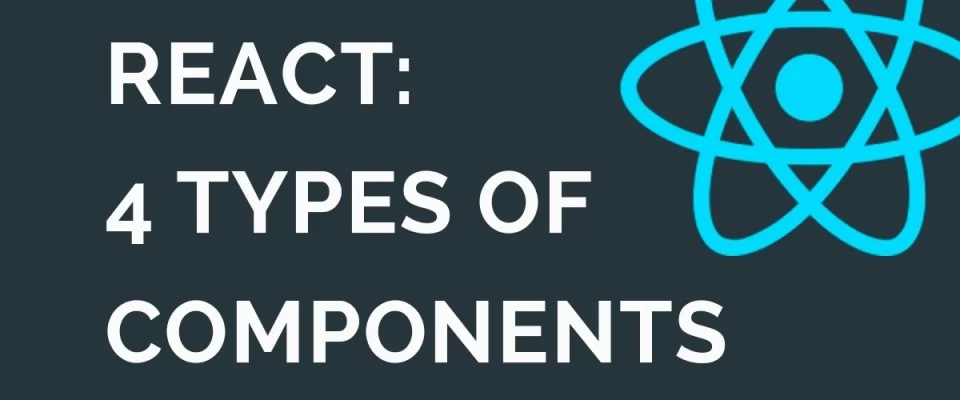
If you have already worked with React, you will probably have found terms like "dumb components", "stateless components" or "high order components" which serve to describe different ways of defining and creating components in an application.
The aim of this article is to explain the different types of components we can create in React and how we can use them to make our code easier to understand and maintain.
From zero
When we create a project in React by means of the create-react-app instruction we will start from a base component in which different functionalities and characteristics are grouped like the render function, the props, its own state or the lifecycle events or its own context:
However, the components that we will normally use will only use a part of all those API's; in fact, we can separate them into two big groups:
1. Those components that use the render function along with the props and the associated context
2. The components that use the render function, the state and the lifecycle events
It is from this point that the first two types of components arise.
Presentational components
This type of components, probably the most basic that we can define, and that is also known as stateless components, is only in charge of painting the elements based on the props received by the other components where they appear embedded. Hence, they also receive the nickname of stateless since they themselves do not store any kind of state, limiting themselves to presenting the data on screen. In fact, we will often define them directly as functions that return the corresponding HTML without the need to extend the React.Component class.
The most obvious advantage of separating these components from the rest is the ability to reuse them whenever you want without having to write the same code over and over again.
Example:
const Box = props => {props.name}
Container components
On the other hand, container components are those components that do use the API state to establish the logic and operation of our application, hence they are also known as state components.
This type of components, therefore, will be in charge of making calls to external API's, connecting to Redux or establishing the logic to be carried out depending on the actions that the user carries out on the interface.
Example:
class ContainerBox { constructor() { super(); this.state = { name: "Pluto" } } render() { return} }
As you can see, the previous Box component can be reused wherever we want, so it's very easy to reuse code while delegating logic to the containers.
However, how could we also reuse our ContainerBox component in the same way we have done with the Box component? This is where the third type of component (or pattern) comes in.
High order components
When we speak in React of high order components, we speak of components that receive a component as a parameter and return a new component, usually encapsulating the received component with additional functionality. In fact, this pattern is used in the react-redux
library when we create a component using the connect(MyComponent)
instruction.
Using this pattern can be confusing at first but the moment we start to feel comfortable with it is one of the most powerful we can employ in the design of our application.
Returning to our example, to define a high order component on our ContainerBox
we could write the following code:
function ContainerBoxHoc(AnyBox) { return class ContainerBox extends React.Component { constructor() { super(); this.state = { name: “Pluto” } } render() { return} } } const ContainerBox = ContainerBoxHoc(Box); function SpanBox = props => {props.name} const OtherContainerBox = ContainerBoxHoc(SpanBox)
As you can see, we can create as many ContainerBox as we want and each of them can paint the type of Box we need. Logically this is a very simple example, but if you browse through the official documentation you will find other more complex ones where you can see the advantages of the high ordered components.
Render props
Finally, the last type of component or pattern (I prefer in this case to use this last term) that we can use when defining the structure of our application is what is known as render props.
The characteristic of this type of components is that they receive a function through the props (which returns an element of React) and that will be the one that they use inside their own render function to paint on screen. For example, our component ContainerBox could be defined as follows:
class ContainerBox extends React.Component { constructor() { super(); this.state = { name: “Pluto” } } render() { return this.props.renderBox(this.state); } } const Box = props => < div >{props.name}< / div > const ContainerBoxWithBox = ( < ContainerBox renderBox={state => (< Box {…state} />)} /> );
As you can see in this case it is an external function to the component that establishes what is painted and how it is painted, limiting the ContainerBox component to establish the rest of the logic and use this property as a return value of its own render
function.
Conclusion
Knowing these 4 types of components (or patterns) you will be able to raise the structure of your application in a way that will allow you to reuse many components and make the code much more maintainable, something that in large applications is thankful.
If you are interested in learning more about the different components we recommend the following talks:
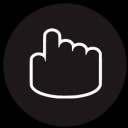
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
What are React Hooks and what problems they solve
Working with React, - and before the release of Hooks in version 16.8 - there was always the possibility to create components in three different ways based on a number of…
Is JavaScript good for machine learning?
One of the things you always hear when you are talking to someone related to the M.L. world is that, one must learn Python because the vast majority of the…
Angular vs React vs Vue: Which is the Best Choice?
With the growing popularity of Vue, Angular and React as frameworks and libraries for the web and app development, a constant doubt is which of these 3 we should learn,…
Why is React so popular as a JavaScript library?
If you want to know why React is so successful as a JavaScript library, we need to talk about its main features to find out what it is currently used…
Vue.js 3.0: What are Hooks and how they work
This article introduces an experimental Vue feature called Hooks. Before you start This post is suited for developers of all stages including beginners. Here are a few things you should already have…
Styling React components: recommendations and suggestions
With CSS we can set the presentation of a document through the rules that control the formatting of an element on a web page. Using CSS techniques, we can make…
Best Open-Source Javascript Date Picker Plugins and Libraries
For date pickers, selecting menus is a common choice as they are simple to set up. But I suppose our customers deserve better after centuries of using MM / DD…
Highlights from the JavaScript Style Guide of Google
For anyone who is not already acquainted with it, Google provides a style guide for writing JavaScript that gives the best stylistic practices for writing clean, understandable code (what Google…
Which Javascript frameworks will rule in 2019?
In this article, we will review and analyze the 2018 State of JavaScript report to later focus attention on frameworks that will be at the center of attention in 2019. Let's…
Async/Await how they really work: an example
Promises give us an easier way to deal sequentially with asynchrony in our code. This is a welcome addition, given that our brains are not designed to deal efficiently with…
Best 5 Frontend Development Topics in 2019
Ah, a new year. A time of positivity, a time to restart. For new objectives and proposals. And in the world of developers, to look at the scenery and decide…
ES2019 JavaScript and its new features
ECMAScript 2019 has finished and rolled out new exciting features for developers. The proposals which have been accepted and reached stage 4 by the TC39 committee would be included in…