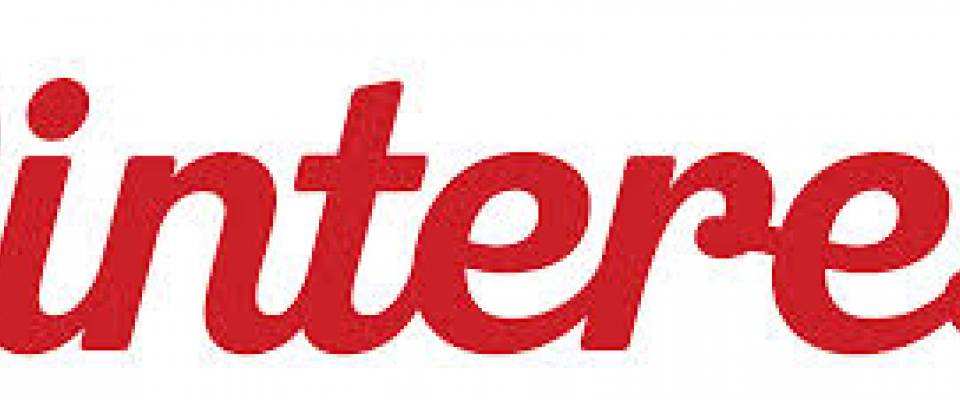
With all of the interest in Pinterest , we thought it would be a good idea to do a simple Pinterest sharing plugin for jQuery.
Let's start!
First we need to downloas the jQuery plugin boilerplate
(function( $ ) { $.fn.pinterest = function(options) { var settings = $.extend( { }, options); return this.each(function() { }); }; })( jQuery );
Boilerplate provides us with the ability to specify setting configurations.
Let's costumize Pinterest Image!
(function( $ ) { $.fn.pinterest = function(options) { var settings = $.extend( { 'button' : 'http://business.pinterest.com/assets/img/builder/builder_opt_1.png' }, options); return this.each(function() { }); }; })( jQuery ); $(document).on('ready', function(){ $('img#share').pinterest({ button: 'pinterest-alt.png'}); });
Now we can add some functionality. We have to update the "this.each" function to initialize each image and add some events. We’ve decided to have the icon hover over the image being shared. Therefore, we need to wrap the image in a span element that positioned ‘relative’, and we have to append the share image and position it absolutely.
(function( $ ) { $.fn.pinterest = function(options) { var settings = $.extend( { 'button' : 'http://business.pinterest.com/assets/img/builder/builder_opt_1.png' }, options); return this.each(function() { img = $(this); img.wrap('<span class="pin-it" style="position: relative;" />'); img.parent('span.pin-it').append('<img src="' + settings.button + '" alt="test pinterest" style="display: none;position: absolute; bottom: 20px; right: 20px;cursor: hand; cursor: pointer;" />''); }); }; })( jQuery ); $(document).on('ready', function(){ $('img#share').pinterest({ button: 'pinterest-alt.png'}); });
and:
(function( $ ) { $.fn.pinterest = function(options) { var settings = $.extend( { 'button' : 'http://business.pinterest.com/assets/img/builder/builder_opt_1.png' }, options); function on_click () { }; function on_hover_in() { $(this).siblings('img:first').show(500); }; return this.each(function() { img = $(this); img.wrap('<span class="pin-it" style="position: relative;" />'); img.parent('span.pin-it').append('<img src="' + settings.button + '" style="display: none;position: absolute; bottom: 20px; right: 20px;cursor: hand; cursor: pointer;" />'); img.hover(on_hover_in); img.siblings('img:first').on('click', on_click); }); }; })( jQuery ); $(document).on('ready', function(){ $('img#share').pinterest({ button: 'pinterest-small.png'}); });
Now, the on_click event looks like the following
function on_click () { img = $(this).siblings('img:first'); description = img.attr('title'); url = document.location; media = img.attr('src'); var pin_url = 'http://pinterest.com/pin/create/button/?url=' + encodeURIComponent( url ) + '&media=' + encodeURIComponent( media ) + '&description=' + encodeURIComponent( description ); window.open(pin_url, 'Pin - ' + description, 'menubar=no,toolbar=no,resizable=yes,scrollbars=yes,height=600,width=600'); $(this).hide(1000); };
It’s grabbing the image we want to share, and then reading it’s title and src attributes. It’s also getting the current pages url using document.location. The Pinterest share url has 3 parameters that we are interested in:
- url – the page we are sharing from
- media – the image we want to share
- description – the text we want to share with the image
We have to use also the encodeURIComponent function to make sure the values are properly escaped.
One final thing we need to allow for is if the image src url is relative or not.
function getUrl(src){ var url = document.location.toString(); var http = /^https?:\/\//i; if (!http.test(src)) { if(src[0] == '/'){ url = url.substring(0, url.lastIndexOf('/')) + src; } else { url = url.substring(0, url.lastIndexOf('/')) + '/' + src; } } else { url = src; } return url; };
Download source: Creating a simple jQuery plugin for Pinterest
Thanks to: developerdrive.com
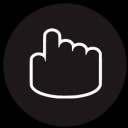
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Hidden Gmail codes to find a lost e-mail
If you have a lot of emails in Gmail, there are a few codes that will help you find what you need faster and more accurately than if you do…
How to download an email in PDF format in Gmail for Android
You will see how easy it is to save an email you have received or sent yourself from Gmail in PDF format, all with your Android smartphone. Here's how it's…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
How to make a multilingual website without redirect
Today, we're going to talk about how to implement a simple language selector on the basic static website, without the need of any backend or database calls or redirection to…
Starting with Bootstrap-Vue step by step
Today we will show you how to use BootstrapVue, describe the installation process and show basic functionality. The project’s based on the world's most popular CSS framework - Bootstrap, for building…
Bootstrap 5 beta2. What offers?
Since the release of the Bootstrap 4 is three years, in this article we will present what is new in the world’s most popular framework for building responsive, mobile-first sites.…
Graphic design and its impact on Web Development
In today's article we will explain the concept of graphic design, its fundamentals and what it brings into web development. Graphic design is applied to everything visual, believe or not,…
Validating HTML forms using BULMA and vanilla JavaScript
Today we are going to write about contact forms and how to validate them using JavaScript. The contact form seems to be one of the top features of every basic home…
A Java approach: While loop
Hello everyone and welcome back! After having made a short, but full-bodied, introduction about cycles, today we are finally going to see the first implementations that use what we have called…
A Java approach: The Cycles - Introduction
Hello everyone and welcome back! Until now, we have been talking about variables and selection structures, going to consider some of the fundamental aspects of these two concepts. Theoretically, to…
A Java Approach: Selection Structures - Use Cases
Hello everyone and welcome back! Up to now we have been concerned to make as complete an overview as possible of the fundamental concepts we need to approach the use…