which will be the element 4, the result of 2 + 2.
As you can see, just like when using normal template literals, we do not specify a function. However, that doesn't mean that no function is executed, as by default a function will be assigned that interpolates variables and strings.
Let's now define a more complex example like the following, where the blue variable has the value '#00bed5' and the green variable has the value '#11b200':
const chain = `I will say that heaven is ${blue} and the grass ${green} or so I think`;
In this example the literals will be an array whose first element will be:
`I will say that heaven is `
The second element, which includes the line break, will be:
` and the grass `
And finally the third element, also including the line break, will be:
` or so I think`
As for the expressions, in this case they are an array with the elements '#00bed5' and '#11b200'.
The function passed to that template literal can do any task with those parameters.
In the following example we are going to define a function or template tag that, as it usually happens when you don't assign any function, interleaves the expressions with the literals:
const result = interpolate`I have ${50}€`
Below is the interpolation function we could use, as an example:
function interpolate(literals, ...expressions) { let chain = ``; for (const [i, exp] of expressions.entries()) { chain += literals[i] + exp; } chain += literals[literals.length - 1]; return chain; }
As mentioned before, both GraphQL and Styled Components or Apollo use template tags.
In Styled Components, template tags are used to create CSS definitions of components. In the following example, the template tag would be the styled.h1
function:
const Title = styled.h1` font-size: 1.6em; text-align: center; color: #000; `;
In Apollo, template tags are used to define GraphQL query schemas. In the following example, the template tag would be the gql
function:
const consult = gql` query { ... } `
And that's it
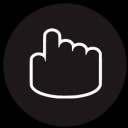
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
What are javascript symbols and how can they help you?
Symbols are a new primitive value introduced by ES6. Their purpose is to provide us unique identifiers. In this article, we tell you how they work, in which way they…