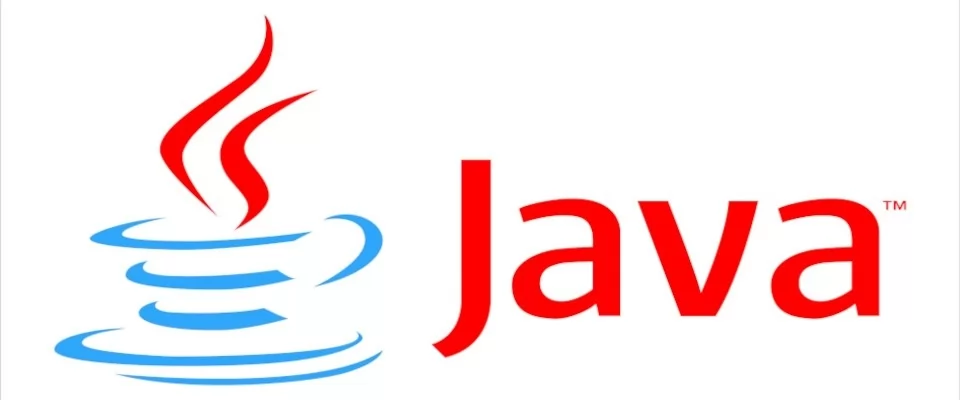
Hello everyone and welcome back! The previous times we have introduced the concept of variable, trying to define some basic concepts about it.
However, some situations suggest that the concept of variable alone is not enough to solve all the possible situations that may arise in front of us. A very banal example could be to understand if a number is odd or even. We immediately understand how the concept of variable alone cannot allow us to solve this problem, because after all, programming means solving problems, doesn't it?
Throughout history, therefore, constructs have been developed and introduced that allow us to vary the flow of execution of the program itself. Let's try to understand better.
First of all, let's see a pseudo-code for solving the problem.
if the number is even do something otherwise do something else
You will immediately notice that there are two possible program executions.
The first involves the case in which the number is even, then you execute the instructions I have indicated, in a very informal way, with "do something". The second case, on the other hand, is the case of the odd number, where the actions performed are those named with "do something else".
The first important thing to say is that the two blocks of instructions are executed in an exclusive way. This means that if one group is executed then the other is not executed, and vice versa. This feature is fundamental precisely because the program execution flow is no longer one, but can have multiple developments. There are many developments, but they all have to be foreseen.
The if-else construct
There are different decision constructs. The first and most basic is the if-else construct. In Java, the syntax for expressing it is as follows:
if(condition is true){ //Instructions to be carried out if the condition is true } else { //Instructions to be carried out if the condition is false }
You can easily see that two fundamental blocks are highlighted. The if block and the other block. Let's see how the fulcrum of the whole construct is the condition. This notion introduces us to a new type of value: the Boolean value. Basically, condition can assume only two possible values: true or false.
We will see next time how Boolean values work.
Let's now consider the solution to the problem that was first posed regarding odd and even numbers.
int a = k; if(a % 2 == 0){ System.out.println("Even"); } else { System.out.println("Odd"); }
The solution is quite simple, as the fundamental skeleton of the problem is the if-else construct. It is up to the programmer to choose what to insert inside the two blocks.
A small note about the notation I used: the k of the variable initialization is a compact way to say that that k can be replaced with any integer value.
This example is ideal for introducing a new operator: the %. This operator allows us to calculate the rest of a division. When we write a % b we are then calculating the rest of the division of a for b. For example, writing 5 % 2 results in 1, because 1 is the rest of the division between 5 and 2.
The if-elseif-else construct
Sometimes it can happen that there are a number of conditions that have to be checked exclusively. Therefore, having, for example, three conditions c1, c2 and c3, check them in an exclusive signigic way that, if c1 is true, neither c2 nor c3 are checked. If c2 is true, c3 is not checked. How to do this?
An inexperienced reader could say that a series of ifs could be the solution. You could then write a solution similar to the following
if(c1 true){ // do something }else{ if(c2 true){ //do something else }else{ if(c3 true){ //do something else } } }
This solution works without a shadow of a doubt, but it is still unnecessarily complex and very unattractive.
The if-elseif-else construct comes to our aid. Let's see the syntax:
if(true condition){ }else if(other true condition){ }else{ }
So let's see the introduction of a new block of code, defined by the else if section. This section is evaluated only if the if condition is false. Let's see a possible example.
The problem to solve is the following: given a variable, print it if it represents a positive number, print the string "zero" if its value is equal to zero and print its value multiplied by two if it is a negative value.
A possible solution is this.
int a = k; if(a > 0){ System.out.println(a); }else if(a == 0){ System.out.println("zero"); }else{ System.out.println(a*2); }
As before, a = k means that k can be replaced with any integer value.
One question that may arise is: how many more branches if I can add? The answer is: as many as you want. Generally, there is a tendency to contain the number, as the code becomes unreadable and unattractive as the else if grows. We'll see later on that you tend to opt for a different solution, which tends to make the code more readable and clearer, regardless of the growth of the conditions to be checked.
If construct
Left for last, the construct if is the elementary brick at the base of the two shown above. The concept is really simple. If a given condition is true, then I perform actions and then continue with the normal flow of the program.
The syntax is the following one.
if(true condition){ //code }
A possible example is the following: given a variable, print it and if it is even add 1.
int a = k; if(a%2 == 0){ a = a + 1; } System.out.println(a);
We therefore see that it is only possible to perform operations in certain cases. In this way, you can vary the behaviour of the program according to the configuration of the variables at that given moment.
This introduction may seem rather theoretical, but later we will see some examples that allow us to see some situations of application of this concept.
A bit of theory: the configurations of the variables
The concept of variable configuration is indispensable to understand in order to fully grasp the meaning of some sentences.
When we refer to a configuration, we are referring to the set of variables and values that those variables have at a specific time of code execution.
If we want to somehow represent a configuration of variables, we could use a notation like this: { variable1 = valueVariable1, variable2 = valueVariable2, ... , variabilen = valueVariabilen }.
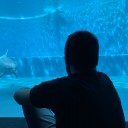
Alessio Mungelli
Computer Science student at UniTo (University of Turin), Network specializtion, blogger and writer. I am a kind of expert in Java desktop developement with interests in AI and web developement. Unix lover (but not Windows hater). I am interested in Linux scripting. I am very inquisitive and I love learning new stuffs.
Related Posts
How to Use ChatGPT to automatically create Spotify playlists
We will explain, step by step, how you can create Spotify playlists using ChatGPT. The arrival of ChatGPT, the chatbot based on OpenAI's natural language model, has revolutionized the world of…
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Hidden Gmail codes to find a lost e-mail
If you have a lot of emails in Gmail, there are a few codes that will help you find what you need faster and more accurately than if you do…
How to download an email in PDF format in Gmail for Android
You will see how easy it is to save an email you have received or sent yourself from Gmail in PDF format, all with your Android smartphone. Here's how it's…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…