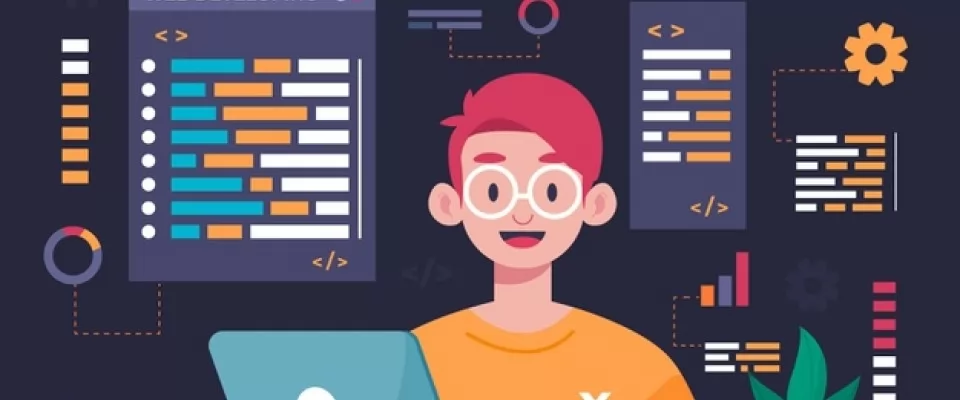
What are JavaScript frameworks?
JavaScript frameworks are application frameworks that allow developers to manipulate code to meet their particular needs.
Web application development is like building a house. You have the option of creating everything from scratch with construction materials. But it will take a long time and can incur high costs.
But if you use ready-made materials, such as bricks, and assemble them according to the architecture, construction is streamlined and saves money and time.
Application development works in a similar way. Instead of writing all the code from scratch, pre-written code can be used as building blocks based on the architecture of the application. Frameworks can be adapted to the website design more quickly and make it easier to work with JavaScript.
How to use JavaScript frameworks
To use a JavaScript framework, read the documentation for the JS framework you intend to use and follow the steps.
What are JavaScript frameworks for?
- To build websites
- Front-end application development
- Back-end application development
- Hybrid application development
- E-commerce applications
- Build modular scripts, e.g. Node.js
- Manually update the DOM
- Automate repetitive tasks using templates and bi-directional linking
- Develop video games
- Create image carousels
- Code testing and debugging
- To group modules
The most popular JavaScript frameworks
AngularJS
Google's AngularJS is an open source JavaScript framework released in 2010. It is a front-end JS framework that you can use to build web applications.
It was created to simplify the development and testing of web applications with a framework for MVC and MVVM client-side architectures.
Features/benefits:
- Supports two-way data binding.
- Use the directive to insert into HTML code and give the application better functionality
- Declare static documents quickly and easily.
- Its environment is readable, expressive and fast to develop.
- Impressive extensibility and customisability to work with
- Built-in testing capability and support for dependency injection.
Use cases:
- Develop e-commerce applications.
- Developing real-time data applications for weather updates.
- Example: YouTube PlayStation 3
Aurelia
Released in 2016, Aurelia is a simple, unobtrusive and powerful open source JS framework for building responsive mobile, desktop and browser applications.
It aims to focus on aligning web specifications with convention rather than configuration and requires fewer intrusions on the framework.
Features/benefits:
- Aurelia is designed to run high performance and perform batch DOM updates efficiently.
- It offers consistent and scalable performance even with a complex user interface.
- A comprehensive ecosystem with state management, validation and internationalisation.
- Enables reactive binding and synchronises your state automatically with high performance.
- Easier unit testing.
- Unprecedented extensibility to create custom elements, add attributes, manage template generation, etc.
- Take advantage of advanced client-side routing, UI composition and progressive enhancements.
Use cases:
- Develop applications.
- Use server-side rendering.
- Perform bi-directional data binding.
Vue.js
Vue.js was created in 2014 by Evan You while working for Google. It is a progressive JavaScript framework for building user interfaces.
Vue.js can be adopted incrementally from its core and can scale between a framework and a library easily based on various use cases.
Features/benefits:
- Supports ES5 compliant browsers.
- It has a core library that is accessible and focuses only on the view layer.
- It also supports other useful libraries that can help you manage the complexities associated with one-page applications.
- Lightning fast virtual DOM, 20 kb min+gzip runtime, and needs less optimization.
Use cases:
- Perfect for use in small projects that need less reactivity, show a modal, include a form using Ajax, etc.
- It can also be used in large single page applications using its Vuex and Router components.
- For creating events, linking classes, updating the content of elements, etc.
Ember.js
The open source JS framework Ember.js is a proven and productive framework for creating web applications with rich user interfaces, capable of running on all devices.
It was released in 2011 and was then called SproutCore 2.0.
Features/benefits:
- Scalable UI architecture.
- The "Stacks Included" perspective helps you find everything you need to start building your application right away.
- Features the Ember CLI functioning as the backbone of Ember applications and offering code generators to create new entities.
- Comes with a built-in development environment with fast auto-reloads, rebuilds and test runners.
- A first-class router that uses data loading with query parameters and URL segments.
- Ember Data is a data access library that works with multiple sources simultaneously and maintains model updates.
Use cases:
- For building modern interactive web applications.
- Used by DigitalOcean, Square, Accenture, etc.
Node.js
Node.js is an open source, server-side JavaScript framework built on top of Chrome's JS V8 engine, created in 2009. It is an execution environment that runs JS code outside of a browser.
Node.js is designed to help you develop scalable, fast and reliable web-based server-side applications.
Features/benefits:
Faster code execution.
You can control asynchronous I/O using its event-driven architecture.
Displays Java-like properties such as bundling, threading, looping.
Single-threaded model.
No buffering issues for video or audio, as processing time is significantly reduced.
Use cases:
For developing server-side applications.
Create real-time web applications.
Communication software.
Develop browser games.
Corporate usage includes GoDaddy, LinkedIn, Netflix, PayPal, AWS, IBM, and more.
Backbone.js
The lightweight JS framework Backbone.js was created in 2010 and is based on the Model View Presenter (MVP) architecture.
It has a RESTful JSON interface and helps you build client-side web applications. It structures web applications with models for custom events and key-value binding, collections with an efficient API and views using declarative event handling.
Features/benefits:
- Free and open source, with over 100 extensions available.
- Impressive design with less code.
- Offers structured and organised application development.
- Code is simple and easy to learn and maintain.
- Softer dependency on jQuery and stronger dependency on Underscore.js.
Use cases:
- Develop simple page applications.
- Seamless front-end JS functions.
- To create organised and well-defined mobile or client-side web applications.
Next.js
The Next.js open source platform provides a React front-end web development framework. Launched in 2016, it enables functionality such as static site creation and server-side rendering.
Features/benefits:
Automatic image optimisation using snapshot builds.
Built-in domain and subdomain routing and automatic language detection.
Real-time analytics scoring showing visitor data and per-page information.
Automatic aggregation and compilation.
Can pre-render a page at request time (SSR) or build time (SSG).
Supports TypeScript, file system routing, API paths, CSS, code splitting and grouping, and more.
Use cases:
This production-ready framework allows you to create both static and dynamic JAMstack sites.
Server-side rendering.
Mocha
Every application needs to be tested before being deployed. This is what Mocha or Mocha.js does for you.
It is a feature-rich open source JS testing framework that runs on Node.js as well as in a browser.
Features/benefits:
- Makes asynchronous testing fun and effortless.
- Allows Node.js tests to run concurrently.
- Automatically detects and turns off the colouring of a non-TTY stream.
- Reports test duration.
- Displays slow tests.
- Meta-generate test suites and test cases.
- Support for multiple browsers, configuration files, node debugger, source map, Growl, and more.
Use cases:
- To perform application audits.
- Execute functions in a given order using functions and record test results.
- Clean up the state of the tested software to ensure that each test case is executed separately.
Ionic
Launched in 2013, Ionic is an open source JavaScript framework for building high-quality hybrid mobile apps. Its latest version allows you to choose any UI framework such as Vue.js, React or Angular. It uses CSS, Sass and HTML5 to create apps.
Features/benefits:
- Leverages Cordova and Capacitor plugins to access OS features such as GPS, camera, torch, etc.
- Includes typography, mobile components, interactive paradigms, beautiful themes and custom components.
- Provides a CLI for object creation.
- Enables push notifications, creates app icons, native binaries and Splash screens.
Use cases:
- To build hybrid mobile apps.
- Build the front-end user interface framework.
- Create engaging interactions.
Webix
The easy-to-use Webix framework helps you develop rich UIs using lighter code. It offers 102 UI widgets such as DataTable, Tree, Spreadsheets, etc., along with feature-rich HTML5/CSS JS controls.
Features/benefits:
- Easy to use JS file management.
- Save time using built-in widgets and UI controls.
- Easy to understand code.
- Cross platform and cross browser compatibility.
- Seamless integration with other JavaScript libraries and frameworks.
- Fast performance for widget rendering, and even for large datasets such as Trees, Lists, etc.
- GDPR and HIPAA compliant along with unlimited extensibility and web accessibility.
Use cases:
- For developing UIs.
- Cross-platform web application development.
Gatsby
Gatsby helps you develop high performance websites and applications with React, a free and open source front-end JS framework. Check it out on GitHub.
Features/benefits:
- High performance with automatic code splitting, inlining styles, image optimisation, lazy-loading, etc. to optimise sites.
- Its serverless rendering creates HTML in the attic during build time. Therefore, no server and DDoS attacks or malicious requests.
- Increased web accessibility.
- More than 2000 plugins, themes and recipes.
Use cases:
- Development of front-end applications and websites.
- Generation of static sites.
- Server-side rendering.
- Used by sites such as Airbnb and Nike, the latter for its "Just Do It" project.
Meteor.js
Meteor is an open source JS framework released in 2012. It allows you to create complete applications for mobile, desktop and web.
Features/benefits:
- Integrate tools and frameworks for more functionality such as MongoDB, React, Cordova, etc.
- Build applications on any device.
- APM to see application performance.
- Live browser reloading.
- Open source isomorphic development ecosystem (IDevE) for easy development from scratch.
Use cases:
- Rapid prototyping.
- Cross-platform applications.
- Sites built with Meteor: Pathable, Maestro, Chatra, etc.
MithrilJS
Although not as popular as some of the other items on this list, Mithril is an advanced client-side JS framework for developing client-side applications. It is lightweight - less than 10kb gzip - but fast and offers XHR and routing utilities.
Features/benefits:
- Pure JS framework.
- Compatibility with all major browsers without polyfills.
- Creates Vnode data structures.
- Provides declarative APIs to handle user interface complexities.
Use cases:
- Single page applications.
- Used by sites like Vimeo, Nike, etc.
ExpressJS
Express.js is a back-end JS framework for web application development. It was released in 2010 under the MIT Incense as free and open source software.
It is a fast and minimalistic Node.js web framework that comes with a number of useful features.
Features/benefits:
- Scalable and lightweight.
- Enables reception of HTTP responses by allowing you to configure middleware.
- Features a routing table to perform actions based on the URL and HTTP method.
- Includes rendering of dynamic HTML pages.
Use cases:
- Rapid development of node-based applications.
- Creation of REST APIs.
Some useful JavaScript tools to know
Slick
Slick is a useful JS tool that takes care of your carousel needs. It is responsive and scalable with its container. Its features include CSS3 support, sliding, mouse dragging, full accessibility, infinite loop, autoplay, lazy loading, and many more.
Babel
Babel is a free and open source JS compiler that you can use to convert new JS features to run old standard JS. The plugin is also used for transforming syntax that is not compatible with an older version. It provides polyfills to support missing features in certain JS environments.
iziModal
iziModal is an elegant, lightweight, flexible and responsive modal plugin that works with jQuery. It's useful for notifying your users of something or requesting information using a modal popup. It is easy to use and comes with many customisations.
ESLint
Finding and fixing bugs in your JS code is easy using ESLint. It analyzes codes statistically to quickly detect syntax errors, command line style problems, etc., and fixes them automatically.
Shave
Shave is a zero dependency JS plugin that you can use to truncate text inside HTML elements by setting a maximum height to fit perfectly inside the element. It also stores some extra original text inside a hidden <span> element, ensuring that no text is lost.
Webpack
Webpack is a tool for packaging JS modules for modern applications. You can write the code and use it to package your assets in a reasonable way while keeping the code clean.
How JavaScript libraries and frameworks work
The difference between JavaScript libraries and frameworks lies in their flow of controls. They are simply opposite in flow, or reversed.
In JS libraries, the primary code calls the function provided by a library.
In JS frameworks, the framework itself calls the code and uses it in a specific way. It defines the overall design of the application.
In short, JavaScript libraries can be thought of as a particular function of the application. In contrast, the framework acts as its skeleton, while an API acts as the connector that binds them together.
Commonly, developers start the development process with a JS framework and then complete the application's functions with JS libraries and the help of an API.
JavaScript libraries and frameworks are effective in speeding up the development process of your website or application. And, as a web developer, using the right one for your project is crucial.
Different libraries and frameworks serve different purposes and have their own sets of pros and cons. Therefore, you should choose them based on your unique requirements and future goals associated with a website or application.
I hope this extensive list of JavaScript libraries and frameworks will help you choose the right one for your next project.
READ ALSO: Top Javascript Libraries and Frameworks Part 1
Table of contents
- What are JavaScript libraries?
- The most popular JavaScript libraries
- What are JavaScript frameworks?
- The most popular JavaScript frameworks
- Some useful JavaScript tools to know about
- How JavaScript libraries and frameworks work
Digital vector created by freepik - www.freepik.com
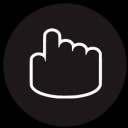
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…