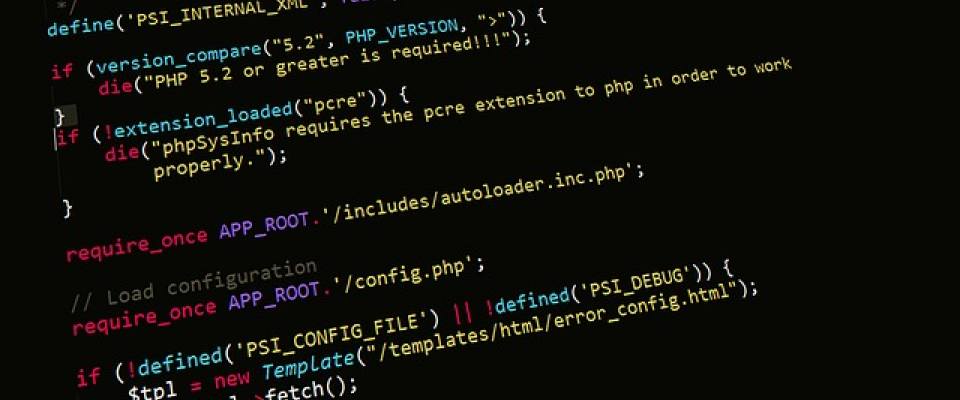
Here we have some set of Useful PHP Snippets, which are useful for PHP Developers. In this tutorial we'll show you the 10 PHP date snippets you can use on your webpages by just cutting and pasting!
Get current time, formatted
Super basic function, takes no parameters and returns the current date. This example uses British date formatting, you can change it on line 2.
function nowuk(){ return date('d/m/Y', time()); }
Format a date
The easiest way to convert a date from a format (here yyyy-mm-dd) to another. For more extensive conversions, you should have a look at the DateTime class to parse & format.
$originalDate = "2010-03-21"; $newDate = date("d-m-Y", strtotime($originalDate));
Source: Stack Overflow
Get week number from a date
When coding you often find yourself in the need of getting the week number of a particular date. Pass your date as a parameter to this nifty function, and it will return you the week number.
function weeknumber($ddate){ $date = new DateTime($ddate); return $date->format("W"); }
Convert minutes to hours and minutes
Here is a super useful function for displaying times: Give it minutes as an integer (let’s say 135) and the function will return 02:15. Handy!
function convertToHoursMins($time, $format = '%02d:%02d') { if ($time < 1) { return; } $hours = floor($time / 60); $minutes = ($time % 60); return sprintf($format, $hours, $minutes); }
Get difference between two times
This function takes two dates and returns the interval between those two. The result is set to be displayed in hours and minutes, you can easily change it on line 5 to fit your needs.
function dateDiff($date1, $date2){ $datetime1 = new DateTime($date1); $datetime2 = new DateTime($date2); $interval = $datetime1->diff($datetime2); return $interval->format('%H:%I'); }
Check if a date is in the past or in the future
Very simple conditional statements to check if a date is past, present, or future.
if(strtotime(dateString) > time()) { # date is in the future } if(strtotime(dateString) < time()) { # date is in the past } if(strtotime(dateString) == time()) { # date is right now }
Source: Art of Web
Calculate age
This very handy function takes a date as a parameter, and returns the age. Very useful on websites where you need to check that a person is over a certain age to create an account.
function age($date){ $time = strtotime($date); if($time === false){ return ''; } $year_diff = ''; $date = date('Y-m-d', $time); list($year,$month,$day) = explode('-',$date); $year_diff = date('Y') - $year; $month_diff = date('m') - $month; $day_diff = date('d') - $day; if ($day_diff < 0 || $month_diff < 0) $year_diff-; return $year_diff; }
Source: AP PHP
Show a list of days between two dates
An interesting example on how to display a list of dates between two dates, using DateTime() and DatePeriod() classes.
// Mandatory to set the default timezone to work with DateTime functions date_default_timezone_set('America/Sao_Paulo'); $start_date = new DateTime('2010-10-01'); $end_date = new DateTime('2010-10-05'); $period = new DatePeriod( $start_date, // 1st PARAM: start date new DateInterval('P1D'), // 2nd PARAM: interval (1 day interval in this case) $end_date, // 3rd PARAM: end date DatePeriod::EXCLUDE_START_DATE // 4th PARAM (optional): self-explanatory ); foreach($period as $date) { echo $date->format('Y-m-d').'<br/>'; // Display the dates in yyyy-mm-dd format }
Source: Snipplr
Twitter Style “Time Ago” Dates
Now a classic, this function turns a date into a nice "1 hour ago" or "2 days ago", like many social media sites do.
function _ago($tm,$rcs = 0) { $cur_tm = time(); $dif = $cur_tm-$tm; $pds = array('second','minute','hour','day','week','month','year','decade'); $lngh = array(1,60,3600,86400,604800,2630880,31570560,315705600); for($v = sizeof($lngh)-1; ($v >= 0)&&(($no = $dif/$lngh[$v])<=1); $v--); if($v < 0) $v = 0; $_tm = $cur_tm-($dif%$lngh[$v]); $no = floor($no); if($no <> 1) $pds[$v] .='s'; $x=sprintf("%d %s ",$no,$pds[$v]); if(($rcs == 1)&&($v >= 1)&&(($cur_tm-$_tm) > 0)) $x .= time_ago($_tm); return $x; }
Source: CSS Tricks
Countdown to a date
A simple snippet that takes a date and tells how many days and hours are remaining until the aforementioned date.
$dt_end = new DateTime('December 3, 2016 2:00 PM'); $remain = $dt_end->diff(new DateTime()); echo $remain->d . ' days and ' . $remain->h . ' hours';
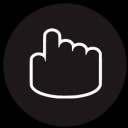
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Examine the 10 key PHP functions I use frequently
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating. 1. Levenshtein This function uses the Levenshtein algorithm to calculate the…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
PHP - The Singleton Pattern
The Singleton Pattern is one of the GoF (Gang of Four) Patterns. This particular pattern provides a method for limiting the number of instances of an object to just one.…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
The State of PHP 8: new features and changes
PHP 8.0 has been released last November 26: let's discover together the main innovations that the new version introduces in this language. PHP is one of the most popular programming languages…
HTTP Cookies: how they work and how to use them
Today we are going to write about the way to store data in a browser, why websites use cookies and how they work in detail. Continue reading to find out how…
The most popular Array Sorting Algorithms In PHP
There are many ways to sort an array in PHP, the easiest being to use the sort() function built into PHP. This sort function is quick but has it's limitations,…
MySQL 8.0 is now fully supported in PHP 7.4
MySQL and PHP is a love story that started long time ago. However the love story with MySQL 8.0 was a bit slower to start… but don’t worry it rules…
A roadmap to becoming a web developer in 2019
There are plenty of tutorials online, which won't cost you a cent. If you are sufficiently self-driven and interested, you have no difficulty training yourself. The point to learn coding…
8 Free PHP Books to Read in Summer 2018
In this article, we've listed 8 free PHP books that can help you to learn new approaches to solving problems and keep your skill up to date. Practical PHP Testing This book…
Best Websites to Learn Coding Online
You know and we know that it’s totally possible to learn to code for free... If you can teach yourself how to write code, you gain a competitive edge over your…