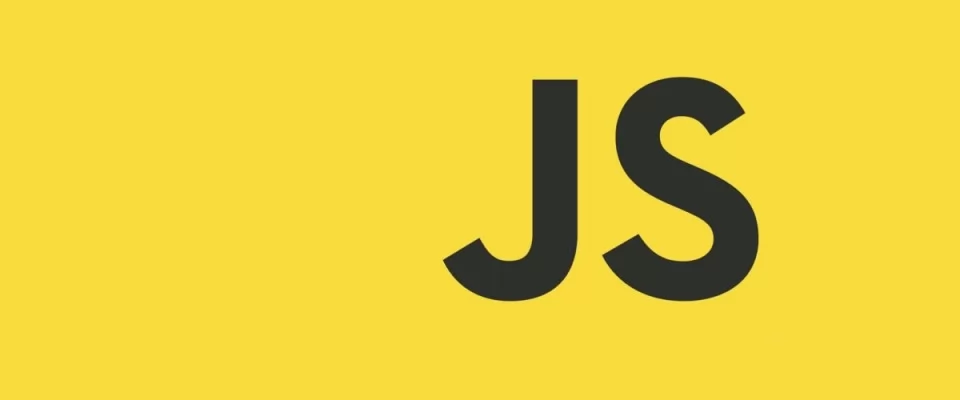
An easy tutorial on how to import one JS file into another using ES6
Until some years ago, it was not possible to import one js file or block of code from one file into another but since 2015, ES6 has introduced useful ES6 modules. With the module
standard, we can use import
and export
keyword and syntax given to include and export the module in JS respectively.
The static import
statement is used to import bindings which are exported by another module. Imported modules are in strict mode whether you declare them as such or not. The import statement cannot be used in embedded scripts unless such script has a type="module".
There is also a function-like dynamic import()
, which does not require scripts of type="module".
Backward compatibility can be ensured using attribute nomodule on the script tag.
How we include the js files:
index.html
<!DOCTYPE html> <html> <head> </head> <body> <script type="module" src="script2.js"></script> </body> </html>
script1.js
export default function Hello() { return "Hello buddy!"; }
script2.js
import { Hello } from './script1.js'; let val = Hello; console.log(val);
Run the above sample code blocks in your local system/local server, you will get the console output as: Hello buddy!
Code and Syntax Explanation of the code above:
In script1.js, we exported the module with the syntax of : export module
Similarly, in our case, we exported the function Hello
by writing the export
keyword in the function definition (line number 1 in script1.js). In script2.js, we imported that module by writing the following code/syntax :
import { Hello } from './script1.js';
Right now in script1.js, we have only one module to export but what if we had multiple modules to export? That is also possible with a similar syntax but with a slight modification:
Sample Code for multiple modules:
script1.js
export let Name = (name) => {return "My name is " + name;} export let Address = (address) => {return "i live in " + address;} export let Age = (age) => {return "my age is "+ age;}
script2.js
import { Name, Address, Age} from './script1.js'; let val = Name("John"); let age = Age(26); console.log(val + " and " + age);
In script1.js, we have three modules named Name, Address, and Age. In Script2.js, we imported those modules with similar but little different syntax.
import { Name, Address, Age} from './script1.js';
Note: we didn’t have { } in earlier import code because that was default export ( remember one file can have only one default export).
When we remove that default keyword in previous code of script1.js, we get an error in the console and that error looks like this:
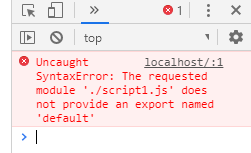
Infact there are two types of exports:
- Named Exports (Zero or more exports per module)
- Default Exports (One per module)
This is only a quick explanation, you can fond more examples at MDN web docs:
Import Statement Reference at MDN
Export Statement Reference at MDN
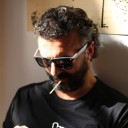
Luigi Nori
He has been working on the Internet since 1994 (practically a mummy), specializing in Web technologies makes his customers happy by juggling large scale and high availability applications, php and js frameworks, web design, data exchange, security, e-commerce, database and server administration, ethical hacking. He happily lives with @salvietta150x40, in his (little) free time he tries to tame a little wild dwarf with a passion for stars.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…