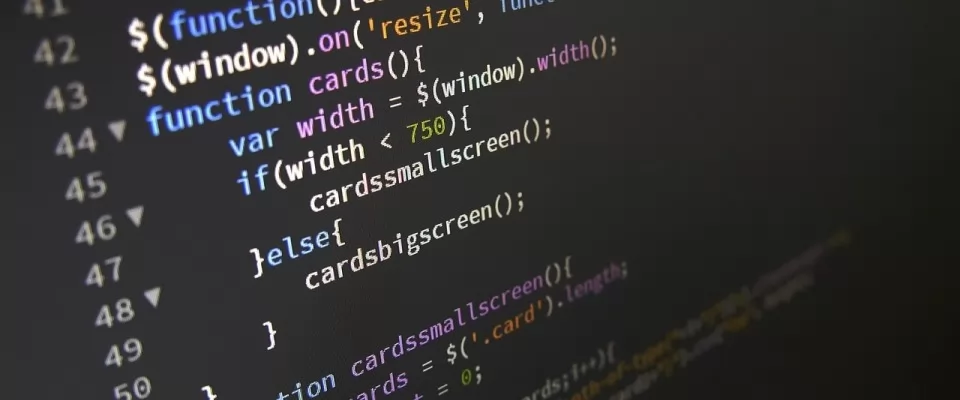
Part one: Introducing the DOM - what is it and why should I get to know it?
For those who are new to web development, surely the amount of new terminology can sometimes be discouraging. But when it comes to learning JS, some of the first new concepts you definitively should wrap your head around is the Document Object Model and what it implies.
To keep it simple and not to resort to citing formal definitions, the DOM represents the website and its elements as a logical structure, resembling a tree with its branches. Each branch is a node, and each node contains objects.

When we have access to a certain node in the DOM, there are ways to gain access to other nodes in the DOM using its related nodes. We can move up or down the DOM tree, or we can move sideways staying at the same DOM level. This is also called traversing the DOM (which sounds super cool if you ask me).
But how can we make use of all this and how is it related to programming in JS?
Since the DOM allows us to access to all the elements of a web page, by using scripting languages, like for example JS, we can make modifications to them. In this way, we are able to change the document's structure, style, or content.
Moreover, the nodes from our imaginary tree allow to attach event handlers to them. Once an event is triggered, the event handlers get executed. This opens up a whole new world of possibilities to control the web page behavior to us. The most obvious ways we can make use of this are:
- Reacting to user input – change the page’s content based on the data entered by the user, and even send those data to another server.
- Using data received from APIs and use them to update the page's contents.
As we already said, the DOM has a tree-like structure: starting with the root element, the
tag. From here, each child element branches out. Usually, the root element is a parent to two child elements:and. The html tag is then parent to any elements within it — paragraphs, articles, sections, buttons, forms,etc. Those child elements can then have children of their own: a form will have labels and inputs, an article can have a heading, text content, links, etc.How to access the DOM elements in JS
There are various ways available for us, but let’s start with using the “document” object and its methods.
document.getElementsByTagName();
As the name suggest, we need to specify the tag name in order to use this method. So, for example if we want to select all paragraph elements, we would usedocument.getElementsByTagName(‘p’);
Of course, it would be useful to save this reference in a variable, for example like this:var paragraphs = “document.getElementsByTagName(‘p’);
It is important to know that thegetElementsByTagName()
method returns an object of type HTMLCollection, or in other words, a collection of all elements in the document with the specified tag name. The HTMLCollection object represents a collection of nodes. The nodes can be accessed by index numbers. The index starts at 0. This is similar to how we would acces an array. But beware! A HTML collection is NOT an array object, so we cannot use Array methods like push(), pop() or indexOf(). But, in order to access the first element in our collection, we use the following:var firstParagraph = paragraphs[0];
We create a constant called firstParagraph and then we assign the first (because, as it always is the case with indexes, we start counting at 0) element of our “ paragraphs” HTML collection to it.
document.getElementById();
Very useful, but careful – as you can see if you observe its name, this method returns only ONE element, not a collection. This makes perfect sense, if you consider that a well structured and correctly designed web should only have one element per ID.document.getElementsByClassName();
Selects all elements with matching class name, for example:var redBackground = document.getElementsByClassName(“bg_red”);
this line of code enables us to reference all elements with class “bg_red” with the constant called redBackground;document.querySelector();
This method returns the first element in the DOM that matches the given selector(s). The selectors used may be tag names, classes, id attributes, or a mixture of the three:- document.querySelector('article') — returns the first "article" element
- document.querySelector('.text-center') — returns the first element with class “text-center”
- document.querySelector('#main') — returns the element with id attribute “main”
- document.queryselector('h2.text-center') — returns the first "h2"element with class “text-center”
There is one more method I would like to add to the list:
document.querySelectorAll();
It returns a Node List object, which is almost the same as the HTML collection object which we commented earlier. In fact, some older browsers return a Node List object instead of an HTML Collection for methods like getElementsByClassName().
This seems a bit confusing, so let’s see what similarities and differences there are between a node list object and a HTML collection object:
- They both resemble an array-like list of objects but they are not arrays! you cannot use Array Methods, like valueOf(), push(), pop(), or join() on them.
- Both have a length property, which gives us the number of items in the list.
- Both use an index, (starting with 0) so that each of their items can be accessed or looped through similar to how we would be accessing elements in an array.
BUT:
- HTML Collection items can be accessed by their name, id, or index number.
- Node List items can only be accessed by their index number.
- Only the Node List object can contain attribute nodes and text nodes (we haven’t covered these yet).
Lastly, there are DOM methods and properties which allow us to use the “family relationships” between the DOM elements to navigate and select them (this is the actual “traversing of the DOM”) . But why do we need to learn to traverse the DOM, if we know methods such as document.querySelector?
Because it’s always quicker and easier to move from an element to another, then doing a full search.
You can traverse in three directions:
- Downwards: element.children — returns a HTML Collection containing all child elements of element
- Sideways element.nextElementSibling + element.previousElementSibling — these allow us to iterate through elements on the same relationship level, like between list items
- Upwards element.parentElement — element's parent element (any given element can only have up to one parent)
To summarize – the DOM allows JavaScript to access and manipulate the page content. In order to access the elements, we need to make ourselves acquainted with using the corresponding object methods, like document.getElementById(), document.getElementsByClassName(), getElementsByTagName() and document.querySelector().
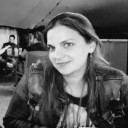
Iveta Karailievova
Originally coming from a marketing background, decided to turn her life around and immerse herself into the wonderful exciting and most importantly – never boring world of technology and web development. Proud employee at MA-NO . Easily loses track of time when enjoying working on code. Big fan of Placebo, cats and pizza.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
