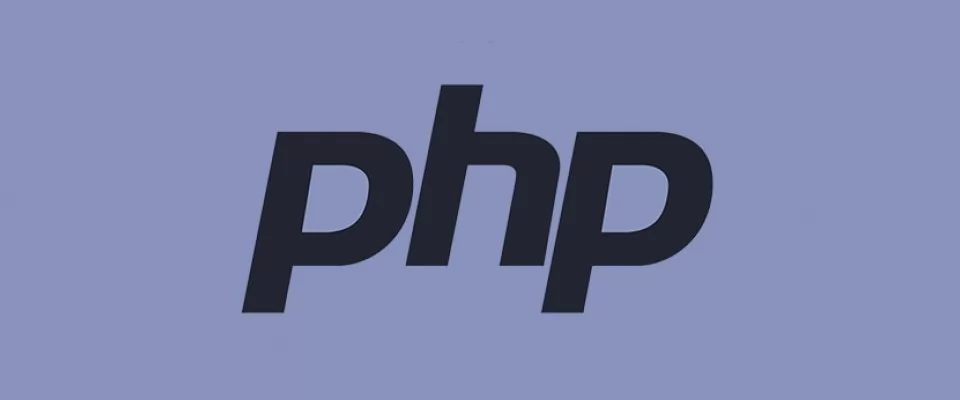
Mastering Repetition and Iteration for Efficient Programming
Loops are an essential part of any programming language, including PHP. They allow you to repeat a block of code multiple times, making it easier to handle repetitive tasks and iterate over data structures. In PHP 8, there are several types of loops available, including the for
, while
, do-while
, and foreach
loops. In this article, we'll explore each type and provide code examples to help you understand how they work.
1. The for
Loop
The for
loop is commonly used when you know the number of iterations in advance. It consists of three parts: initialization, condition, and increment/decrement.
Here's the syntax for a for
loop:
for (initialization; condition; increment/decrement) { // code to be executed }
Let's say we want to print the numbers from 1 to 5 using a for
loop:
for ($i = 1; $i <= 5; $i++) { echo $i . ' '; }
Output:
1 2 3 4 5
2. The while
Loop
The while
loop is used when you want to repeat a block of code as long as a certain condition is true. It checks the condition before each iteration.
Here's the syntax for a while
loop:
while (condition) { // code to be executed }
Let's print the even numbers from 2 to 10 using a while
loop:
$i = 2; while ($i <= 10) { echo $i . ' '; $i += 2; }
Output:
2 4 6 8 10
3. The do-while
Loop
The do-while
loop is similar to the while
loop, but it checks the condition after each iteration. This means that the loop body is executed at least once, even if the condition is initially false.
Here's the syntax for a do-while
loop:
do { // code to be executed } while (condition);
Let's print the numbers from 1 to 5 using a do-while
loop:
$i = 1; do { echo $i . ' '; $i++; } while ($i <= 5);
Output:
1 2 3 4 5
4. The foreach
Loop
The foreach
loop is specifically designed to iterate over arrays and objects. It automatically assigns the current element's value to a variable, which can be used within the loop body.
Here's the syntax for a foreach
loop:
foreach ($array as $value) { // code to be executed }
Let's iterate over an array and print its elements using a foreach
loop:
$fruits = ['apple', 'banana', 'cherry']; foreach ($fruits as $fruit) { echo $fruit . ' '; }
Output:
apple banana cherry
You can also access the key and value of each element using the following syntax:
foreach ($array as $key => $value) { // code to be executed }
Let's print the keys and values of an associative array:
$person = [ 'name' => 'John', 'age' => 30, 'city' => 'New York' ]; foreach ($person as $key => $value) { echo $key . ': ' . $value . ' '; }
Output:
name: John age: 30 city: New York
Loops are powerful constructs in PHP that allow you to repeat code and iterate over data structures. In this article, we covered the for, while, do-while, and foreach loops in PHP 8. By understanding these loop types and practicing with code examples, you'll be able to handle repetitive tasks more efficiently and work with arrays and objects effectively.
Remember to choose the appropriate loop type based on your specific requirements, such as knowing the number of iterations in advance (for loop), repeating until a condition is false (while loop), ensuring at least one execution of the loop body (do-while loop), or iterating over arrays and objects (foreach loop).
Happy coding with loops in PHP 8!
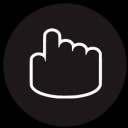
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Examine the 10 key PHP functions I use frequently
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating. 1. Levenshtein This function uses the Levenshtein algorithm to calculate the…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
PHP - The Singleton Pattern
The Singleton Pattern is one of the GoF (Gang of Four) Patterns. This particular pattern provides a method for limiting the number of instances of an object to just one.…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
The State of PHP 8: new features and changes
PHP 8.0 has been released last November 26: let's discover together the main innovations that the new version introduces in this language. PHP is one of the most popular programming languages…
HTTP Cookies: how they work and how to use them
Today we are going to write about the way to store data in a browser, why websites use cookies and how they work in detail. Continue reading to find out how…
The most popular Array Sorting Algorithms In PHP
There are many ways to sort an array in PHP, the easiest being to use the sort() function built into PHP. This sort function is quick but has it's limitations,…
MySQL 8.0 is now fully supported in PHP 7.4
MySQL and PHP is a love story that started long time ago. However the love story with MySQL 8.0 was a bit slower to start… but don’t worry it rules…
A roadmap to becoming a web developer in 2019
There are plenty of tutorials online, which won't cost you a cent. If you are sufficiently self-driven and interested, you have no difficulty training yourself. The point to learn coding…
10 PHP code snippets to work with dates
Here we have some set of Useful PHP Snippets, which are useful for PHP Developers. In this tutorial we'll show you the 10 PHP date snippets you can use on…
8 Free PHP Books to Read in Summer 2018
In this article, we've listed 8 free PHP books that can help you to learn new approaches to solving problems and keep your skill up to date. Practical PHP Testing This book…