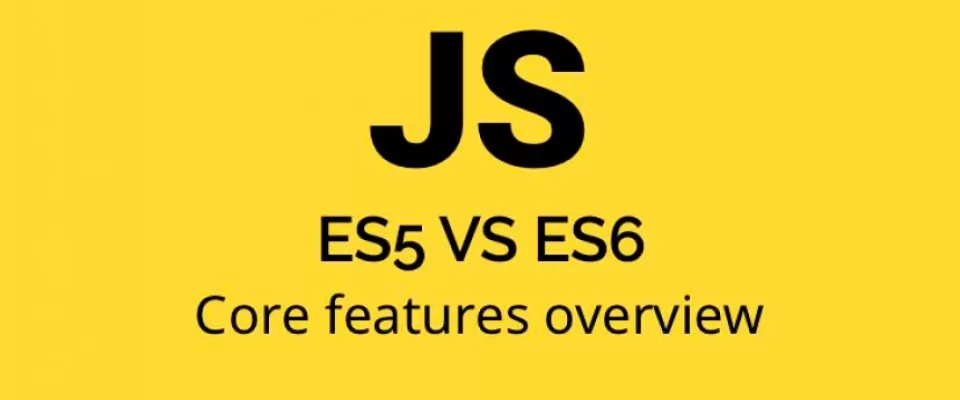
Several changes to JavaScript were introduced by ECMAScript 2015, also well-known as ES6. Here is a summary of a number of the foremost common options and syntactic variations, where applicable, with comparisons to ES5.
Variables and constant comparison
In the following table, we provide a brief overview of the concepts of scope and the differences between let, var, and const.
Keyword | Scope | Hoisting | Can Be Reassigned | Can Be Redeclared |
---|---|---|---|---|
var | Function scope | Yes | Yes | Yes |
let | Block scope | No | Yes | No |
const | Block scope | No | No | No |
Variable declaration
"Let" allows us to define variables. Originally we used the keyword "var" to declare our variables. However, when it comes to scope, it has some limitations because it does not offer a block scope. But now we can simply declare real block-scoped variables with the "let" keyword. This is a difference from the var keyword, which defines a variable globally or locally for a whole function, regardless of the block scope.
ES5
var x = 0;
ES6
let x = 0;
Constant declaration
ES6 has introduced the const keyword, which cannot be redeclared or reassigned, but isn't immutable.
We will use the "const" keyword to generate and initialize a read-only variable that has a constant value and something that we can never change. In ES6, "const" has block scoping like the "let" keyword. The value of a constant can not change through reassignment, and cannot be redeclared. A constant can not share its name with a function or variable in the same scope. Constants are uppercases by convention.
Here's the MDN definition for const:
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned.
ES6
const CONST_IDENTIFIER = 0;
Arrow functions
Arrow functions were also implemented with ES6 as a new syntax for JavaScript functions. They save developers time and optimize the scope of functions.
Arrow functions, also commonly known as "fat arrow" functions, are a more clear and concise for writing function expressions. They just use a new token, = >, which looks like a fat arrow. Arrow functions are anonymous and change the way they bind to functions.
They make the code more clear and simplify function scoping.
// (param1, param2, paramN) => expression
ES5
var multiplyES5 = function(x, y) { return x * y; };
ES6
const multiplyES6 = (x, y) => { return x * y };
Template literals
Concatenation/string interpolation
We can embed expressions in template literal strings.
ES5
var str = 'b-day date: ' + date;
ES6
let str = `b-day date: ${date}`;
Multi-line strings
To implement template literal syntax, we can span multiple lines without the need for concatenation. Here's an example of HTML content with interpolated data written in ES5 first and ES6 second:
var joe = { profile_image: 'http://namesite.com/200/200', name: 'Joe', title: 'Dance, Work, Code' } function get_user_widget_markup (user) { return [ '<div class="user-profile">', '<img src="' + user.profile_image + '" alt="" class="user-image">', '<h2 class="user-name">', user.name, '</h2>', '<p class="user-title">', user.title, '</p>', '</div>' ].join('') } get_user_widget_markup(Joe)
How about ES6?
let joe = { profile_image: 'http://namesite.com/200/200', name: 'Joe', title: 'Dance, Work, Code' } function get_user_widget_markup (user) { return `<div class="user-profile"> <img src="${user.profile_image}" alt="" class="user-image"> <h2 class="user-name">${user.name}</h2> <p class="user-title">${user.title}</p> </div>` } get_user_widget_markup(Joe)
Implicit returns
In ES6 the return keyword can be omitted if we use arrow functions without a block body.
ES5
function func(a, b, c) { return a + b + c; }
ES6
let func = (a, b, c) => a + b + c;
Key/property shorthand
Unlike ES5, if you want to define an object whose keys have the same name as the variables passed-in as properties, you can use the shorthand and simply pass the key name.
ES5
var cat = 'Miaow'; var dog = 'Woof'; var bird = 'Peet peet'; var someObject = { cat: cat, dog: dog, bird: bird }
ES6
let cat = 'Miaow'; let dog = 'Woof'; let bird = 'Peet peet'; let someObject = { cat, dog, bird } console.log(someObject); //{ // cat: "Miaow", // dog: "Woof", // bird: "Peet peet" //}
Method definition shorthand
Object methods in ES5 require the function statement. For example:
// ES5 code var lib = { sum: function(a, b) { return a + b; }, mult: function(a, b) { return a * b; } }; console.log( lib.sum(2, 3) ); // 5 console.log( lib.mult(2, 3) ); // 6
This isn't necessary in ES6; it allows the following shorthand syntax:
// ES6 code const lib = { sum(a, b) { return a + b; }, mult(a, b) { return a * b; } }; console.log( lib.sum(2, 3) ); // 5 console.log( lib.mult(2, 3) ); // 6
We can't use ES6 fat arrow => function syntax here, because the method requires a name. That said, we can use arrow functions if we name each method directly (like ES5). For example:
// ES6 code const lib = { sum: (a, b) => a + b, mult: (a, b) => a * b }; console.log( lib.sum(2, 3) ); // 5 console.log( lib.mult(2, 3) ); // 6
Destructuring (object matching)
We have to use curly brackets to assign properties of an object to their own variable, such as:
var obj = { a: 1, b: 2, c: 3 };
ES5
var a = obj.a; var b = obj.b; var c = obj.c;
ES6
let {a, b, c} = obj;
Array iteration (looping)
ES6 introduced a simple syntax for iteration through arrays and other iterable objects.
var arr = ['a', 'b', 'c'];
ES5
for (var i = 0; i < arr.length; i++) { console.log(arr[i]); }
ES6
for (let i of arr) { console.log(i); }
Default parameters
Default parameters allow us to initialize functions with default values. A default value is used when an argument is either omitted or undefined. A default parameter can be anything from a number to another function.
In ES5, you may have used a pattern like this one:
function getInfo (user, year, color) { year = (typeof year !== 'undefined') ? year : 2018; color = (typeof color !== 'undefined') ? color : 'Blue'; // remainder of the function... }
In this case, the getInfo() function has only one mandatory parameter: name. Year and color parameters are optional, so if they’re not provided as arguments when getInfo() is called, they’ll be assigned default values.
Default parameter values can be defined in ES6 and beyond as follows:
function getInfo (name, year = 2018, color = 'blue') { // function body here... }
It’s really simple.
If the year and color values are passed to the function call, the values passed as arguments will replace those defined as parameters in the function definition. This works exactly as with ES5 models, but without all that extra code. Much easier to maintain and much easier to read.
Spread syntax
We can use Spread syntax to expand an array.
ES6
let arr1 = [1, 2, 3]; let arr2 = ['a', 'b', 'c']; let arr3 = [...arr1, ...arr2]; console.log(arr3); // [1, 2, 3, "a", "b", "c"]
or for function arguments.
let arr1 = [1, 2, 3]; let func = (a, b, c) => a + b + c; console.log(func(...arr1)); // 6
Classes and constructor functions
ES6 establishes the class
syntax on top of the prototype-based constructor function.
ES5
function Func(a, b) { this.a = a; this.b = b; } Func.prototype.getSum = function() { return this.a + this.b; } var x = new Func(3, 4);
ES6
class Func { constructor(a, b) { this.a = a; this.b = b; } getSum() { return this.a + this.b; } } let x = new Func(3, 4); x.getSum(); // returns 7
Inheritance
After we have classes, we also want an inheritance. Once again, it is possible to simulate inheritance in ES5, but it was quite complex to do so.
ES5
function Computer (name, type) { this.name = name; this.type = type; }; Computer.prototype.getName = function getName () { return this.name; }; Computer.prototype.getType = function getType () { return this.type; }; function Laptop (name) { Vehicle.call(this, name, ‘laptop’); } Laptop.prototype = Object.create(Computer.prototype); Laptop.prototype.constructor = Laptop; Laptop.parent = Computer.prototype; Laptop.prototype.getName = function () { return 'It is a laptop: '+ this.name; }; var laptop = new Laptop('laptop name'); console.log(laptop.getName()); // It is a laptop: laptop name console.log(laptop.getType()); // laptop
ES6
class Computer { constructor (name, type) { this.name = name; this.type = type; } getName () { return this.name; } getType () { return this.type; } } class Laptop extends Computer { constructor (name) { super(name, 'laptop'); } getName () { return 'It is a laptop: ' + super.getName(); } } let laptop = new Laptop('laptop name'); console.log(laptop.getName()); // It is a laptop: laptop name console.log(laptop.getType()); // laptop
We see how easy is to implement inheritance with ES6.
Modules – export/import
We can create modules to export and import code between files, example:
Our index.html
<script src="export.js"> </script> <script type="module" src="import.js"> </script>
Our .js first file, export.js:
let func = a => a + a; let obj = {}; let x = 0; export { func, obj, x };
Our .js second file, import.js:
import { func, obj, x } from './export.js'; console.log(func(3), obj, x);
Promises/Callbacks
Promises are among the most interesting news to JavaScript ES6. Along with many other things, JavaScript uses callbacks as an alternative to chaining functions to support asynchronous programming.
ES5 Callback
function doSecond() { console.log('Do second.'); } function doFirst(callback) { setTimeout(function() { console.log('Do first.'); callback(); }, 500); } doFirst(doSecond);
ES6 Promise
let doSecond = () => { console.log('Do second.'); } let doFirst = new Promise((resolve, reject) => { setTimeout(() => { console.log('Do first.'); resolve(); }, 500); }); doFirst.then(doSecond);
In the following example, we use an XMLHttpRequest ES6
function makeRequest(method, url) { return new Promise((resolve, reject) => { let request = new XMLHttpRequest(); request.open(method, url); request.onload = resolve; request.onerror = reject; request.send(); }); } makeRequest('GET', 'https://url.json') .then(event => { console.log(event.target.response); }) .catch(err => { throw new Error(err); });
SOURCES:
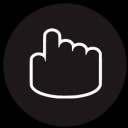
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…