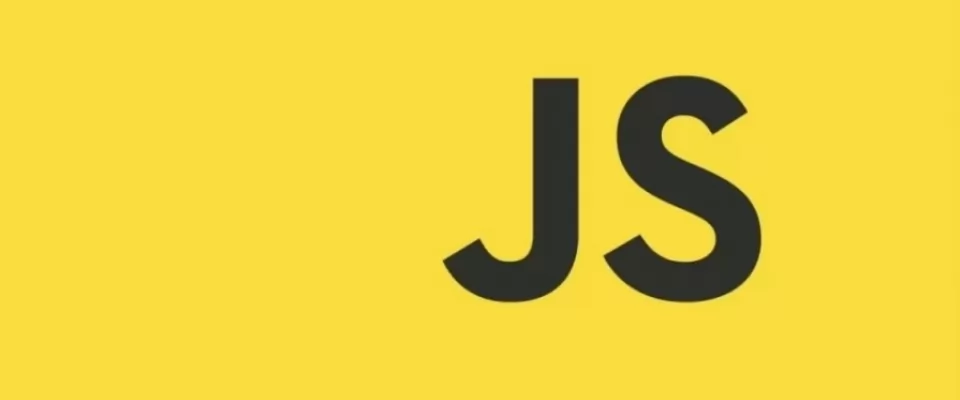
Creating dynamic PDF files directly in the browser is possible thanks to the jsPDF JavaScript library.
In the last part of this article we have prepared a practical tutorial where I show you how to implement the library. Below you can see the completed exercise.
The client side is gaining ground over the server side
The JsPDF library is an example of this. Through its API, PDF files can be generated on demand, directly in the browser. This is perfect for creating tickets, reports or certificates in real time.
In fact, this library supports both front end and back end development. Its JavaScript API can be implemented in the browser and in NodeJs environments alike. However, on this occasion, we will focus on the front end implementation.
JsPDF is a tool created by the digital agency Parallax. Its repository is actively maintained by a team of 191 people. And at the time of writing, it boasts more than 25K stars in its Github repository.
The "packaged" version occupies about 350Kb. Around here, we are used to see lighter libraries. But considering the problem it solves, it's understandable that it takes up that much.
Of course, you can install it using the command "npm install jspdf -save", and integrate it comfortably into your project.
Creating PDF is easy with JavaScript and jsPDF
Its API exposes a large number of methods, properties and modules. The presentation page of the library offers a perfectly ordered documentation. Even so, it is advisable to visit the "live demo" section. In this section, you can see several examples of application of the library.
In any case, it is essential to start by instantiating an object of the jsPDF class. This object, by convention, we call it "doc", referring to the PDF document it represents.
As usual, instantiating the class, you can pass an object with optional configuration parameters. Properties such as page formatting and orientation can be decided. The units of measurement and document comprehension. Even encryption, in case you want the file to be password protected.
The "doc" object is reminiscent in some respects of the "CanvasRenderingContext2D" of the canvas tags. Through methods such as rect()
, lineTo()
, ellipse()
, stroke()
or fill()
, vector graphics can be drawn. Also, through the text()
method, text can be included at certain points of the pages. On another occasion, we saw another library whose API was also very similar to that of Canvas.
Creating art with JavaScript and P5.js
But jsPDF goes further. In addition, it exposes functions to create more complex elements, or PDF files themselves. For example, through the table() method, you can create data tables with rows and columns. Or through the createAnnotation() method, you can even include comments to the file.
The option to include more pages in the document is available through the addPage() method.
Once the document is set up and ready, jsPDF solves the whole downloading process by calling the save() function.
Next, we will see how to create PDF files dynamically with JavaScript and JsPDF.
Create a PDF file dynamically with JavaScript
To create a PDF file dynamically with JavaScript, we will start by programming a simple web application. In this application, the user will be able to create and export a fake character profile. Starting from a template, we can enter data such as the name, the type of character or the skills. And later, download a PDF with this information.
We will start by preparing the user interface. The interface will be composed, on the one hand, of an HTML form with different fields. And on the other hand, an iframe tag, which will help us to preview the pdf, before downloading it.
In the form we will include the following fields: name, nickname, description, type, strength, magic and speed. Then we will also include an element to display error messages when necessary, and a couple of buttons. The buttons will allow the user to view the file and download it.
Then we include an empty iframe tag. Using the Tailwind css style library, we will include CSS classes to all the elements to style them. It is important to include "id" attributes in the form, error message, and iframe tags. Later, these ids will be used for the Js script.
It should be noted that in the list of character type options, a special data-image-url
attribute has been included.
This will serve to include a specific image for each type.
<body class="h-screen flex flex-col items-center bg-slate-50"> <div class="block md:flex main-layout w-screen"> <div class="flex justify-center items-center p-5"> <form id="form-character-profile" class="w-full"> <div class="mb-5"> <label for="" class="block text-sky-900 font-bold"> Name: </label> <input type="text" name="name" placeholder="Name" value="Mario" class="block w-full p-3 border-2 border-sky-700 bg-sky-50 rounded" /> </div> <div class="mb-5"> <label for="" class="block text-sky-900 font-bold"> Sobrenombre: </label> <input type="text" name="surname" placeholder="Last Name" value="Mario" class="block w-full p-3 border-2 border-sky-700 bg-sky-50 rounded" /> </div> <div class="mb-5"> <label for="" class="block text-sky-900 font-bold">Description </label> <textarea name="description" cols="30" rows="5" class="block w-full p-3 border-2 border-sky-700 bg-sky-50 rounded" placeholder="Descripción" >Mario is a balanced character in terms of weight and speed. His cape can be used as a counterattack, repelling any projectile or approach. His jumping movement has the characteristic that if he touches an enemy he can obtain coins and, at the same time, send him out of the screen. .</textarea> </div> <div class="mb-5"> <label for="" class="block text-sky-900 font-bold"> Tipo: </label> <select name="type" class="block w-full p-3 border-2 border-sky-700 bg-sky-50 rounded"> <option value="player" data-image-url="imgs/Mario_Bross.png" >Player 1</option> <option value="enemy" data-image-url="imgs/goomba.png" >Enemy</option> <option value="enemy 2" data-image-url="imgs/koopa.png" >Ememy 2</option> <option value="player 2" data-image-url="imgs/luigi.png" >Player 2</option> <option value="player 3" data-image-url="imgs/toad.png" >Player 3</option> <option value="enemy-3" data-image-url="imgs/Wario.png" >Enemy 3</option> </select> </div> <div> <div> <label class="block text-sky-900 font-bold">Strength:</label> <input type="range" name="strength" value="40" class="w-full" /></div> <div> <label class="block text-sky-900 font-bold">Strength:</label> <input type="range" name="strength" class="w-full" value="90" /> </div> <div> <label class="block text-sky-900 font-bold">Speed:</label> <input type="range" value="60" name="velocity" class="w-full" /></div> </div> <div id="error-message-container" class="hidden text-red-500 bg-red-100 p-4 rounded mt-3"> *Error message </div> <hr class="my-5"> <div class="mt-3"> <button type="button" class="bg-sky-500 text-white px-3 py-2 rounded preview-pdf-btn">Preview PDF</button> <button type="submit" class="bg-sky-500 text-white px-3 py-2 rounded">Download PDF</button> </div> </form> </div> <div> <iframe id="frame" src="" frameborder="0"> </iframe> </div> </div> </body>
In the SCSS file, we will include the basic Tailwind resources, and add a few lines of additional CSS.
@tailwind base; @tailwind components; @tailwind utilities; body { margin: 0; padding: 0; } .main-layout { & > div { width: 100%; iframe { width: 100%; min-height: 500px; height: 100%; max-height: 800px; } } }
With the interface structure and styles ready, we can start programming the script. First, we import the JsPDF library and styles.
import { jsPDF } from "jspdf"; import "./SCSS/index.scss";
Next, we store in variables, references to elements of the DOM. These elements will be the form, the preview button, the error message container, and the iframe.
const formCharacterProfile = document.querySelector("#form-character-profile"); const previewBtn = formCharacterProfile.querySelector(".preview-pdf-btn"); const errorMessageContainer = document.querySelector("#error-message-container"); const frame = document.querySelector("#frame");
Before generating the pdf with the JsPDF library, it is important to extract and validate the form data. To do this, we declare the function handleOnSubmitForm. This function will be in charge of going through all the fields of the form with attribute "name". For each one, it will try to save in the variable "characterData" the property and the value. In case a value is not defined, it will throw an error and update the interface to show that error.
const handleOnSubmitForm = (e) => e.preventDefault(); try const characterProperties = Array.from(e.target.querySelectorAll("[name]")); const characterData =}; errorMessageContainer.classList.add("hidden"); for (let i = 0, j = characterProperties.length; i < j; i++) const field = characterProperties[i]; const attribute = field.getAttribute("name"); const value = field.value; if (!field.value) throw new Error(`Empty ${attribute} field!`); } characterData[attribute] = value; if (attribute === "type") const option = field.querySelector(`[value=${value}]`); characterData[attribute] = name: option.innerHTML, image: option.dataset.imageUrl, }; } } generatePDF(characterData, e.isPreview); } catch (err) errorMessageContainer.innerHTML = err.message; errorMessageContainer.classList.remove("hidden"); } };
At the end of the function, if everything is correct, it will call generatePDF()
.
GeneratePDF is the function that will create the PDF file dynamically. It will receive two arguments. The first one is the object that describes the properties of the character. And the second is a boolean, to decide whether to preview or download.
At the beginning of this function we instantiate the jsPDF class. Although we could pass a configuration object, we will leave the predefined values. By means of the object "doc" we position all the texts and images. To do this, we use the methods text()
and addImage()
.
Additionally, we can style those texts with methods such as setFont()
and setFontSize()
. As well as adding some separator lines, with the line()
method.
Once the content is "laid out" in the document, we generate the PDF. If the "preview" variable is "true, we update the iframe property, calling the "output("bloburl")" method. In case it is false, we can directly call the save()
method. This last method will create and download the file for us with the name indicated.
const generatePDF = (characterData, preview) => { const doc = new jsPDF(); doc.setFontSize(40); doc.setFont("helvetica", "bold"); doc.text(characterData.name, 60, 30); doc.setFont("helvetica", "normal"); doc.text(characterData.surname, 60, 42); doc.addImage(characterData.type.image, "PNG", 5, 0, 50, 50); doc.setFontSize(20); const docWidth = doc.internal.pageSize.getWidth(); const docHeight = doc.internal.pageSize.getHeight(); doc.line(0, 60, docWidth, 60); doc.setFont("helvetica", "italic"); const splitDescription = doc.splitTextToSize( characterData.description, docWidth - 20 ); doc.text(splitDescription, 10, 80); doc.setFontSize(20); doc.setFont("helvetica", "bold"); doc.text(characterData.type.name, docWidth - 20, 45, { align: "right" }); doc.line(0, docHeight - 60, docWidth, docHeight - 60); doc.text(`Fuerza: `, 10, docHeight - 40); doc.text(`Magia: `, 10, docHeight - 30); doc.text(`Velocidad: `, 10, docHeight - 20); doc.setFont("helvetica", "normal"); doc.text(`${characterData.strength}`, 50, docHeight - 40); doc.text(`${characterData.magic}`, 50, docHeight - 30); doc.text(`${characterData.velocity}`, 50, docHeight - 20); if (preview) { frame.src = doc.output("bloburl"); return; } doc.save(`${characterData.name}-${characterData.surname}`); };
Finally, the only thing left to do is to listen to the form submission events, and click on the preview button. When submitting the form, we will call the function handleOnSubmitForm
. And when clicking on the button, we will also trigger a submission. However, in that case, we will expand the event, with the "isPreview" boolean set to "true.
previewBtn.addEventListener("click", () => { const event = new Event("submit"); event.isPreview = true; formCharacterProfile.dispatchEvent(event); }); formCharacterProfile.addEventListener("submit", handleOnSubmitForm);
With this simple exercise, we have seen how to easily create PDF files with JavaScript and JsPDF.
Connecting the online and offline world
The communication power of a PDF file should not be underestimated. Despite being something seemingly simple, it can be the perfect bridge that connects the online and offline world.
A clear example of this statement is that today, many people still print tickets and airline tickets. And a dynamically generated QR appears on them, proving their authenticity.
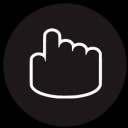
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
