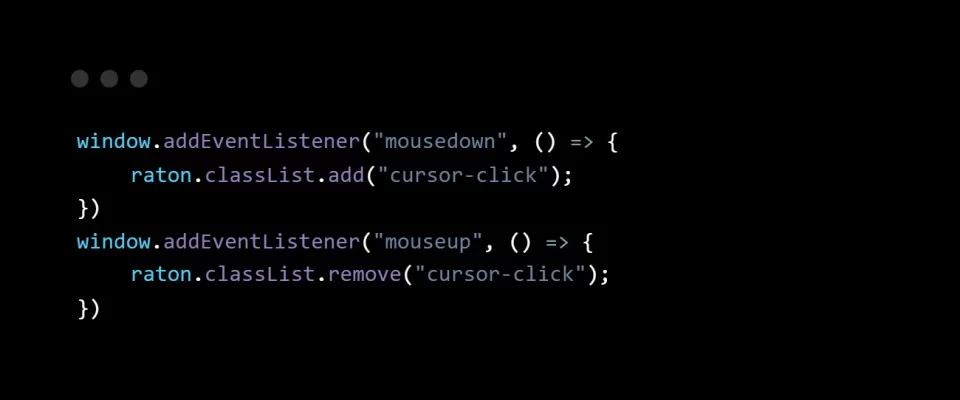
When I started browsing different and original websites to learn from them, one of the first things that caught my attention was that some of them had their own cursors, so I investigated how to incorporate it into my website.
Today I bring you a simple version of a custom cursor so that you can use it as a base and so that you can begin to play until you get to have the cursor you want.
The html is very simple, it is a simple div with the class you want to put in my case will be "cursor" and I have added a list to have something and see how the cursor interacts.
<body> <div class="cursor"></div> <ul> <li>Enlace 1</li> <li>Enlace 2</li> <li>Enlace 3</li> <li>Enlace 4</li> </ul> </body>
After having the html we have to add the css of our cursor, it is a simple black circle with absolute position, I have added a transition to him for when we add interactions to him it is seen with a small short transition.
.cursor { width: 2rem; height: 2rem; border: solid 2px #000000; border-radius: 50%; position: absolute; transform: translate(-50%, -50%); pointer-events: none; transition: all ease 0.1s; }
Now that we have the css we go with the javascript so that we can use that circle like cursor, we make a variable with the div of the cursor and I have made another with the li that I will use them as if they were links.
To the cursor we put a function to move that calculates the horizontal and vertical position of the cursor and applies it to the div that we have created positioning it, that is why it is important that had absolute position, if you look at the css also had a translate, what makes this translate is that the position of the cursor is in the center of the div that we have created, if we did not put it would be at the top left, you can try it if you want and if you do not want it in the center position it as you want.
let raton = document.querySelector(".cursor"); let enlaces = document.querySelectorAll("li"); window.addEventListener("mousemove", moveCursor); function moveCursor(e) { raton.style.top = e.pageY + "px"; raton.style.left = e.pageX + "px"; }
Once we check that our cursor is working and is right where the default cursor is, we would have to remove the cursor that is by default, it is very simple, simply remove the cursor throughout the web, it's that easy.
*{ cursor: none; }
And finally we are going to add the effect of click and hover. I have made a class in css of how I want the cursor to be when I click or pass over an element that can be clicked.
.cursor-click { width: 1rem; height: 1rem; border: solid 3px #27e6bc; background-color: hsla(167, 79%, 53%, 0.4); } .cursor-hover { border: solid 3px #27e6bc; }
I have also applied css to the li and a hover to see how everything interacts as a whole.
li { font-size: 3rem; list-style: none; margin: 3rem; text-align: center; } li:hover { color: rgb(187, 255, 0); }
First let's see the click effect, just add two simple functions, adding the click class on click and removing it when the mouse is released.
window.addEventListener("mousedown", () => { raton.classList.add("cursor-click"); }); window.addEventListener("mouseup", () => { raton.classList.remove("cursor-click"); });
Now we will add the hover to the li elements, you can add it to whatever you want, but if there are going to be many different elements I recommend you to make a class and add it to all the elements that you want to interact in this way with the cursor.
Simply we make a for each with all the elements and we add the 2 functions as before, one of over to add the class when being on top of the element, and another of out to remove that class when leaving that element.
enlaces.forEach(function (element) { element.addEventListener("mouseover", function () { raton.classList.add("cursor-hover"); }); element.addEventListener("mouseout", function () { raton.classList.remove("cursor-hover"); }); });
And we would already have the basics for our cursor to work, now it's time to try to make it more personal or to your style and try different things like adding a svg, put elements inside the cursor div and apply some filter, but from here it's up to your imagination.
I give you a link with the example and the code Here so it will be easier for you to start trying to customize your cursor.
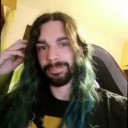
Nadal Vicens
Desde niño apasionado de los videojuegos, gracias a ello me ha interesado la tecnología, y he acabado dentro del mundo de la programación, donde puedo estar horas sin que me de cuenta. Interesado en aprender tanto de la parte de tecnología como de diseño. No podría vivir sin videojuegos, café y pizza.
Related Posts
Alternative tools for graphic design
There are many people today who only use the following for design purposes Canva as it is a really popular software and website and there is no denying that it…
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…