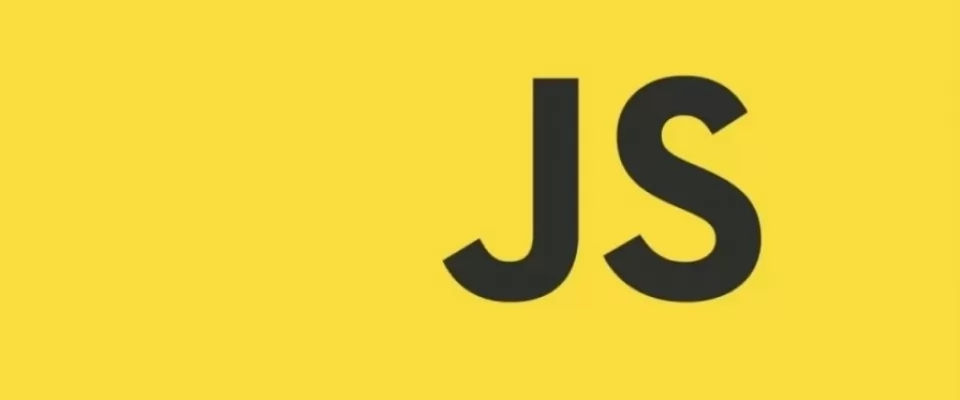
When programming with JavaScript there are certain conventions that you should apply, especially when working in a team environment. In fact, it is common to have meetings to discuss standards to follow. The reason is that code is much more readable when certain standards are followed. We have already seen in another article some of the worst JavaScript practices, explaining certain things to avoid. But when we talk about good practices we are not only referring to those that have a better performance, but also to the way you program. In addition to making sure that the code is syntactically correct, you should also be concerned about styles; that is, things like where you place the opening and closing braces or the spaces you use as indentation or indentation in the code.
What are programming styles
Programming styles are nothing more than an agreement you make with your team and yourself about the styles and standards you will follow in a project. The goal of following a style is to achieve a consistent result, with code that is easy to understand and maintain. Even if you are working alone on a personal project you should follow certain standards. You may not see why during its initial release, but you will when you modify the code in a few months or when another programmer starts working on the project as well.
The importance of styles
Programming is something similar to craftsmanship, where factors such as creativity, perseverance and expertise come into play. For example, you can paint a wall and leave it smooth, with everything perfectly clean around you, or you can paint the wall with irregularities or hand marks, fill the floor with paint, and leave it all over. The same thing happens with programming, becoming even more important, because when many programmers modify the same code over and over again, if each one applies his own rules, the result may look more like the second case.
It is very common that some inexperienced programmers do not care about this, since they have not yet been able to understand its importance. I was also one of them and absolutely all programmers have been. Besides, it is in this kind of little things where the team leaders will perceive your experience.
Sets of style standards
There are different sets of standards that you could follow. Each company has its own, and that is what styles are all about, following the same ones within the framework of a company or project, without there being a set that is better or worse. Here are the two most famous sets of styles:
- On the one hand you have the Google JavaScript styles.
- On the other hand you have the AirBnb JavaScript styles.
In my case, the styles I follow are very similar to AirBnb's, as they are the ones I've gotten used to in the companies I've worked for. If you prefer another set use it, but don't change it until you finish the project.
You also have the option of using tools like ESLint or Prettier, which somehow force you to use certain rules.
Method, function and variable notation
There are different types of notations that you could follow when defining the names of functions and variables. The most famous are the following:
Camel Case: This style combines the words of the names you define, setting the first letter of each word to be capitalized except for the first word, with the rest of the letters being lowercase. If you wanted to define an account management function using Camel Case, its name would be accountManagement.
Pascal Case: This style combines the words of the names you define, establishing that the first letter of each word is in upper case, including the first word, the rest of the letters being in lower case. If you wanted to define an account management function using Pascal Case its name would be GestionDeCuentas.
Snake Case: This style combines the words of the names you define, setting all letters to lower case and all words to be separated by an underscore. If you wanted to define an account management function using Snake Case its name would be account_management.
Kebab Case: This style combines the words of the names you define, setting all letters to lower case and all words to be separated by a hyphen. If you wanted to define an account management function using Snake Case its name would be account-management.
There is no better method, although the truth is that in JavaScript the Kebab Case would be practically discarded. The usual is to use Pascal Case for class names and Pascal Case for almost everything else, although there are many developers who prefer to define variable names with Snake Case. If you want more examples, see the guide with the different types of notation for names.
Rules to use: Yours
That's the way it is and will be as long as you are consistent. Here are mine, which although they are very similar to AirBnb's, they don't coincide 100%:
Semicolons: Many developers choose not to put them at the end of each line, although in my case I use them religiously at the end of each line to avoid possible problems that could occur in some cases.
Spaces: I always prefer to use more spaces than less. I usually use them whenever I close a parenthesis unless it is the end of a line and also before and after the symbols of arithmetic operations such as +, -, / or *, checks or conditions.
Blank lines: I use a line break to separate blocks of code that deal with different logical operations. This way I think it is easier to read the code.
Indenting: I use 4 spaces instead of pressing the tab key. It is also common to use two spaces.
Line length: The length of my lines does not exceed 120 characters. Many developers opt for 80 characters, but I find it too short. I have a bad habit of using lines that are too long.
Comments:I usually use comment blocks to document the code, and in case of commenting something, I write the comment in the line above the one I want to comment instead of writing it at the end of the line. In the same way, I only write the comments that are necessary. That is, I don't use comments when the code blocks that follow the JSDoc standard are sufficient or when the variable names make it very easy to understand what I want to do.
Variable declarations: I never declare variables with var. I use const when a value or reference is not going to change, and let when I want to declare a variable. I always declare constants first and then variables, being both at the beginning of the document in the case of global variables, or at the beginning of functions, in the case of local variables. This way we avoid the unwanted effects of JavaScript hoisting.
Function structure: I use arrow functions whenever possible because of the treatment of this. Sometimes, as in the case of constructors, it is not. Whenever I can, I define functions like this:
const miFuncion = (a, b) => a * b;
Variable names: I always use Camel Case, although until not too long ago I used Snake Case. This is an example of camelCase
Class names: In this case I use Pascal Case, both in the class name and in the name of the file that includes it. This is an example of PascalCase
.
Single or double quotes: Whenever possible I use single quotes instead of double quotes. I usually reserve double quotes for HTML attribute tags, so that if I have to insert JavaScript inside them, I can do it with double quotes. I also apply this criterion with other programming languages such as PHP.
Template Literals: These are expressions that use inverted quotation marks ` to define strings. Whenever I have to insert variables in text strings, I use these quotes, inserting the variable in the middle. In the following tutorial you can find more information about template literals. Example: `Here I am inserting a ${variable}` .
Function and method names: As in the case of variables, I use Camel Case for both functions and methods of classes. Example: CamelCase.
If statements: In this case I usually use two varieties, since I can place the statements in one or several lines depending on how long they are:
// If normal if (condition) { // code } // If con un else if (condition) { // código } else { // code } // If with several else if (condition) { // code } else if (condition) { // code } else { // code }
In the case of very short statements, I use a single line. This is something quite criticized by some developers but I find it very readable as long as the statements are very short, like when you make assignments to a variable. In any other case I use several lines:
// If normal if (statement) variable = true; // If with one else if (statement) variable = true; else variable = false;
Switch statements: In this case I always define a default condition and also use several lines:
switch (expression) { case expression: // code default: // code }
For loops: I always use several lines. In this case I always initialize the element defining the loop iterations in its own definition. I consider this better than inserting it in the condition. I separate the different elements of the loop definition by a ;
:
for (initialization; condition; update) {
// code
}
While loops: I always use several lines:
while (condition) { // code }
Do while loops: I don't usually use this loop too much. In several lines:
do { // code } while (condicion);
Try/catch/finally statements: This is how I define them:
// try catch try { // code } catch (error) { // code } // try catch finally try { // code } catch (error) { // code } finally { // code }
Whatever style you choose, remember that the important thing is to be consistent and maintain it throughout your project.
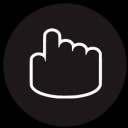
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
