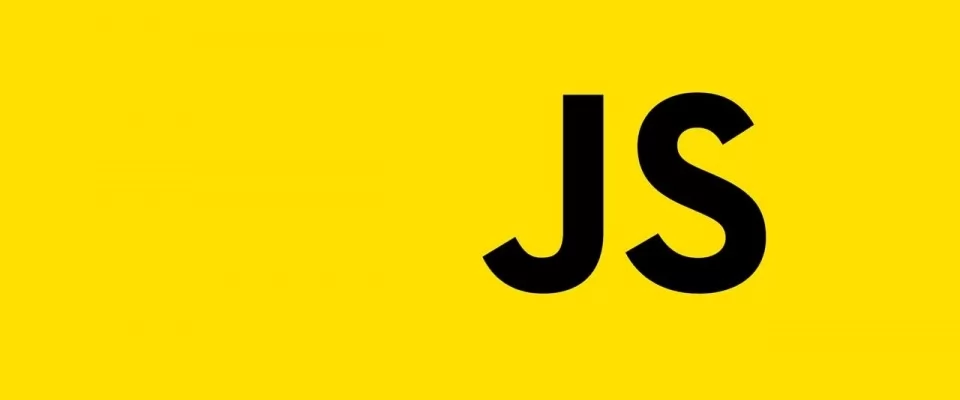
This article will show that the prominent JavaScript array functions are .map()
, .filter()
, and .reduce()
, and will then go through examples of instances in which .every()
and .some()
would save computing power over the more prominent solutions.
You will learn helpful methods in JavaScript Array, an array is one of the most common data structures that we use a lot. I will be going over array methods that are going to use a lot as a programmer. These array methods will make your life easier in your javascript project. Here is the list of all array methods provided by Array class. Today we are going to discuss the most important array methods
- map()
- filter()
- reduce()
- reverse()
- reduceRight()
- every()
- some()
- includes()
- concat()
- sort()
- slice()
- splice()
- pop()
- shift()
- push()
- unshift()
map()
The map() method creates a new array containing the results of calling a provided function on every element in the calling array
The callback function runs once for each element in an array, in order, and constructs a new array from the results. It is invoked only for indexes of the array which have assigned values (including undefined). Please note that it is not called for missing elements of the array
map() is invoked with a callback function with the following three arguments:
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, map method was called upon
const heros = [{ id: 1, name: "Iron Man", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 2, name: "Batman", fullName: "Terry McGinnis", publisher: "DC Comics" }, { id: 3, name: "Spider-Man", fullName: "Peter Parker", publisher: "Marvel Comics" }, { id: 4, name: "Venom", fullName: "Eddie Brock", publisher: "Anti-Venom" }, { id: 5, name: "Deadpool", fullName: "Wade Wilson", publisher: "Evil Deadpool" }, { id: 6, name: "Thanos", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 7, name: "Thor", fullName: "Thor Odinson", publisher: "Marvel Comics" }, { id: 8, name: "Hulk", fullName: "Bruce Banner", publisher: "Marvel Comics" }, { id: 9, name: "Flash", fullName: "Jay Garrick", publisher: "DC Comics" }, { id: 10, name: "Wolverine", fullName: "Logan", publisher: "Marvel Comics" }]; const transformedHeros = heros.map((element) => { return { name: element.name, publisher: element.publisher, isFamous: true, } }); console.log(transformedHeros); // OUTPUT // [{ name: 'Iron Man', publisher: 'Marvel Comics', isFamous: true }, { name: 'Batman', publisher: 'DC Comics', isFamous: true }, { name: 'Spider-Man', publisher: 'Marvel Comics', isFamous: true }, { name: 'Venom', publisher: 'Anti-Venom', isFamous: true }, { name: 'Deadpool', publisher: 'Evil Deadpool', isFamous: true }, { name: 'Thanos', publisher: 'Marvel Comics', isFamous: true }, { name: 'Thor', publisher: 'Marvel Comics', isFamous: true }, { name: 'Hulk', publisher: 'Marvel Comics', isFamous: true }, { name: 'Flash', publisher: 'DC Comics', isFamous: true }, { name: 'Wolverine', publisher: 'Marvel Comics', isFamous: true }]
When you need to transform input array into a new array use map() method
filter()
The filter() method creates a new array with all elements that pass the test implemented by the provided function
filter() is invoked with a callback function with the following three arguments:
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, filter method was called upon
const heros = [{ id: 1, name: "Iron Man", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 2, name: "Batman", fullName: "Terry McGinnis", publisher: "DC Comics" }, { id: 3, name: "Spider-Man", fullName: "Peter Parker", publisher: "Marvel Comics" }, { id: 4, name: "Venom", fullName: "Eddie Brock", publisher: "Anti-Venom" }, { id: 5, name: "Deadpool", fullName: "Wade Wilson", publisher: "Evil Deadpool" }, { id: 6, name: "Thanos", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 7, name: "Thor", fullName: "Thor Odinson", publisher: "Marvel Comics" }, { id: 8, name: "Hulk", fullName: "Bruce Banner", publisher: "Marvel Comics" }, { id: 9, name: "Flash", fullName: "Jay Garrick", publisher: "DC Comics" }, { id: 10, name: "Wolverine", fullName: "Logan", publisher: "Marvel Comics" }]; // GET HEROS PUBLISHED BY MARVEL COMICS const transformedHeros = heros.filter((element) => {return element.publisher === 'Marvel Comics'}); console.log(transformedHeros); // OUTPUT // [{ id: 1, name: "Iron Man", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 3, name: "Spider-Man", fullName: "Peter Parker", publisher: "Marvel Comics" }, { id: 6, name: "Thanos", fullName: "Tony Stark", publisher: "Marvel Comics" }, { id: 7, name: "Thor", fullName: "Thor Odinson", publisher: "Marvel Comics" }, { id: 8, name: "Hulk", fullName: "Bruce Banner", publisher: "Marvel Comics" }, { id: 10, name: "Wolverine", fullName: "Logan", publisher: "Marvel Comics" }]
When you need to filter input array into a new array use filter() method
reduce()
The reduce() function is used when the programmer needs to iterate over a JavaScript array and reduce it to a single value. A callback function is called for each value in array from left to right. The value returned by callback function is available to next element as an argument to its callback function. In case the initialValue is not provided, reduce’s callback function is directly called on 2nd elementwith 1st element being passed as previousValue. In case the array is empty and initial value is also not provided, a TypeError is thrown.
The reducer function takes four arguments:
- accumulator - The accumulator accumulates callback's return values. It is the accumulated value previously returned in the last invocation of the callback
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, reduce method was called upon
const numbers = [10, 7, 5]; // Sum of all array elements const sum = numbers.reduce( (accumulator, currentValue) => { console.log('accumulator:', accumulator) return accumulator + currentValue; }, 0 ) console.log('sum:', sum) // OUTPUT // accumulator: 0 accumulator: 10 accumulator: 17 22
Let's breakdown what happens in the reduce method using the above example. We have passed the reducer function and initial value of the accumulator as an argument. Let's see what happened when we looped over numbers array
- In first iteration, The initial value of accumulator as 0(second argument of reduce method). 'accumulator: 0' got logged. In second line of reducer function currentValue(10) got added in accumulator(accumulator = 0 + 10)
- In second iteration, The accumulator now has value 10 so 'accumulator: 10' got logged. In second line of reducer function currentValue(7) got added in accumulator(accumulator = 10 + 7)
- In third iteration, The accumulator now has value 17 so 'accumulator: 17' got logged. In second line of reducer function currentValue(5) got added in accumulator(accumulator = 17 + 5)
- After array iteration is complete, reduce function returned the accumulator(22) and stored in sum variable and 'sum: 22' got logged
Please note the following mistakes while using reduce method
- If you don’t pass in an initial value, reduce will assume the first item in your array is your initial value
- You must return something for the reduce function to work. Always double-check and make sure that you’re returning the value you want
When to use => when you want to map and filter together and you have a lot of data to go over use reduce() method
reverse()
.reverse() is used to reverse a JavaScript array. Reverse() function modifies the calling array itself and returns a reference to the now reversed array.
var arr = [1,2,3,4,5,6,7,8,9,10]; arr.reverse(); console.log(arr); //Output : [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
reduceRight()
The reduceRight() method executes a reducer function on each element of the array (from right-to-left), resulting in single output value
In short, reduceRight() works the same as the reduce(), the only difference is in order of processing array elements
The reducer function takes four arguments, the same as reduce() method:
- accumulator - The accumulator accumulates callback's return values. It is the accumulated value previously returned in the last invocation of the callback
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, reduceRight method was called upon
const numbers = [10, 7, 5]; // Sum of all array elements const sum = numbers.reduceRight( (accumulator, currentValue) => { console.log('accumulator:', accumulator) return accumulator + currentValue; }, 0 ) console.log('sum:', sum) // OUTPUT // accumulator: 0 accumulator: 5 accumulator: 12 sum: 22
I'm not going over how this code worked as it works the same as reduce() method
When to use => When you need to reduce an array from Right to left use reduceRight() method
every()
every() function is used when you need to validate each element of a given array. It accepts a callback function as an argument which is called for each element of the array. The callback function has to return either true or false. If all elements of the array satisfy the validation function and thus callback function returns true on all elements of the array, then it returns true. Otherwise, it returns false, as soon as it encounters the first element which does not satisfy the validator function.
every() is invoked with a callback function with the following three arguments:
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, every method was called upon
const numbers1 = [10, 0, -5, 8, 9]; // Check if every number in array is positive console.log(numbers1.every((e) => e > 0)) // false const numbers2 = [35, 50, 52, 48, 39]; // Check if every number in array is greater than 30 console.log(numbers2.every((e) => e > 30)) // true
When to use => When you need check if every array element/object satisfies a particular condition, use every() method
some()
The some() method tests whether at least one of elements in the array pass the test implemented by the provided function. It returns a boolean value
some() is invoked with a callback function with following three arguments:
- currentValue - The current element being processed in the array
- indexOptional(Optional) - The index of the current element being processed in the array
- array (Optional) - The array, some method was called upon
const numbers1 = [10, 0, -5, 8, 9]; // Check if some numbers in the array are negative console.log(numbers1.some((e) => e < 0)) // true const numbers2 = [35, 50, 52, 48, 39]; // Check if some numbers in the array are greater than 100 console.log(numbers2.some((e) => e > 100)) // false
When to use => When you need check if at least one array element/object satisfies a particular condition, use some() method
includes()
The includes() method case-sensitively determines whether an array contains a specified element. It returns true if the array contains the element otherwise false.
includes() can be invoked with the following two arguments:
- element - The element to search for
- startIndex(Optional) - Default 0. At which position in the array to start the search
const fruits = ["Banana", "Peach", "Apple", "Mango", "Orange"]; console.log(fruits.includes("Mango")); // true console.log(fruits.includes("Jackfruit")); // false
When to use => When you need to find if a value exists in an array
concat()
The concat() method is used to merge two or more arrays. It returns a new array containing all the elements of calling and called arrays
concat() can be invoked with the following argument:
- nArray(Optional) - one or more Arrays and/or values to concatenate
const num1 = [1, 2, 3]; const num2 = [4, 5, 6]; const num3 = [7, 8, 9]; console.log(num1.concat(num2, num3)); // [1, 2, 3, 4, 5, 6, 7, 8, 9] console.log(num1.concat(num3)); // [1, 2, 3, 7, 8, 9]
When to use => When you need to merge two or more arrays without modifying existing arrays
sort()
The sort() method sorts array elements. By default, alphabetically sorts the elements. If you want numerically sort the elements we can do it with the help of comparator function
const fruits = ["Banana", "Peach", "Apple", "Mango", "Orange"]; console.log(fruits.sort()); // [ 'Apple', 'Banana', 'Mango', 'Orange', 'Peach' ] const numbers = [100, 1, 40, 5, 25, 10]; console.log(numbers.sort()); // [1, 10, 100, 25, 40, 5] console.log(numbers.sort((a, b) => { return a - b })); // [ 1, 5, 10, 25, 40, 100 ]
Let's see what happened in above examples
- The first example is pretty straight forward. fruits array got sorted alphabetically
- In the second example, [1, 10, 100, 25, 40, 5] got printed. That's weird. It happened because array got sorted alphabetically not numerically. eg: "25" is bigger than "100", because "2" is bigger than "1"
- In the third example, We corrected the above example with the help of comparator function
When to use => When you need to sort elements of an array
slice()
The slice() method returns the selected elements in a new array
slice() is invoked with the following 2 arguments:
- startIndex(Optional) - An integer that specifies where to start the selection. You can use negative numbers to select from the end of an array. If ignored, it acts like "0"
- endIndex(Optional) - An integer that specifies where to end the selection. If ignored, all elements from the start position and to the end of the array will be selected. You can use negative numbers to select from the end of an array
const fruits = ["Banana", "Peach", "Apple", "Mango", "Orange"]; console.log(fruits.slice(2, 4)); // [ 'Apple', 'Mango' ] console.log(fruits.slice(3)); // ['Mango', 'Orange']
When to use => When you need to return the selected elements into a new array
splice()
The splice() method adds/removes elements to/from an array, and returns the removed elements. It is a very handy method for array elements modifications
slice() is invoked with the following arguments:
- index - An integer that specifies at what position to add/remove elements, You can use negative values to specify the position from the end of the array
- howManyToRemove (Optional) - The number of elements to be removed. If set to 0, no elements will be removed
- item1, ... , itemX(Optional) - The new elements to be added to the array
const fruits1 = ["Banana", "Peach", "Apple", "Mango", "Orange"]; // At position 2, add the new elements, and remove 1 item fruits1.splice(2, 1, "Lemon", "Kiwi"); console.log(fruits1) // [ 'Banana', 'Peach', 'Lemon', 'Kiwi', 'Mango', 'Orange' ] // At position 1, remove 2 elements const fruits2 = ["Banana", "Peach", "Apple", "Mango", "Orange"]; fruits2.splice(1, 2); console.log(fruits2) // [ 'Banana', 'Mango', 'Orange' ]
When to use => When you need to add/remove elements to/from an array
pop()
The pop() method removes the last element from an array and returns that element. This method changes the length of the array
const animals = ['rat', 'cat', 'bat', 'snake', 'dog']; console.log(animals.pop()); // "dog" console.log(animals); // ['rat', 'cat', 'bat', 'snake']
When to use => When you need remove last element of an array, use map() method
shift()
The shift() method removes the first element from an array and returns that element. This method changes the length of the array
const animals = ['rat', 'cat', 'bat', 'snake', 'dog']; console.log(animals.shift()); // "rat" console.log(animals); // ['cat', 'bat', 'snake', 'dog']
When to use => When you need to remove the first element of an array, use shift() method
push()
The push() method adds a new element to the end of an array and returns the new length. As array in JavaScript are mutable object, we can easily add or remove elements from the Array. And it dynamically changes as we modify the elements from the array.
const animals = ['rat', 'cat', 'bat', 'snake', 'dog']; animals.push('bull'); console.log(animals); // ['rat', 'cat', 'bat', 'snake', 'dog', 'bull']
When to use => When you need add an element at the end of an array use push() method
unshift()
The unshift() method adds a new element to the beginning of an array and returns the new length
const animals = ['rat', 'cat', 'bat', 'snake', 'dog']; animals.unshift('bull'); console.log(animals); // ['bull', 'rat', 'cat', 'bat', 'snake', 'dog'];
When to use => When you need to add an element at the beginning of an array, use unshift() method
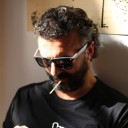
Luigi Nori
He has been working on the Internet since 1994 (practically a mummy), specializing in Web technologies makes his customers happy by juggling large scale and high availability applications, php and js frameworks, web design, data exchange, security, e-commerce, database and server administration, ethical hacking. He happily lives with @salvietta150x40, in his (little) free time he tries to tame a little wild dwarf with a passion for stars.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…