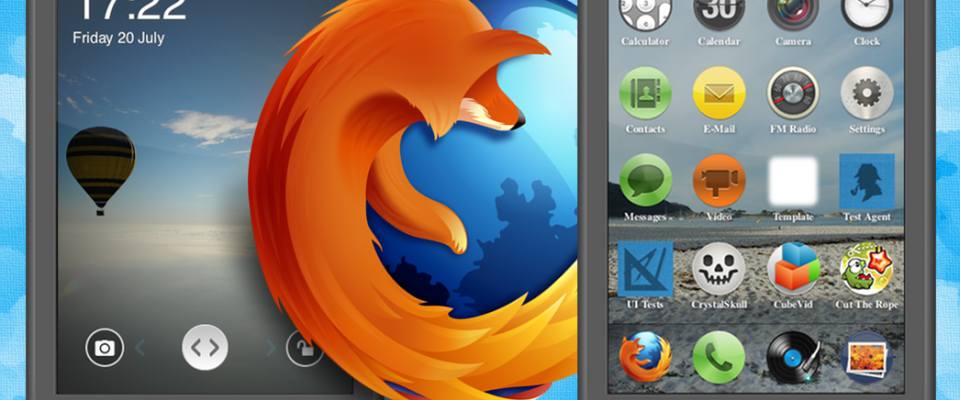
Nearly two years ago, Mozilla announced "Boot to Gecko" (a.k.a. B2G), a project whose primary goal was to provide a standalone web-centric operating system in which the preferred (and in fact, only) platform for application development is the HTML5 stack. In essence, this operating system would have filled a gap that prevented web developers from writing true native apps.
This announcement was met with a real sense of excitement from the mobile developer community. Firefox OS, as it would soon be appropriately named, captivated and excited web developers because of Mozilla's embrace of the HTML5 stack. No more picking and choosing between Objective C and Java—HTML5 would be a first class citizen. In theory, with minimal tweaks to existing HTML5 web apps, developers could port these same apps to Firefox OS in no time (much like PhoneGap developers have been doing for other platforms).
In spite of the excitement (and inherent fear) of developing for a new OS, Mozilla is facing an uphill battle to carve out even a niche market from the current dominant mobile platforms (such as iOS and Android). Combined with the heavyweight presence of Microsoft (Windows Phone 8) and Blackberry (RIM), Mozilla clearly has their work cut out for them. Just as importantly, Mozilla has to convince existing web and mobile developers as to why they should develop for Firefox OS given these obstacles.
Figure 1. An example Firefox OS home screen
For the moment, let's ignore the "why" we should develop for Firefox OS and instead focus on the "how".
WebAPIs and Web Activities
The following is a simplified example of Robert Nyman's Introducing Web Activities post. Some of the more intriguing aspects of Firefox OS include WebAPIs and Web Activities. A WebAPI is essentially a JavaScript interface that lets your app access native device functions (managing contacts, sending SMS messages, push notifications, and so forth). A Web Activity is a WebAPI. Specifically, a Web Activity allows your app to delegate an activity to another app on the device. For example, if you want to allow your user to grab an image from his device, you can call the "pick" Web Activity and specify an array of data types as follows:
var pickImg = new MozActivity({ name: "pick", data: { type: ["image/jpg", "image/jpeg", "img/png"] } });
This code produces the following dialog for the user.
Figure 2. Using a Web Activity to produce a dialog for the user.
Most WebAPIs and Web Activities have
onsuccess
and
onfail
event handlers, which you can use to handle the response of the API call. For example, in the following code:
pickImg.onsuccess = function () { // we got an image! (returned as a blob) var blob = this.result.blob; var img = document.createElement("img"); img.src = window.URL.createObjectURL(blob); // now display the image in your app $("#image-display").append(img); }; pickImg.onerror = function () { // didn't work... alert("Something went wrong!") };
I use a simple WebAPI example in the sample app below.
Developing your first Firefox OS app
There are two types of apps (both which Mozilla calls Open Web Apps) that you can install on Firefox OS: a hosted app and a packaged app.
A hosted app is essentially a web site hosted on a central server, but executed within the app context. Users need to be online to access the app resources (HTML, images, and so forth) and developers are limited in the scope of WebAPI calls the app can make. You can see the difference between regular and privileged WebAPI calls; see more in the article, Using WebAPIs to make the web layer more capable. However, like a regular web site, developers have more control over app updates since the app resources are still centrally hosted. This article does not focus on hosted apps, but I encourage you to read more about them by following Robert Nyman at Mozilla.
A packaged app is similar to a hosted app (it's still HTML5) but it has access to all of the WebAPIs and is downloaded and installed on the device itself (similar to iOS and Android). By default, packaged apps are expected to function without requiring network access. Even though the app itself lives on your device, it is possible for a packaged app to update itself. You may also use the Firefox Marketplace to push packaged app updates to your users.
Enough talk. Lets build a simple Firefox OS (packaged) app from scratch that lets a user find out how much juice is left in their battery.
Creating your sample packaged app
Copy the following code and save it as index.html.
<!DOCTYPE html> <html> <head> <title>Check My Battery Level</title> <meta name="viewport" content="width=device-width" /> <script src="js/jquery-1.9.1.min.js"></script> <script src="js/app.js"></script> </head> <body> <button id="get-battery">Get Battery Level</button> <p>How much juice is left? <span id="battery-pct"></span></p> </body> </html>
Next, paste the following JavaScript code into a new file called app.js inside a folder called js:
$(function() { $("#get-battery").click(function() { $("#battery-pct").text(Math.round(navigator.battery.level * 100) + "%"); }); });
Ensure that you have downloaded jQuery and placed the file within your js folder (update the file name in the HTML above if it has changed).
What have we learned? Not much yet. We have an HTML5 app that makes a simple WebAPI call, but now we need to make some relatively minor additions to make it into a packaged app.
During the development process of your app, use of the Firefox OS Simulator. This is a free Mozilla add-on (for the Firefox browser of course) that allows you to simulate the Firefox OS environment for both hosted and packaged apps.
Creating the manifest file
All Firefox OS apps (hosted and packaged) need a manifest file. A manifest file is simply a JSON file that contains metadata about your app. Below you'll find a simple version of an app manifest.
{ "name": "Check Battery", "description": "This app allows you to check your battery levels!", "launch_path": "/index.html", "developer": { "name": "Rob Lauer", "url": "http://roblauer.me" }, "icons": { "16": "/img/icon16.png", "32": "/img/icon32.png", "48": "/img/icon48.png", "64": "/img/icon64.png", "128": "/img/icon128.png" }, "default_locale": "en" }
Save this file as manifest.webapp in the same directory as your index.html file. (And yes, you have to use that exact name.)
Note: If you are utilizing Web APIs that are only available to packaged apps, you will need to specify some additional permissions in your manifest file.
Testing the app in the simulator
If you haven't already done so, install the Firefox OS Simulator. Once it is installed you will see the following simulator dashboard.
Figure 3. Simulator dashboard
If you don't see this screen, go to the Web Developer Firefox menu and select Firefox OS Simulator.
Since we are working with a local app, click the Add Directory button and select the directory containing the manifest.webapp file you just created. The simulator automatically starts and displays the lock screen (Figure 4).
Note: If nothing happens here, don't panic. You may have a problem with your manifest file or your manifest may be in the wrong directory. Make sure you copy and paste exactly what I have provided above, and that your manifest.webapp file is located in the same directory as your index.html file.
Figure 4. The Lock screen
Click and drag the little arrow and then click the lock icon to unlock your device. There are numerous pre-installed apps available, so click and drag a couple screens until you find your app. If you followed my naming conventions, your app will have a default icon named Check Battery. Click the app and see your first app in action!
Figure 5. Selecting your app from the screen
Archiving and validating your app
Next, create a ZIP archive of your entire application (including the manifest file you just created). Archive the files, but do not archive the containing folder. Be sure you've named your manifest file manifest.webapp.
In addition, Mozilla provides a website that allows you to validate your app called Validate an app. I recommend taking this step to avoid any potential issues, whether it be a syntax error in your manifest file or a security violation due to inappropriate use of a WebAPI.
Creating the mini manifest file
To make this into a packaged app, you must create another manifest file. It's a small one. Ensure that your developer information in this manifest file matches your first one. This manifest file is primarily meant to guide the distribution of your app—whether you are using the Firefox Marketplace or allowing installation from your own server. I cover more details on distribution in the next section.
{ "name": "Check Battery", "package_path" : "http://somewhere.com/awesome.zip", "version": "1", "developer": { "name": "Rob Lauer", "url": "http://roblauer.me" } }
Save this file as mypackage.webapp, or using a similar naming convention.
Distributing your app
One of the great things about Firefox OS is that you can distribute apps from your own server, though for more visibility you will also want to use the Firefox Marketplace. If you want to allow a user to install an app from your site, take two simple steps.
- Use feature detection to ensure that the client is a Firefox OS device, as follows:
var canInstall = !!(navigator.mozApps && navigator.mozApps. installPackage);
- Prompt the user to install the app, as follows:
if (canInstall) { var manifestURL = location.href.substring(0, location.href.lastIndexOf("/")) + "/mypackage.webapp"; var installApp = navigator.mozApps.installPackage(manifestURL); }
Apps delivered from the Firefox Marketplace do not require this code. (They are pushed from the marketplace using the package_path property specified in your mini manifest file).
Keep in mind that, at the time of writing, the Firefox OS Simulator only supports app installations through the dashboard interface, meaning you cannot install an app inside of the simulator itself.
Privileged versus certified versus plain packaged apps
Packaged app developers should be aware that there are actually three different types of packaged apps.
- A privileged app is one that undergoes an approval process at the Firefox Marketplace (such as the iOS and Android app stores). This is primarily meant for apps that use any sensitive WebAPI (such as managing contacts).
- A certified app is only for critical Firefox OS device functions (such as dialing the phone), therefore, the vast majority of developers can ignore this type of packaged app.
- A plain app does not undergo the same review process, but is limited in the scope of WebAPI calls it can make.
We have gone over how to create an app for Firefox OS, and now let's get back to why one would want to develop an app for Firefox OS.
Why consider developing for Firefox OS?
Given all of the inherent roadblocks with developing for a new mobile OS, why should we, as web/mobile developers, even consider Firefox OS? In my opinion the answers are:
- Because it's Mozilla
- You've got nothing to lose
It is still Mozilla
Many of us have grown up with Mozilla and, even though the Firefox browser may be showing its age, Mozilla itself remains a massive player in the open web. This alone lends a certain amount of weight to support assertions by Strategy Analytics who forecast a global market penetration for Firefox OS of ~1% in 2013 alone. Consider also the possibility that Firefox OS takes over the role of the increasingly prevalent low-cost Android devices sold at electronics stores around the world. Most mobile developers would agree that getting un-upgradable Android 2.x devices off of the market would do nothing but benefit the community as a whole. My point? Mozilla is no fly-by-night company and has a legitimate shot at making this work.
You've got nothing (well, not much) to lose
As we have already seen, you can take an existing HTML5 application and with a smattering of code turn that into a Firefox OS app. Am I oversimplifying it? Yes, of course, but you can't come close to saying the same thing for any other mobile platform (save for utilizing PhoneGap). If we see market penetration of at least 1% in the next year, it may become a no-brainer when picking and choosing mobile app platform development options.
Where to go from here
Firefox OS is new. Brand new. It is so new that as of this writing, test devices are only just now being delivered to developers. It is important however to consider the potentially broad reach of this platform. If you are interested in learning more, I recommend keeping up with David Walsh and Robert Lyman, who has released an incredibly useful Firefox OS Boilerplate App.
We will all watch and see how this plays out, but in this developer's opinion, it is well worth being aware of the potential and to properly position you to deliver a top notch Firefox OS app.
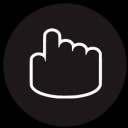
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Android Hidden Codes: unveiling custom dialer codes and their functionality
In the world of Android smartphones, there exist numerous hidden codes that can unlock a treasure trove of functionalities and features. These codes, known as custom dialer codes, provide access…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
Loading images by resolution with HTML5
Normally the way to load images in HTML is through the img element to which we pass as a parameter the URL of the image to load. But since HTML5…
Secret iPhone codes to unlock hidden features
We love that our devices have hidden features. It's fun to learn something new about the technology we use every day, to discover those little features that aren't advertised by the…
How to set up your Android phone: learn how to get your new phone up and running
If you've just bought a great new Android phone, you'll want to start using it as soon as possible. You'll see that it's not hard to get the initial set-up and…
What is DNS Blockchain and how to use it
To be able to navigate the Internet, to enter a website and have it show us all the content we want and search for, certain functions and characteristics are necessary.…
How to Unlock Secret Games in Chrome, Edge and Firefox
Your web browser is full of secrets. I usually spend a lot of time studying new features that I can unlock through pages like chrome://flags and about:config in the browser,…
How to setup an Android TV with androidtv.com/setup or the Google application
Android TVs and players are a good investment if you want to have all the entertainment at your fingertips. However, they require an installation that, although simple, has several ways…
How to move Chrome, Firefox or Edge cache to save space
Caching is a technique used by many programs to improve their performance, especially for applications that run over the Internet, such as streaming programs or web browsers. This technique consists…
How to turn your smartphone into a webcam for your pc
With the propagation of Covid-19 we all had to adapt to a new quarantine situation at home, and since the human being needs to communicate constantly, we used the internet…
5 Fool-Proof Ideas For Location-Based Apps
Like it or not, geolocation is a part of our everyday lives. In fact, you’ll find the technology pre-installed on 90% of the apps currently living on your smartphone. Location-based…
Linux for Dummies: Ubuntu Terminal
I introduced in the previous article, available here, the basic concepts concerning the Linux world. Today we are going to have a look to some basic operations that we can perform…