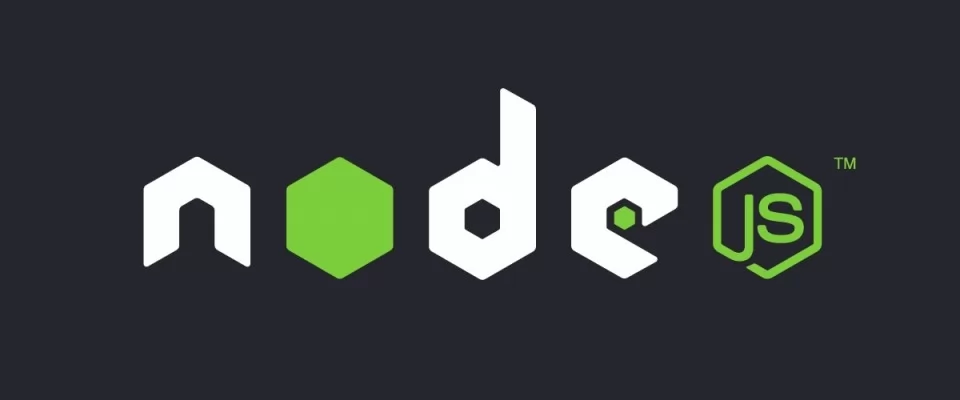
How to install and how to use Node.js and npm package manager
In this tutorial we will see how to install and use both Node.js and the npm package manager. In addition, we will also create a small sample application. If you have ever used or thought about using a JavaScript-based development tool, you have probably noticed that you need to install both Node.js and npm.
Some examples of these tools are Grunt, Gulp, Sass, Less or Webpack, among many others.
Requirements for this tutorial: This tutorial does not require you to have any prior knowledge except for one, and that is that you will need some minimal knowledge about the command line.
If you don't know how to use it, see the following tutorial, which explains how to use the command line on Linux, macOS and Windows.
In addition to learning what Node.js and npm are, you will also learn how to configure both tools and how to create a project with them.
What is Node.js
JavaScript began as a language whose utility was to be used client-side; that is, scripts programmed in this language are processed in the user's browser. However, with the appearance of Node.js, it also began to be used on the server side. Node.js is a JavaScript runtime environment that enables the creation of networked applications.
By default, Node.js supports multiple concurrent connections, so it is very common to use it for applications that need to support multiple connections in real time, such as chat applications or video games. Likewise, its use is also very common in the creation of microservices; that is, when a large application is divided into several smaller applications, each one taking care of a single task or functional area.
What is npm
Npm is a JavaScript package manager used to download applications or packages created by any developer. You can add, update or remove packages, among many other things. You can even create an npm account and upload your own packages.
With regard to package installation, it is important to note one consideration, and that is that you can install packages either locally or globally. Let's take a look at what both types of installation consist of.
When you install applications on your computer, you install them globally.
That is, you install the application once and it is immediately available for use by both the user and other applications that interact with it.
When you install packages using npm, you may install some packages globally, but most of the time you will install them locally to the project you are working on. Installing packages locally means that the package will only be available for the project you install it in. This way you can install different versions of the same package, depending on the requirements of each project. Locally installed packages are included in a project directory. It is important to keep this concept in mind, as we will see later on how to install them both ways.
How to Install Node.js and npm on Windows
Installing Node.js and npm on Windows is very easy. Just follow these steps:
1) Go to the node download page and download the node installer for Windows. Choose the version for your operating system, which is likely to be Windows 64-bit, unless your computer is very old.
2) Run the installer and click Next to continue with the installation. Then simply follow the steps on the screen, selecting the default options. When you are asked if you want to install chocolatey, it is recommended that you install it, although this is optional.
3) If you have accepted the installation of chocolatey during the installation, a powershell or command prompt window will open when the installation of Node.js and npm is finished. You must press ENTER to start the installation of the required components.
4) When the installation is complete, open a terminal or command prompt window and run the node -v and npm -v commands to verify the installation of Node and npm. In both cases a version number should be displayed:
$ node -v v12.16.3 $ npm -v 6.14.4
And with that, the installation of Node and npm on Windows is complete. If you have problems during the installation, please refer to the Node installation tutorial on Windows novice edition.
How to Install Node.js and npm on Mac
To install Node.js and npm on Mac, you will need to open a terminal window and follow the steps below:
1) For the installation of Node.js and npm we will use nvm (Node Version Manager). Enter the following command to install nvm:
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.35.3/install.sh | bash
2) Next, verify the nvm installation using the following command, which should display the nvm version number:
command -v nvm
3) It is recommended that you perform an additional check. Open the ~/.bash_profile
file and make sure that the line source ~/.bashrc
is written anywhere in the file. Then restart the terminal.
4) Now let's install Node using the following command:
nvm install node
5) When the installation is complete, run the following command to make nvm use the installed version of Node:
nvm use node
A confirmation message like the following should be displayed, indicating that nvm is using the version of Node you have installed (the versions of Node.js and npm are likely to be different):
Now using node v12.16.3 (npm v6.14.4)
6) Finally, run the node -v
and npm -v
commands to verify that both Node.js and npm have been installed correctly:
$ node -v v12.16.3 $ npm -v 6.14.4
How to Install Node.js and npm on Linux
To install Node.js and npm on Linux in a generic way (in another post I will explain how to install it on Ubuntu), you will need to open a terminal window and follow the steps below:
1) For the installation of Node.js and npm we will use nvm (Node Version Manager). To install nvm enter the following command:
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.35.3/install.sh | bash
2) Now verify the nvm installation by using the following command to display the nvm version number:
command -v nvm
3) It wouldn't be bad if you do an additional check to avoid problems. Open the ~/.bash_profile
file with any text editor and check that the line source ~/.bashrc
is written somewhere in the file. Then restart the terminal.
4) Now let's install Node using the following command:
nvm install node
5) When the installation is completed, run the following command to make nvm use the installed version of Node:
nvm use node
You should see a confirmation message like the following, indicating that nvm is using the version of Node you have installed (the versions of Node.js and npm are likely to be different):
Now using node v12.16.3 (npm v6.14.4)
6) Finally, run the node -v
and npm -v
commands to verify that both Node.js and npm have been installed correctly:
$ node -v v12.16.3 $ npm -v 6.14.4
And with that, the installation of Node and npm on Linux is complete.
How to create a project
At this point, you will already be able to use a lot of development tools that require Node, such as Webpack or Gulp. However, what we are going to do next is to create our first project. It will be a very simple project, but you will learn how to install dependencies and how to run it.
Initialise the project
The first thing we are going to do is initialise a new project. To do this, create a directory where you want the project to be located. In my case, I usually create node projects in the /code folder. In this example we will call the project "hello-node", so in my case, I will access the /code directory and then create the hello-node directory:
mkdir hello-node
Then, you must access the newly created directory:
cd hello-node
Then initialise the project using the following command:
npm init
The above command will launch an application that will guide you through the creation of the project:
1) First you will need to enter the name of the package you are creating. By default, the directory name is used, so press ENTER to set the next one, unless you prefer another one:
hello-node
2) You will then need to enter the version number of the package, which defaults to the following version:
1.0.0
3) Then you have to enter the description of the package:
Simple application that displays "Hello Node" on the screen.
4) Next we will have to enter the main file of the application, which by default is index.js, so press ENTER to continue.
5) Then press ENTER to accept the rest of the options with their default values, except for the author field, where you can enter your name.
6) Then press the ENTER key in the following sections until the creation of the project is complete.
As you can see, there is now a new file in the root folder of the project called package.json. If you open it, you will see that it contains the information you have entered:
{ "name": "hello-node", "version": "1.0.0", "description": "Simple application that displays "Hello Node" on the screen.", "main": "index.js", "scripts": { "test": "echo "Error: no test specified" && exit 1" }, "author": "Salvietta", "license": "myLicense" }
The package.json file contains information about the project, as well as the project's dependencies. The dependencies are the Node packages you use in the project. At the moment you don't have any yet.
Installing dependencies
Once the project is created, let's try to install some dependencies with npm. The command used to install dependencies is the npm install
command. We are going to try to install the terminal-kit package, which is a famous package that adds a set of functions that allow us to apply styles to the command line, among other things. To install terminal-kit we will have to use the command npm install
followed by the package name:
npm install terminal-kit
You will notice there is a new directory called node_modules, which is where the project's packages are installed. If you look inside it, you will see that it now contains a directory called terminal-kit
, which is the one we have added.
The package,json file will also have been modified, which will now contain the package you just installed as an element of the "dependencies" option:
"dependencies": { "terminal-kit": "^1.35.2" },
Now you will be able to run this package in your project. It will also create the package-lock.json file, which is used by npm internally, so you don't have to worry about it.
In the hypothetical case that you would like to install the terminal-kit package globally and not only for this project, you would have to use the -g flag together with the npm install command, like this:
npm install -g terminal-kit
The process is exactly the same for installing any other package. In case the package you are going to install needs other packages, they will be installed automatically. If you want to search for any other package, you can try searching for it on the npm website.
In case you want to install some dependency but you want it to be saved only in your local development environment and not in production, you should use the --save-dev or -D flags together with the npm install command. It is not necessary to run this command in this tutorial, since we will not use any framework for testing, but in case we do, we could install the mocha framework with the following command:
npm install mocha -D
By adding the
--save-dev
or -D
flags, the packages will only be installed in your local development version. In fact, if you have installed mocha and you edit the packages.json file, you will see that the mocha package has been added as an item in the "devDependencies"option and not in "dependencies":
"devDependencies": { "mocha": "^7.2.0" }
In case you wanted to uninstall a package, you would use the command npm uninstall, followed by the package name. For example, let's uninstall mocha:
npm uninstall mocha
The project code
What we are going to do is very simple. We are going to create the main access point to the project, which as we have specified during the initialization of the project, is the index.js file, which we will create from the terminal using the touch command or, even better, perhaps at this point it would be more appropriate to open the project folder with an editor such as VS Code, Sublime or Netbeans.
It is not an order, it is just a recommendation, young padawan.
Well, once the index.js file is created, edit it and add the following function at the top of it:
const term = require( 'terminal-kit' ).terminal;
What we have done is to assign terminal-kit to the variable "term", from where we will be able to access all its functions. The require()
function is used to include external packages. Since JavaScript ES6 also includes the import()
function, which is becoming more and more widespread.
To print text to the console, we would normally use the console.log()
function. However, what we are going to do is to display the text in magenta color using the term.magenta()
function.
Here is the complete code from the index.php file:
// We require terminal-kit package const term = require( 'terminal-kit' ).terminal; // Assign the text to a variable const text = "Hello from Node!"; // Display the text term.magenta(text) ;
Start the project
To start the project we have created, you must use the node command in the command terminal, followed by the name of the file you want to start, which in this case is the index.js file:
node index.js
If everything went well, you should be able to see the text "Hello from Node!" in the terminal with the color we have indicated.
Your next step
In this tutorial you have learned how to install Node.js and npm on Windows, Mac and Linux. With this you will now be able to make use of a wide range of development tools such as Gulp, Grunt or Webpack. You also know how to start a project and how to run a simple application.
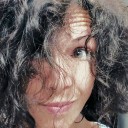
Silvia Mazzetta
Web Developer, Blogger, Creative Thinker, Social media enthusiast, Italian expat in Spain, mom of little 9 years old geek, founder of @manoweb. A strong conceptual and creative thinker who has a keen interest in all things relate to the Internet. A technically savvy web developer, who has multiple years of website design expertise behind her. She turns conceptual ideas into highly creative visual digital products.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…