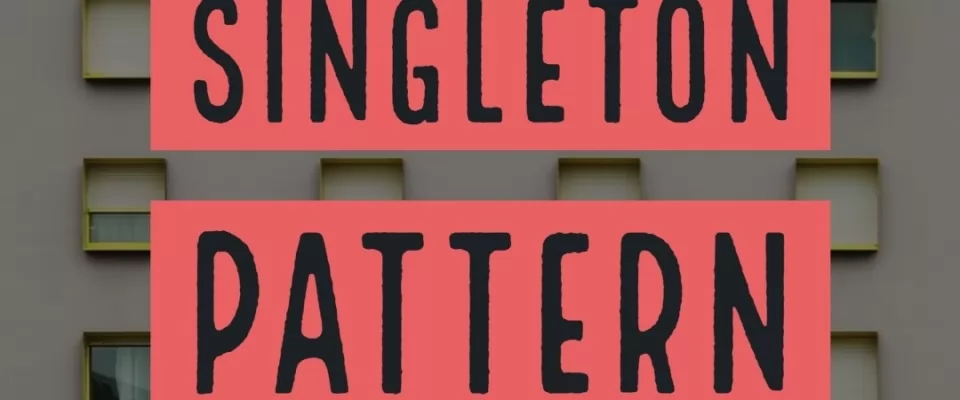
Anyone with even a minimum experience of programming, should have realized that the majority of the problems have common elements. In fact we often find problems with the same pattern but in different contexts.
For example, a management software for s supermarket and the one for a great industry will operate on different data but the functions implemented could be similar. By extending this type of concept to all programming, it can be said that the problems encountered in developing large software projects are often recurrent and predictable.
This type of reasoning gives rise to patterns, better known as design patterns.
What does it mean to use design patterns?
Using design patterns means not to invent from the beginning new solutions but using working and consolidated solutions developed over the years. The idea is of providing a common "dictionary" to the majority of the developers.
Trying to give a theorical definition, a pattern is a collection of classes and objects that communicate with each other adaptable to solve a recurrent problem in a specific context.
The important thing is that they were designed as non-domain-specific, so they are not intended for specific applications, but are reusable in parts of different applications.
This type of design is commonly used also in java classes like Iterator.
Nowadays, patterns can be sorted as shown below:
- creational patterns: they deal with the objects creation process;
- structural patterns: they deal with the classes and objects' composition;
- behavioral patterns: they deal with the interaction and the responsibility distribution among classes;
A very simple example: Singleton
The need of this kind of design pattern is originated from the fact that we could need a class with one and only one instance.
The implementation, possibly, has to be thread-safe. Let's look at an example:
Classical implementation
Here is the UML diagram:
public class Singleton{ /* This will be the only instance of the class Every access will be through this instance and no instance will be created anymore */ private static Singleton instance = null; /* private constructor to avoid other instances to be created */ private Singleton(){ } /* Unique access point */ public static Singleton factory(){ //create the object if it doesn't exist if(instance==null) instance = new Singleton(); return instance; } //other methods }
This kind of implementation is not suitable for a multi-threading context. A possible modification could be:
public class Singleton{ /* This will be the only instance of the class Every access will be through this instance and no instance will be created anymore */ private static Singleton instance = null; /* private constructor to avoid other instances to be created */ private Singleton(){ } private synchronized static Singleton createInstance(){ if(instance==null) instance=new Singleton(); return instance; } public static Singleton factory(){ if(instance==null) //invoke the synchronized method only if //the instance doesn't exist createInstance(); return instance; } //other methods. }
Understanding the code
The two examples carry out similar functions even in in slightly different ways.
The first example has a static attribute that represents the only accessible instance of the class. The constructor has been made private in order to avoid the creation of other instances of the class.
The access to the class can happen only through the method factory that checks the static attribute state. If it doesn't exist, it is instantiated. The method ends returning to the caller the attribute.
There will be two possible cases:
- instance == null: the method will instantiate "instance" and return it to the caller;
- instance != null: the method return to the caller the pointer to instance;
At first sight, the implementation is relatively easy for anyone who has a little bit of programming experience. In a multithread situation some synchronization problems may occurr, so a thread-safe version has been realized.
The logic of the second version is exactly the same as the first, like the final result, but with a slightly different application logic.
The first thing that we can notice is that in the thread-safe version there are two methods despite of the first where there were only one. The first method is necessarily synchronized in order to guarantee a correct synchronization amont the threads.
Remeber that a synchronized method is a method such that when a thread call the synchronized method on an object, every other thread that call that method later suspend its execution until the execution of the method that has been called first, ends.
The method createInstance() is responsible for checking the attribute status and based on its value, instantiate it or not.
Factory is a wrapper for the createInstance() methdo, checking also the instance attribute state.
This double check, that could seem to be without any sense, is useful in order to decrease the overhead. Strategies like this are called double-checked locking.
There are other strategies to implement a singleton that are more complex that decrease the overhead too.
A possible alternative could be:
public class Singleton{ private static Singleton instance = null; private Singleton(){ } public static Singleton getIstance() { if(istance==null) synchronized(Singleton.class) { if( instance == null ) istance = new Singleton(); } return istance; } //eventuali altri metodi }
Must be said that nowadays several criticisms are made towards this model, because the Singleton Pattern problem is misunderstood and often used to introduce the concept of global variables in its own system by introducing the application in the global state in the application domain. This is bad, because global variables are notoriously not concerned with the structure of the program.
Another strong criticism that is made concern with the fact that the majority of the Singleton classes don't respect the general principles of the software engineering, working as an aggregator for different functions without any relation between each other, introducing the dependence concept, violating the concept of single responsibility.
In fact, standing to the software engineering principles, every element of the software must have only one responsibility that must be encapsulated by the element itself.
The Singleton allows the access by a static method and this charateristic makes it possible to use the instance within other methods without going through the parameters. Despite it could seem convenient, it means that the methods' signature don't show the dependencies anymore. This means that the programmer has to know the internal logic of the code. In this way, the code is more difficult to test and debug.
A third defect is the violation of the Liskov's Substitution Principle. In fact, since it does not present inheritance relationships, it is impossible to replace the objects linked by a family relationship.
Despite of all the criticisms, if not abused, the Singleton can represent a resource, although we will see more strategies to solve similar problems later.
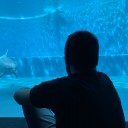
Alessio Mungelli
Computer Science student at UniTo (University of Turin), Network specializtion, blogger and writer. I am a kind of expert in Java desktop developement with interests in AI and web developement. Unix lover (but not Windows hater). I am interested in Linux scripting. I am very inquisitive and I love learning new stuffs.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…