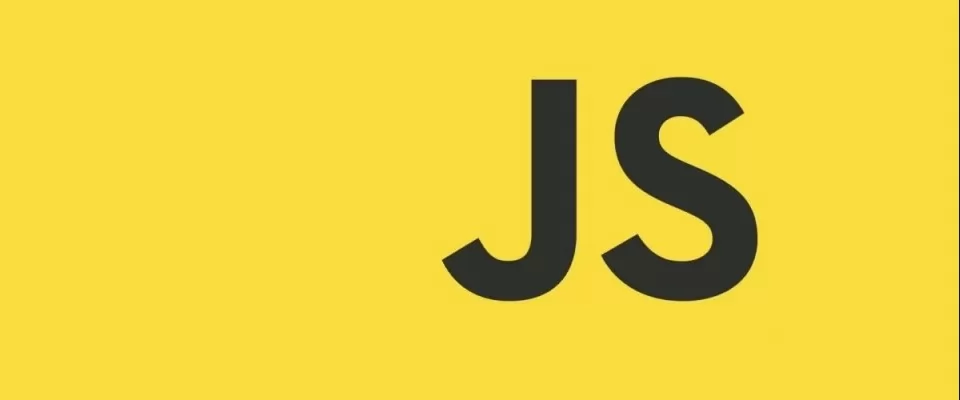
In this short tutorial we are going to look at the differences between primitive types and objects in JavaScript. To start with, we're going to look at what primitive types are. Then we will see what objects are and how they differ.
Introduction
The primitive types in JavaScript are as follows:
- Boolean: 'boolean
- String: 'string
- Number: 'number', 'bigint', 'bigint'.
- Symbol: 'symbol
- Undefined: 'undefined
As a general rule, anything that is not a primitive type in JavaScript is an object of type 'object'.
You are probably wondering why the type null
is not in the list. This is because null
is an object type.
To get the type of a variable in JavaScript you can use the typeof
operator. In fact, if you run the expression typeof null
you will see that the result you get is 'object'. This is one of the big differences between null
and undefined, as we have seen in the tutorial where we explained the differences between null
and undefined
.
As for functions, it is true that they are of type function, although we have not included this type in the list of primitive types. This is because the constructor of the function type is derived from the object type.
Differences between primitive types and objects
We will now look at the most notable differences between primitive types and objects:
- Primitive types are always passed by value, while objects are passed by reference.
- Primitive types are copied by value while objects are copied by reference.
- Primitive types are compared by value while objects are compared by reference.
- Primitive types are immutable, while the only immutable element of an object is its reference, and its value can be modified.
We will now look at some examples that validate these assertions.
To begin with, let's copy an object of primitive type to see what happens:
let animal = 'cat'; let pet = animal;
Now let's change the value of the animal variable and see what happens to the pet variable:
animal = 'dinosaur'; console.log(pet);
The value that will be shown on the screen will be 'cat', because when working with primitive types, the value assignment that we have done at the beginning has been done by value and not by reference. That is, although we can assign the value of one variable to another, they are totally independent.
Let's see now what happens when we copy an object:
let animal = { species: 'cat' } let pet = animal;
In this example, the pet variable points to the same object as the animal variable, since the assignment has been made by reference and not by value.
To demonstrate this, let's modify the species property:
animal.species= 'dinosaur'; console.log(pet.species);
The value that will be displayed on the screen will be 'dinosaur', since pet pointed to the same object as animal. In other words, we actually have a single object with two references to it. In fact, if we compare the animal object with the pet object we will see that the variables are identical:
if ( animal === pet) { console.log('We are the same object'); }
However, if we define two different objects, the result of the comparison will be false even if the objects are identical, as they will have a different reference:
let animal = { species: 'cat' } let pet = { species: 'dinosaurio' } if (animal !== pet) { console.log('We are not the same object'); }
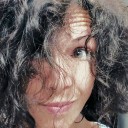
Silvia Mazzetta
Web Developer, Blogger, Creative Thinker, Social media enthusiast, Italian expat in Spain, mom of little 9 years old geek, founder of @manoweb. A strong conceptual and creative thinker who has a keen interest in all things relate to the Internet. A technically savvy web developer, who has multiple years of website design expertise behind her. She turns conceptual ideas into highly creative visual digital products.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…