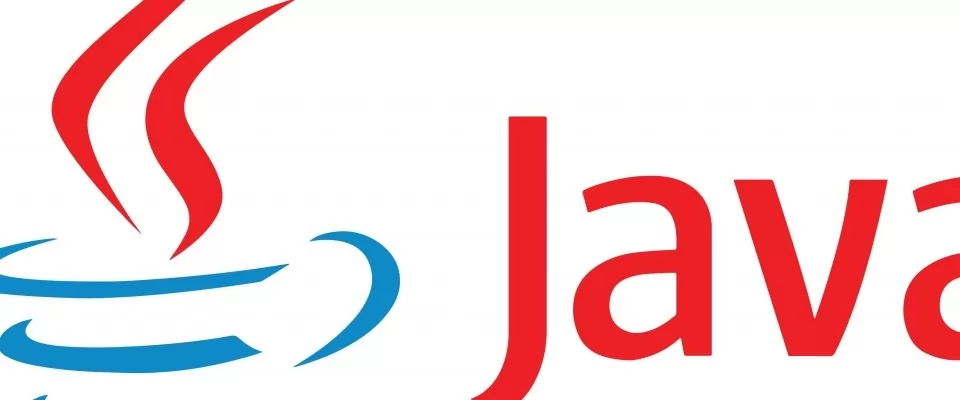
Hello everyone and welcome back!
Today we will begin a journey that will lead us to study, and possibly review, what are the basics of programming. We will start by talking about variaibli.
Introduction
Anyone who wants to approach the world of programming will soon realise that coding is not exactly like what is shown in films. It is not just about printing messages on screen, or rather, printing messages on screen is only the final part of a much more complex process that requires real craftsmanship.
In this series of articles, we will try to understand what the basic bricks of this activity are and above all we will try to understand why it is an artisan activity, even though it seems to be anything but.
Variables - Definition
The first fundamental element that anyone needs to know in order to approach the world of coding is the concept of variable.
This is a concept as simple as it is fundamental. Wanting to give a first definition, however crude, one could say that a variable is an entity that changes over time. Putting it that way might seem a rather random definition. Let's try to understand it better.
Let's start with an example
Let's imagine that we have to develop a simple application to calculate the area of a square plot of land. The user will be asked to enter the side of his terrain and the program will return the area on screen.
To print on screen, there are several instructions provided by the various languages, Java in our case. The bulk of the work consists of the actual resolution of the problem.
The first practical problem that arises is: is there a way to save the data that the user provides us with? The answer is yes. It is possible to achieve this through the use of variables.
It is therefore necessary to know that every data that our program uses is saved in what in the literature is called primary memory, commonly known as RAM memory. Therefore, each variable is saved in RAM. It is the programmer's responsibility not to fill up memory with useless data unnecessarily. At the same time, we must always ask ourselves on the one hand if the variable is necessary, but on the other hand if its removal brings advantages.
At this point, it is possible to give a more refined and more relevant definition of the variable context. A variable is therefore a memory location within which data is read and written.
Declaration and initialization of a variable
At this point, after a long introduction, we can move on to practice. We must, first of all, understand what are the fundamental operations that are carried out on a variable.
Declaration
The first thing we have to do is "communicate" in some way to the computer that from a certain moment on, we intend to use a certain variable.
This is possible through an operation that is called declaration.
The declaration of a variable is mainly composed of two parts. We could define a prototype like the following: dataType variableName.
Data type
Let's take a closer look at what a given type is.
Type is a classification term that groups together all those variables that are stored in the same way and to which the same set of operations applies.
In mathematics, when we define a numerical set, we define a set of operations that can be performed on that set. Thinking of the set of natural numbers, we know of operations that we can perform such as sum or division. We also know that the same operations will be applicable to each number of that set.
In the same way, we classify the variables in a series of sets. Let's summarize in a table what these sets are.
Tipo |
Memory quantity |
Information represented | |
byte |
8 bit |
Variable with sign and represents values in a range [-128 and 127] (extremes included) | |
short |
16 bit |
Integer numbers (with sign) in a range [-32768, 32767]. | |
int |
32 bit |
Integer numbers (by default with sign, signed) in a range [-231, 231-1]. | |
long |
64 bit |
Integer numbers (by default with sign, signed) in a range [-263, 263-1]. | |
float |
32 bit |
Single precision floating point numbers according to the IEEE 754 specification, using the sign, mantissa exponent representation. | |
double |
64 bit |
Double precision floating point numbers according to IEEE 754 specification. | |
boolean |
Not specified. just one bit would be enough | represents two values: true and false | |
char |
16 bit |
Used to store Unicode encoded characters in the range ['u0000', 'uffff'](in hexadecimal) or equivalent [0.65535]. |
Variable name
When we declare a variable we will also have to name it. This necessity arises from the fact that we cannot know a priori in which area of memory the data we are interested in will be stored. We therefore need to have an alias to refer to a specific memory location.
We can therefore give a third and final definition of variable. A variable is a reference to a memory location in which a data of interest is stored.
Initialisation
Initialisation of variables is the preliminary phase of programming in which they assign initial values to previously declared variables. Initialisation follows the declaration of the variables or, alternatively, it can be done jointly.
Let's see an example.
int var; var = 5;
In this way, after declaring the variable var, we assign the value 5 to the point cell.
Alternatively, the operation can be performed together with the declaration as follows:
int var = 5;
The initialization uses an operator who is the assignment operator, characterized by the symbol =.
Assignment operator
The assignment operator is the operator with whom the variables are valued. The fundamental thing to remember is that a memory location can contain one value at a time. It is categorically impossible for a location to contain two values at the same time. From this we can deduce that an assignment completely deletes the previous value of that cell.
It must be said at this point that I will be making relatively improper use of language from now on. When I say "assign a value to a variable" I mean "write a value in the memory cell pointed by the variable".
Let's see an example.
int a = 0; //The variable contains 0 a = 3; //The variable a now contains the value 3 a = 5; //The variable a now contains the value 5
Conclusions
Here we have given the basic definitions, introducing the indispensable operations to know in order to use the variables. We will soon see, starting from a practical example, how this concept can be used to develop a working application.
As boring and useless as this introduction may seem, we will see later on how these concepts are really fundamental, not only to learn how to program in Java, but to program in the vast majority of languages.
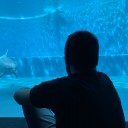
Alessio Mungelli
Computer Science student at UniTo (University of Turin), Network specializtion, blogger and writer. I am a kind of expert in Java desktop developement with interests in AI and web developement. Unix lover (but not Windows hater). I am interested in Linux scripting. I am very inquisitive and I love learning new stuffs.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
