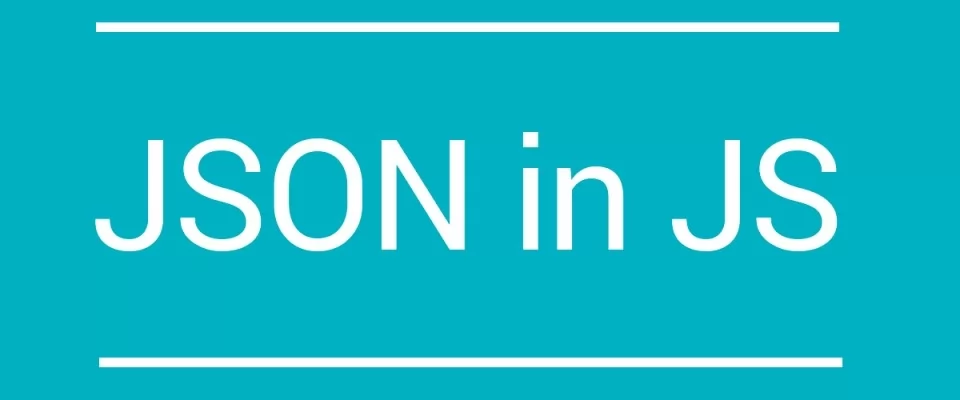
JSON is short for JavaScript Object Notation and an easy - to - access way to store information. In short, it gives us a collection of human - readable data that we can access in a very logical way.
In addition to RSS feeds, any sites now share data using JSON, and for good reason.
JSON feeds can be loaded asynchronously much more easily than XML / RSS.
Although it’s derived from JavaScript it may be used with many programming languages. In this article however, we’ll be focusing on the use of JSON in JavaScript.
How do we use JSON in a project?
As described above, a JSON is a string whose format very much resembles JavaScript object literal format.
You can include the same basic data types inside JSON as you can in a standard JavaScript object — strings, numbers, arrays, booleans, and other object literals.Where do we use JSON?
- Data storage
- Data configuration and verification
- Generating data structures from user input
- Data transfer from client to server, server to client, and between servers
JSON is also used with AJAX, asynchronous JavaScript and XML. AJAX is often used to transfer data without refreshing the browser page. Later in this article, we'll see how to make an AJAX request for a JSON file and show the content in the browser.
JSON Syntax & Structure
JSON syntax is derived from JavaScript object notation syntax:
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
JSON data is written as name/value pairs.
A name/value pair consists of a field name (in double quotes), followed by a colon, followed by a value:
{ "key": "value" } { "name": "Pluto" }
JSON names require double quotes. JavaScript names don't.
Data Types
The following data types can be used with JSON:
- strings (in double quotes)
- numbers
- objects (JSON object)
- arrays
- Booleans (true or false)
- null
In JavaScript values can be all of the above, plus any other valid JavaScript expression, including:
- a function
- a date
- undefined
In JSON, string values must be written with double quotes:
Use cases
As a simple example, information about me might be written in JSON as follows:
{ "name":"Paul", "age":30, "address":{ "street":"1 Main St", "city": "London" }, "interests":["design", "sports"] }
Here we have a name set as a string, age as a number, the address is an embedded object and the interests are an array of strings.
If you work with JSON, you may also find JSON as an object or string in a program context. If your JSON object is in a file.js or.html, the variable will probably be set. Our example would then look like:
let contacts = { name:"Paul", age:30, address:{ street:"1 Main St", city: "London" }, interests:["design", "design"] }
Or if stored as a string, it's going to be a single line::
let contacts = { "name":"Paul", "age":30, "address":{ "street":"1 Main St", "city": "London" }, "interests":["deesign", "design"]}
Naturally writing on multiple lines in the JSON format makes it much more readable, especially when dealing with a lot of data. JSON ignores the whitespace between its elements, so you can space your key - value pairs to facilitate the eye data!
JSON & JavaScript
Let's do an example. Create an HTML file as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>JSON Demo</title> </head> <body> <script> let contacts = { name: "Paul", age: 30, address: { street: "1 Main St", city: "London" }, interests: ["design", "cooking"] } </script> </body> </html>
We can access our JSON data through the console using dot notation, like so:
contacts.name // Paul contacts.age // 30 contacts.address.street // 1 Main St contacts.address.city // London contacts.interests[0] // design contacts.interests[1] // cooking
While developing a JavaScript application, you will sometimes want to serialize your data into a plain string. This can be useful for things like:
- Storing object data in a database
- Outputting object data to the console for debugging
- Sending object data over AJAX or to an API
The simple syntax for converting an object to a string is:
JSON.stringify()
Let’s return our previous example and pass our contacts object into the function.
let contacts = { name: "Paul", age: 30, address: { street: "1 Main St", city: "London" }, interests: ["design", "cooking"] }
let s = JSON.stringify(contacts);
We’ll see in the console, the JSON available as a string rather than an object.
{"name":"Paul","age":30,"address":{"street":"1 Main St","city":"London"},"interests":["design","cooking"]}
To do the opposite, we use the JSON.parse() function.
JSON.parse()
To convert a string back into a function we use JSON.parse() function.
let s = JSON.parse(contacts);
Make sure the text is written in JSON format, or else you will get a syntax error.
Looping through arrays
When working with JSON, it is often necessary to loop through object arrays. Let's create a fast array:
let users = [ { name: "Paul", age: 30 }, { name: "Sylvia", age: 38 }, { name: "Luis", age: 45 } ];
And now, letscreate a for loop, to iterate through our data:
for (var i = 0; i < users.length; i++) { console.log(users[i].name); }
------------ output:
Paul Sylvia Luis
Lets see revise our HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>JSON Demo</title> </head> <body> <ul id="users"></ul> <script> let contacts = { name: "Paul", age: 30, address: { street: "1 Main St", city: "London" }, interests: ["design", "cooking"] } // let s = JSON.stringify(contacts); // let s = JSON.parse(contacts); let users = [ { name: "Paul", age: 30 }, { name: "Sylvia", age: 38 }, { name: "Luis", age: 45 } ]; let output = ''; for ( var i = 0; i < users.length; i++) { // console.log(users[i].name); output += '<li>'+users[i].name+'</li>'; } document.getElementById('users').innerHTML = output; </script> </body> </html>
How to access JSON from a URL
We'll need to access JSON most of the time from a URL.
There is an additional step involved in this, we will use AJAX to make a GET application for the file.
Take the above JSON string for our demo and put it in a new file called users.json. It should be like:
[ { "name": "Paul", "age": 30 }, { "name": "Sylvia", "age": 38 }, { "name": "Luis", "age": 45 } ]
Now we’ll make an XMLHttpRequest().
let request = new XMLHttpRequest();
We'll open the file users.json via GET (URL) request.
request.open('GET', 'users.json', true);
Clearly, if your JSON file is stored externally, you'd replace users.json
with the full URL address, e.g. https / api.myjson.com/demo/sample.json
.
Now we parse our JSON data within the onload function. And then loop through the data, displaying in our ul element..
request.onload = function () { // Convert JSON data to an object let users = JSON.parse(this.response); let output = ''; for ( var i = 0; i < users.length; i++) { output += '<li>' + users[i].name + ' is ' + users[i].age + ' years old.'; '</li>' } document.getElementById('users').innerHTML = output; }
Finally, we submit the request!
request.send();
Here’s the final code.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>JSON Demo</title> </head> <body> <ul id="users"></ul> <script> let request = new XMLHttpRequest(); request.open('GET', 'users.json', true); request.onload = function () { // Convert JSON data to an object let users = JSON.parse(this.response); let output = ''; for ( var i = 0; i < users.length; i++) { output += '<li>' + users[i].name + ' is ' + users[i].age + ' years old.'; '</li>' } document.getElementById('users').innerHTML = output; } request.send(); </script> </body> </html>
We're nearly done!
But if you open the HTML file, you get an error from the console.
As we call users.json from the file system instead of a real domain (a security protocol for the browser).
So we'll have to run it on a local server to test it locally.
If you installed xampp, go ahead and upload it to your webroot. However, the installation of liveserver via npm would be a simpler solution. Make sure you have installed NodeJS and run npm install-g live server from the directory in which your HTML file is stored. After the installation of the module is complete, you simply run live servers from the same directory and the HTML file will open on a local server.
And our data will print as specified!
Paul is 30 years old. Sylvia is 38 years old. Luis is 45 years old.
The Fetch API
We all remember the dreaded XMLHttpRequest we used back in the day to make requests, it involved some really messy code, it didn't give us promises and let's just be honest, it wasn't pretty JavaScript, right? Maybe if you were using jQuery, you used the cleaner syntax with jQuery.ajax().
Well JavaScript has it's own built-in clean way now. Along comes the Fetch API a new standard to make server request jam-packed with promises and all those things we learned to love over the years.
We can use Fetch with our data like so:
fetch('./users.json').then( response => { return response.json(); }).then( data => { // Work with your JSON data here.. console.log(data); }).catch( err => { // What do when the request fails console.log('The request failed!'); });
Using JSON with Query
If you use jQuery on your project, the getJSON) (function can be used to retrieve data.
$(document).ready(function () { $.getJSON('users.json', function (data) { // Work with your JSON data here.. console.log(data[0].name); }); });
Don’t forget to add jQuery above this function, if you’re testing it out!
We hope this article has helped you to better understand JSON and to see it in action with AJAX! ;-)
Business vector created by freepik - www.freepik.com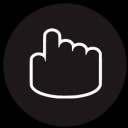
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…