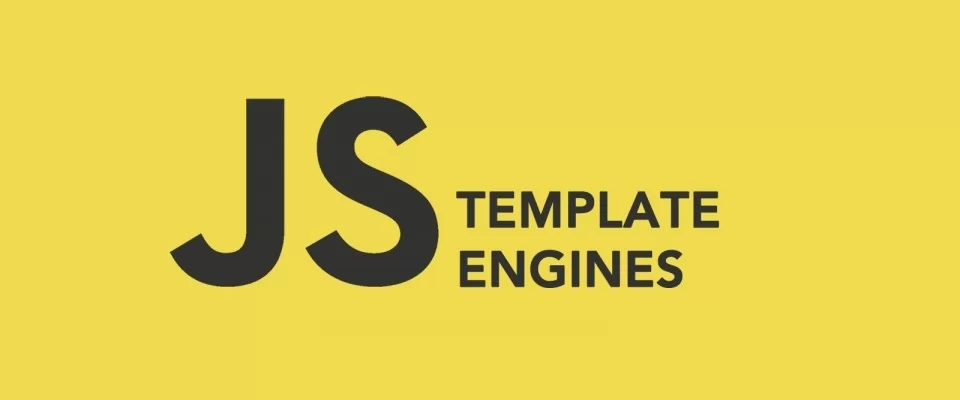
Today we want to publish a resource that can generate an instant boost in your workflow, here we have a list of the Best JavaScript template engines to choose from, and each of them could make your development faster and easier.
When you build a JavaScript application, you'll almost certainly use some JavaScript templates. Rather than use a library like jQuery (or vanilla JavaScript) to update your HTML when values update, you can use templates, which cleans up your code hugely. In this article, we'll look at some popular templating libraries.
You’d probably be pumped to read about them. Lucky for you, that’s exactly what we are going to share with you in this post. If you wish to capitalize on the sentiments of the market by using the best JavaScript template engines platform, in that case, youare in the right place.
Mustache
is often considered the base for JavaScript templating. Another popular solution, Handlebars, actually builds on top of Mustache, but that doesn't mean that it isn't a very good templating solution.
Mustache.render("Hello, {{name}}", { name: "Jack" }); // returns: Hello, Jack
Once Mustache is included on your page, you have access to the global 'Mustache'
object. The main method you'll use is 'render'
, which takes two arguments. The first is the actual template, and the second is any arguments that need to be passed to it.
In the above example, you can see that is referenced '{{name}}'
. Two braces around the variable is the Mustache syntax to show that it's a placeholder. When Mustache compiles it, it will look for the 'name'
property in the object we pass in, and replace '{{name}}' with the value, which is "Jack", in this case.
Here we have passed in the template as a string, but if you had a more complex template, you might not like to do it this way. Instead, a common solution is to place a template inside 'script' tags:
<script type="text/x-mustache" id="template"> <p>Hello, {{name}}</p> </script>
We can then access the contents of that script tag. For example, with jQuery it's as easy as:
var temp = $("#template").html(); Mustache.render(temp { name: "Jack" }); // returns: <p>Hello, Jack</p>
By giving the 'script'
tag a 'type'
attribute of something the browser doesn't understand, it will ignore the contents, so it doesn't try to execute it as JavaScript.
You can also use loops in your templates. Taking this template:
{{#people}} {{name}} {{/people}}
With this data passed in:
{ people: [ { name: "Jack" }, { name: "Fred" } ] }
You'll get the string "JackFred" returned.
Mustache is capable of a lot more than covered here, so do check the Github for more.
Underscore Templates
Underscore is a utlity belt library for JavaScript, providing all sorts of useful methods. It also provides simple templates we can use. It uses a slightly differet syntax to Mustache. Here's a simple example:
_.template("Hello, <%= name %>", { name: "Jack" }); // returns: Hello, Jack
If you've ever used Embedded Ruby (or ERB for short), you may be more familiar with this syntax. The '<%= name %>'
denotes that whatever the value of `name`
should be outputted in place of '<%= name %>'
. Underscore can also do things like loops and conditionals, but it does it slightly differently to how Mustache does.
var template = "<% _.each(people, function(name) { %> <li><%= name %></li> <% }); %>" _.template(template, { people: ["Jack", "Fred"] } );
In Underscore templates, you can embed arbitary JavaScript within '<% %>'
tags. Note that we use '<%= %>'
to output to the page, and `<% %>`
to contain JavaScript. This means any form of loop or conditional you can do in JS, you can use in Underscore.
Art-template
Art-template is a simple and superfast templating engine that use simple templating syntax, simultaneously supports two syntax of template. Standard syntax allows templates to be easier to read and write. While original syntax has powerful logical processing ability, compatible with EJS template. Also it optimizes template rendering speed by scope pre-declared technique.
standard syntax:
{{if user}} <h2>{{user.name}}</h2> {{/if}}
original syntax:
<% if (user) { %> <h2><%= user.name %></h2> <% } %>
It achieves runtime performance which is close to the limits of JavaScript. At the same time, it supports both NodeJS and browser.
DOT
Created in search of the fastest and concise JavaScript templating function with emphasis on performance under V8 and Nodejs.
Basic usage:
<html> <head> <script id="headertmpl" type="text/x-dot-template"> <h1>{{=it.title}}</h1> </script> <script id="pagetmpl" type="text/x-dot-template"> <h2>Here is the page using a header template</h2> {{#def.header}} {{=it.name}} </script> <script id="customizableheadertmpl" type="text/x-dot-template"> {{#def.header}} {{#def.mycustominjectionintoheader || ''}} </script> <script id="pagetmplwithcustomizableheader" type="text/x-dot-template"> <h2>Here is the page with customized header template</h2> {{##def.mycustominjectionintoheader: <div>{{=it.title}} is not {{=it.name}}</div> #}} {{#def.customheader}} {{=it.name}} </script> <script src="../doT.min.js" type="text/javascript"></script> </head> <body> <div id="content"></div> <div id="contentcustom"></div> </body> <script type="text/javascript"> var def = { header: document.getElementById('headertmpl').text, customheader: document.getElementById('customizableheadertmpl').text }; var data = { title: "My title", name: "My name" }; var pagefn = doT.template(document.getElementById('pagetmpl').text, undefined, def); document.getElementById('content').innerHTML = pagefn(data); pagefn = doT.template(document.getElementById('pagetmplwithcustomizableheader').text, undefined, def); document.getElementById('contentcustom').innerHTML = pagefn(data); </script> </html>
It shows great performance for both Nodejs and browsers.
doT.js is fast, small and has no dependencies.
JavaScript-Templates
JavaScript-Templates It's a 1KB lightweight, fast & powerful JavaScript templating engine with zero dependencies. Compatible with server-side environments like Node.js, module loaders like RequireJS, Browserify or webpack and all web browsers.
Example: Add a script section with type "text/x-tmpl"
, a unique id property and your template definition as content:
<script type="text/x-tmpl" id="tmpl-demo"> <h3>{%=o.title%}</h3> <p> Released under the <a href="{%=o.license.url%}">{%=o.license.name%}</a>. </p> <h4>Features</h4> <ul> {% for (var x=0; x < o.features.length; x++) { %} <li>{%=o.features[x]%}</li> {% } %} </ul> </script>
In your application code, create a JavaScript object to use as data for the template:
var data = { title: 'JavaScript Templates', license: { name: 'MIT license', url: 'https://opensource.org/licenses/MIT' }, features: ['lightweight & fast', 'powerful', 'zero dependencies'] }
JavaScript-Templates it's compatible with server-side environments like node.js, module loaders like RequireJS and all web browsers.
View on Github
EJS
EJS is a simple templating language that lets you generate HTML markup with plain JavaScript,is inspired by ERB templates and acts much the same. No religiousness about how to organize things. No reinvention of iteration and control-flow. It's just plain JavaScript, it uses the same tags as ERB (and indeed, Underscore) and has many of the same features. It also implements some Ruby on Rails inspired helper.
EJS is different in that it expects your templates to be in individual files, and you then pass the filename into EJS. It loads the file in, and then gives you back HTML.
// in template.ejs Hello, <%= name %> // in JS file new EJS({ url: "template.ejs" }).render({ name: "Jack" }); // returns: Hello, Jack
Note that you can load in text templates, too:
new EJS({ text: "Hello, <%= name %>" }).render({ name: "Jack" });
Here's how we would loop over some people, and link to their profile pages on our website:
// template.ejs <ul> <% for(var z = 0; z < people.length; z++) { %> <li><%= link_to(people[z], "/profiles/" + people[z]) %></li> <% } %> </ul> // in JS file new EJS({ url: "template.ejs" }).render({ people: [ "Jack", "Fred" ] }) // Each rendered <li> will look like: <li><a href="/profiles/Jack">Jack</a></li>
That's very similar to how Underscore might do it, but note the use of `link_to`. That's a helper that EJS defines to make linking a little bit easier. It implements a lot of others too, which are documented here. To find out more about EJS, I suggest the EJS home page.
Template.js
A JavaScript template engine, simple, easy & extras, support webpack, and fis.
Provides a set of template syntax, the user can write a template block.
Writing a template:
Use a script tag of type="text/html" to store the template, or put it in a string:
<script id="tpl" type="text/html"> <ul> <%for(var z = 0; z < list.length; z++) {%> <li><%:=list[z].name%></li> <%}%> </ul> </script>
Rendering template:
var tpl = document.getElementById('tpl').innerHTML; template(tpl, { list: [ {name: "yan"}, {name: "haijing"} ] } );
Output results:
<ul> <li>yan</li> <li>haijing</li> </ul>
Each time incoming data, generate HTML fragments generated by the corresponding data, rendering different effects.
HandlebarsJS
Handlebars is one of the most popular templating engines and builds on top of Mustache. It provides the power necessary to let you build semantic templates effectively with no frustration.
Handlebars is largely compatible with Mustache templates. In most cases it is possible to swap out Mustache with Handlebars and continue using your current templates.
Anything that was valid in a Mustache template is valid in a Handlebars template. Handlebars add lots of helpers to Mustache. One of these is 'with'
, which is great for working with deep objects:
// with this template: var source = "{{#with author}} <h2>By {{firstName}} {{lastName}}</h2> {{/with}}"; var template = Handlebars.compile(source); var html = template({ author: { firstName: "Jack", lastName: "Franklin" } }); // returns: <h2>By Jack Franklin</h2>
Notice that the Handlebars compiler works slightly differenly. Firstly, you pass the template into 'Handlebars.compile', which then returns a function. You can call that, passing in the object containing the data, and then you get the HTML back. The '{{#with}}' helper takes an object and then within it allows you to refer to properties within that object. This means rather than doing:
{{ author.firstName}} {{author.lastName}}
We can do:
{{#with author}} {{firstName}} {{lastName}} {{/with}}
Which can save on typing, especially if you're doing it a lot.
Handlebars also provides an each helper:
var source = "{{#each people}} {{name}} {{/each}}"; var template = Handlebars.compile(source); var html = template({ people: [{ name: "Jack" }, { name: "Fred" }] }); // returns: "JackFred"
Handlebars it's also very easy to extend with your own methods
Tempo
Tempo is an easy, intuitive JavaScript rendering engine that enables you to craft data templates in pure HTML.
It Clears separation of concerns: no HTML in your JavaScript files, and no JavaScript in your HTML
Include the Tempo script:
<script src="js/tempo.js" type="text/javascript"></script> <script>Tempo.prepare("tweets").render(data);</script>
Compose the data template inline in HTML:
<ol id="tweets"> <li data-template> <img src="default.png" data-src="{{profile_image_url}}" /> <h3>{{from_user}}</h3> <p>{{text}}<span>, {{created_at|date 'HH:mm on EEEE'}}</span></p> </li> <li data-template-fallback>Sorry, JavaScript required!</li> </ol>
It Makes working with AJAX/JSON content a piece of cake, also It Works in Safari, Chrome, FireFox, Opera, and Internet Explorer 6+
Jade Templating
With the popularity of NodeJS and the number of web apps being built in it now, there's a lot of templating libraries out there designed to be used on the server. Jade templates are very different to any we've looked at so far, in that it depends hugely on indents and whitespace.
Here's a simple example:
// template.jade p | Hello, = name // JS jade.renderFile("template.jade", { name: "Jack" }, function(err, result) { console.log(result); // logs: Hello, Jack });
It's a bit difficult to understand at first, we indent the two lines below the 'p'
to denote that they exist within it. The '|'
is used to tell Jade that what's on that line is just plain text to output, and the '='
tells Jade to look for a variable named 'name'.
We can also do loops in Jade too:
each person in people li = person
Called with an array of names: '{ people: [ "Jack", "Fred" ]}'
, this will output:
<li>Jack</li> <li>Fred</li>
Jade is capable of a lot more - unlike the other template engines we've looked at, the idea with Jade is that you write the entire of your HTML in it. It can do things like output script tags:
script(type="text/javascript", src="myfile.js")
A good place to start is the Jade examples. You can download them and run them with Node to see the output and how Jade works.
ECT
ECT is a performance focused JavaScript template engine with embedded CoffeeScript syntax. His best features are:
- Excellent performance
- Templates caching
- Automatic reloading of changed templates
- CoffeeScript code in templates
- Multi-line expressions support
- Tag customization support
- Node.JS and client-side support
- Powerful but simple syntax Inheritance, partials, blocks
For example to generate a list, we need first these params:
{ title : 'Hello, world!', id : 'main', links: [ { name : 'Google', url : 'https://google.com/' }, { name : 'Facebook', url : 'https://facebook.com/' }, { name : 'Twitter', url : 'https://twitter.com/' } ], upperHelper : function (string) { return string.toUpperCase(); } }
Then the list template:
<% linkHelper = (link) -> %> <li><a href="<%- link.url %>"><%- link.name %></a></li> <% end %> <% if @links?.length : %> <ul> <% for link in @links : %> <%- linkHelper link %> <% end %> </ul> <% else : %> <p>List is empty</p> <% end %>
That will generate this result:
<ul> <li><a href="https://google.com/">Google</a></li> <li><a href="https://facebook.com/">Facebook</a></li> <li><a href="https://twitter.com/">Twitter</a></li> </ul>
Dot Dom
.dom is a tiny (512 byte) template engine that uses virtual DOM and some of react principles, .dom borrows some concepts from React.js (such as the re-usable Components and the Virtual DOM) and tries to replicate them with the smallest possible footprint, exploiting the ES6 javascript features. With such library you can create powerful GUIs in tight space environments, such as IoT devices, where saving even an extra byte actually matters!
Example with Stateless Component:
function Hello(props) { return H('div', `Hello ${props.toWhat}`); } R( H(Hello, {toWhat: 'World'}), document.body )
The library never exceeds the 512 bytes in size. It is heavily exploiting the ES6 specifications.
Template7
Template7 is a mobile-first JavaScript template engine with Handlebars-like syntax. It is used as a default template engine in Framework7. Template7 templates looks like Handlebars templates, it is like regular HTML but with embedded handlebars expressions:
First of all we need to deliver string template. For example, we can store in script tag:
<script id="template" type="text/template7"> <p>Hello, my name is {{firstName}} {{lastName}}</p> </script>
Now we need to compile it in JavaScript. Template7 will convert our template string to plain JavaScript function:
var template = $$('#template').html(); // compile it with Template7 var compiledTemplate = Template7.compile(template); // Now we may render our compiled template by passing required context var context = { firstName: 'John', lastName: 'Doe' }; var html = compiledTemplate(context);
Now, html variable will contain:
<p>Hello, my name is John Doe</p>
It is ultra-lightweight (around 1KB minified and gzipped) and blazing fast (up to 2-3 times faster than Handlebars in mobile Safari).
Bunny
BunnyJS is a modern Lightweight native (vanilla) JavaScript (JS) and ECMAScript 6 (ES6) browser library, package of small stand-alone components without dependencies: FormData, upload, image preview, HTML5 validation, Autocomplete, Dropdown, Calendar, Datepicker, Ajax, Datatable, Pagination, URL, Template engine, Element positioning, smooth scrolling and much more
the package of small stand-alone components without dependencies. It has No dependencies – can be used in any project anywhere anytime
Squirrelly
Squirrelly is a modern, configurable, and fast template engine implemented in JavaScript.
With Squirrelly, you can write templates that are blazing fast and can be rendered in milliseconds, server-side or client-side.
Squirrelly doesn't just limit you to HTML--you can use it with any language, and custom delimeters make it so there aren't parsing errors. It's also tiny (~2.5 KB gzipped), has 0 dependencies, and is blazing fast.
Simple Template example:
var myTemplate = "<p>My favorite kind of cake is: {{favoriteCake}}</p>" Sqrl.Render(myTemplate, {favoriteCake: 'Chocolate!'}) // Returns: '<p>My favorite kind of cake is: Chocolate!</p>
Conditionals:
{{if(options.somevalue === 1)}} Display this {{#else}} Display this {{/if}}
Loops:
{{each(options.somearray)}} Display this The current array element is {{@this}} The current index is {{@index}} {{/each}}
Squirrelly has a number of features that set it apart from the competition:
- It's faster than most templating engines
- It supports user-defined helpers and native helpers
- It supports filters
- It supports partials
- It supports custom tags (delimeters)
- It's incredibly lightweight: in comparison: the full version only weighs about 2.5 KB gzipped, compared to Pug's 237 KB and
- Handlebars' 21.5 KB
- It works with other languages than HTML
- It's not white-space sensitive
It works out of the box with ExpressJS and the full version weighs only ~2.5KB gzipped.
View on GithubConclusion
It is upon the developer to decide which JavaScript template engine presents him/her with the best development capabilities and features that come with each ecosystem. Developers are tasked to choose among other criteria performance and visually oriented preferences.
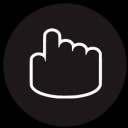
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…