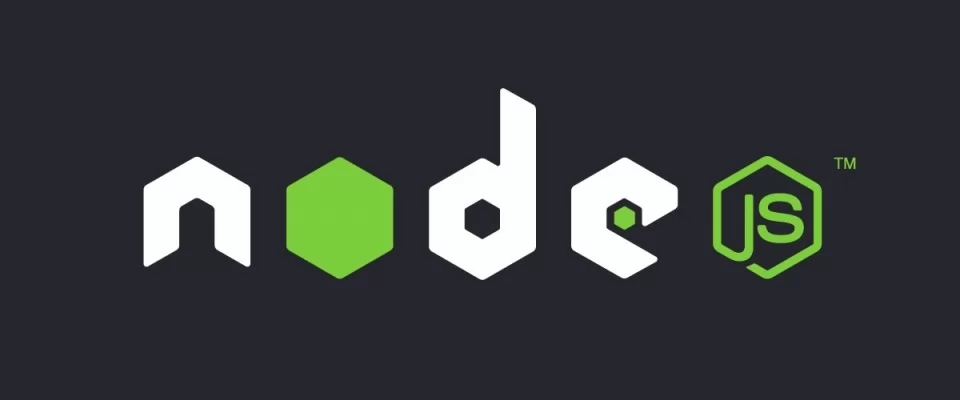
Let's see how you can connect to a MySQL database using Node.js, the popular JavaScript runtime environment.
Before we start, it is important to note that you must have Node.js installed on your system. Likewise, you must create a MySQL database.
Create a MySQL database
If you haven't already done so, you can create the database in any way you like. You can create it using applications such as phpMyAdmin or manually using the MySQL command line. To create it via the command line, follow these steps:
Connect to MySQL from the command line and enter the password when prompted:
mysql -u USER -p
Create a sample table, which in this case we will call employees:
CREATE DATABASE employees;
Select the database you have just created:
USE employees;
Create a table in the database:
CREATE TABLE IF NOT EXISTS employees ( id int(11) NOT NULL AUTO_INCREMENT, name varchar(50), PRIMARY KEY (id) );
Insert some example data in the table:
INSERT INTO employees (nombre) VALUES ("Silvia"); INSERT INTO employees (nombre) VALUES ("Luigi"); INSERT INTO employees (nombre) VALUES ("Alejandro");
You can now run queries on the table.
Install node-mysql
You will need to have the node-mysql package installed, so if you don't have it installed, you will need to install it. To do this you must use the npm package manager:
npm install mysql
After installing node-mysql you will be able to connect to the database via Node.js.
Establish the MySQL connection
Create a Node.js script.
The first thing we do in this example is create a connection object to the MySQL database.
We use the table employees as an example, but replace it with the name of the database you want to use. Similarly, replace USER and PASS with the MySQL user data.
var mysql = require('mysql'); var conexion= mysql.createConnection({ host : 'localhost', database : 'employees', user : 'USER', password : 'PASS', }); conexion.connect(function(err) { if (err) { console.error('Error de conexion: ' + err.stack); return; } console.log('Connected to the identifier ' + conexion.threadId); });
Once the connection is established, you will be able to execute queries using the query method:
conexion.query('SELECT * FROM empleados', function (error, results, fields) { if (error) throw error; results.forEach(result => { console.log(result); }); });
Finally, terminate the connection:
connection.end();
And that's it. If you want more information about node-mysql, we recommend you to consult this guide.
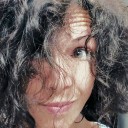
Silvia Mazzetta
Web Developer, Blogger, Creative Thinker, Social media enthusiast, Italian expat in Spain, mom of little 9 years old geek, founder of @manoweb. A strong conceptual and creative thinker who has a keen interest in all things relate to the Internet. A technically savvy web developer, who has multiple years of website design expertise behind her. She turns conceptual ideas into highly creative visual digital products.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
