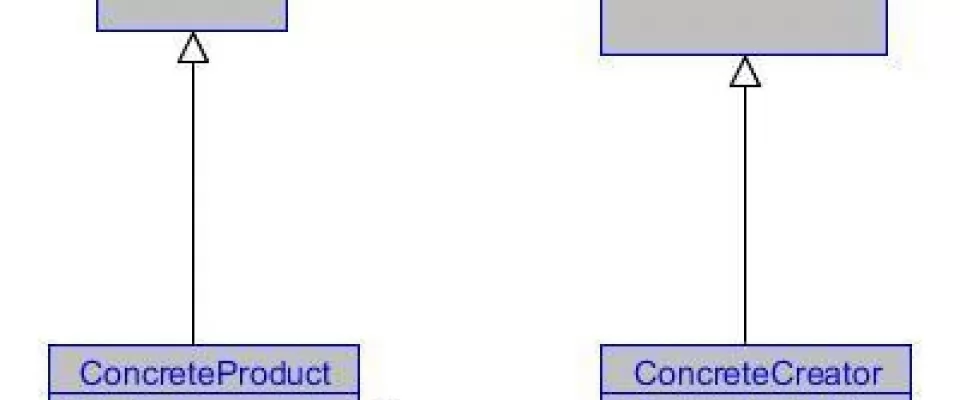
Going on with the speach about design patterns started previously, we are going to talk about another pattern often used: the Factory Method Pattern.
The GoF (Gang of Four Design Patterns) defines it in this way:
It defines an interface to create objects, but leaves to the subclasses the decision about the type of the class to instantiate.
Starting from the definition, we can see that the problem regarding the inheritance among classes discussed for the Singleton Pattern is no longer present. In fact, we decide to use the Factory Method Pattern when we are not able to know the exact type of object to be created beforehand or when we want to delegate the task of creating objects to other entities
In the majority of the books about this topic, the pattern is represented using an UML diagram like the one below:
Foundamental patterns's entities
- Creator: it has the duty of declaring the Factory that will take care of returning the correct object;
- ConcreteCreator: it makes the override of the Factory's method in order to return the correct implementation of the object;
- Product: it defines the interface of the object that Factory has to return;
- ConcreteProduct:it implements the object basing on Product;
The first observation, even if trivial, is that the implementation is definitely more complicated than the almost elementary one of the Singleton.
Some examples
Starting from an elementary example, let's define a CatFactory:
//Product interface Cat{ public void speak (); }
Every returned element from the factory has to implement this interface.
Let's create the concrete classes:
//ConcreteProduct class Abissinian implements Cat{ public void speak(){ System.out.println("Abissinian"); } } class AmericanCurl implements Cat{ public void speak(){ System.out.println("American Curl"); } } class Asian implements Cat{ public void speak(){ System.out.println("Asian"); } }
I decided to implement every class as non-public, assuming its placement in the same file. In fact, as we know, Java prohibits more than one public class in the same file, since the file must have the same name as the public class.
The foundamental concept of this pattern is the fact that every concrete class is a derivative of a basic type. In this case, every class implements Cat.
The Factory Class
The element to which the creation of objects is entrusted is precisely the so-called Factory class, which will have a static method with this functionality inside.
Let's see how this class could be implemented:
public class Factory{ public static Cat generateCat(String criteria){ if(criteria.equals("Abissino")) return new Abissino(); if(criteria.equals("Curl")) return new AmericanCurl(); if(criteria.equals("Asian")) return new Asian(); return null; } }
We can notice that the code of the Factory class is quite easy, because it accepts only three strings. In a hypothetical real situation, the code would be longher and more complex, but this one is sufficient to give an idea of how the pattern works.
The Factory Method has different advantages and disadvantages, such as:
- it represents a link to the subclasses: through the creator it is possible to dynamically choose which concrete class to use without any impact on the use of the end user;
- it links class hierarchies in parallel: the ConcreteCreator can connect to the ConcreteProduct and generate a parallel link between different hierarchies;
Why use the Factory Method Pattern?
As I told before, there are different situations where we can't know before what the concrete type of the objects that will be instanciated. But the problem is much more bigger.
We could run into cases where we know the exact type of objects, but the latter may change in the future. So the client is free from the burden of knowing what kind of objects has to instanciate and the pattern returns an abstract object which is then realized through the classes inherited from the abstract entity. The client often guides the creation of the object, as in the example shown above, but ignores the details of its construction.
The Iterator pattern
It could sound strange, but the Iterator pattern can be assimilated to the Factory Method family. In fact Iterator allows us to access sequentially to the elements of a list, relieving the caller of the need to know what the instantiated classes are.
Iterator is an interface that exposes three methods:
- boolean hasNext() that returns true if another iteration is possible;
- Object next() which returns the item following the current one;
- void remove() that removes the current element from the list;
Let's look at an example:
import java.io.*; import java.util.*; class Test { public static void main(String[] args) { ArrayListlist = new ArrayList (); list.add("1"); list.add("2"); list.add("3"); list.add("4"); list.add("5"); // Iterator to scan the list Iterator iterator = list.iterator(); System.out.println("List elements : "); while (iterator.hasNext()) System.out.print(iterator.next() + " "); System.out.println(); } }
We will obtain as output:
List elements : A B C D E
Java makes available a class called ListIterator that allows to scan objects collections in both directions, from the head to the tail and from the tail to the head.
Summing up
We use the Factory Method Pattern when:
- A class can not know in advance the exact type of the object to create;
- The class knows the exact type of objects, but it needs to delegate to another extern entity their creation;
Participants:
- Product: is the interface of the object created by the Factory Method;
- ConcreteProduct: implements Product;
- Creator: declares the Factory method and returns a Product object;
- ConcreteCreator: specifies the Factory Method and returns the correct instance;
So:
- The code has a higher flexibility level;
- A wrong usage can lead to the generation of too much classes;
Focusing on some implementation details:
- Creator can be concrete or abstract, and it can create a default version of the Factory Method;
- A Factory can build objects of different types using if-then-else constructs;
Typically the Factory Method Pattern is used to create Logger and other softwares of this kind.
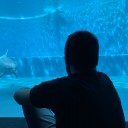
Alessio Mungelli
Computer Science student at UniTo (University of Turin), Network specializtion, blogger and writer. I am a kind of expert in Java desktop developement with interests in AI and web developement. Unix lover (but not Windows hater). I am interested in Linux scripting. I am very inquisitive and I love learning new stuffs.
Related Posts
Alternative tools for graphic design
There are many people today who only use the following for design purposes Canva as it is a really popular software and website and there is no denying that it…
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Hidden Gmail codes to find a lost e-mail
If you have a lot of emails in Gmail, there are a few codes that will help you find what you need faster and more accurately than if you do…
How to download an email in PDF format in Gmail for Android
You will see how easy it is to save an email you have received or sent yourself from Gmail in PDF format, all with your Android smartphone. Here's how it's…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
