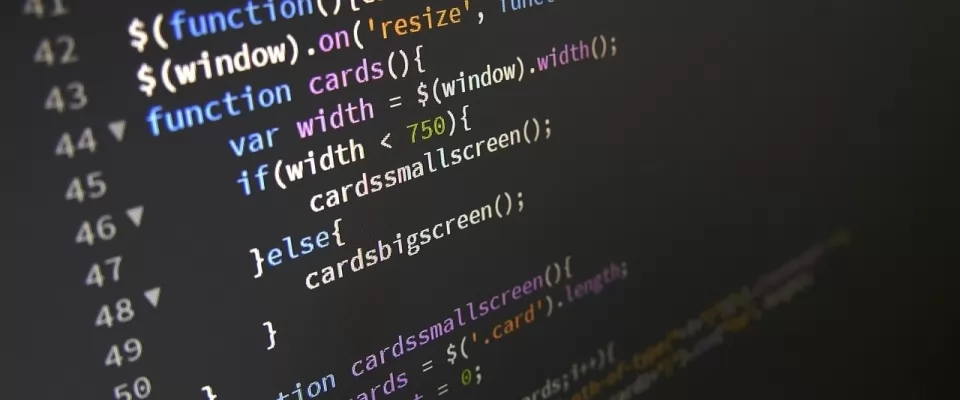
Modifying the DOM
In the previous aticle First steps into JavaScript – a practical guide we covered the basics of the DOM and its relation to us becoming JS literate. Now that we know how to access DOM elements, we can continue with learning how to change them.
But before we start, let's make sure we are familiar with a few facts:
When we talk about HTML elements in the DOM, we are actually talking about objects (that's their definition). Objects have properties (this means values, we can get or set) and methods (or actions, stuff we can do to/with the objects). The programming interface is the properties and methods of each object. Now that we cleared this up, we can start making modifications.
Changing content:
Many times, two simple properties will be all we need to use to do so. First of them is textContent. It uses straight text, does not parse HTML, and is therefor faster. Its output is text/plain.
On the other hand, we have innerHTML, which parses content as HTML, outputs text/html and thus takes longer.
Here is a simple example of how these two differ:
let myLink = document.getElementById('myLink');
myLink.textContent = '<a href="https://www.ma-no.org/">Ma-No Web</a>';
This will give us the following output:
<a href="https://www.ma-no.org/">Ma-No Web</a>
But when we use:
myLink.innerHTML = '<a href="https://www.ma-no.org/">Ma-No Web</a>';
Our output will be:
Ma-No Web
Changing CSS classes
This is easily done by using the classList property, and then the corresponding method (add, remove):
myElement.classList.add(className);
myElement.classList.remove(className);
What would be the result of:
let h2 = document.getElementById('#heading2);
h2.classList.add('red','underlined');
h2.classList.remove('red');
Well, the selected element, in our case h2 would first receive two new CSS classes ("red" and "underlined") and then lose one class in the next step ("red);
As you can see, it's possible to add multiple comma-separated classes with the classList.add() method.
One important thing to notice here, which even though may seem obvious I think is good to remark: classList is a property, and can only be called on a single element, in our example we called it on a single h2 element, which we got accessed to by using the getElementById method. If we tried accessing a collection of elements, using getElementsByTagName for example, the classList property would not be available.
Changing styles
Sometimes we only need to make a quick change of the style of our element, and we can do this without having to create a CSS class simply by using the style property:
header.style.margin = '10px';
We just use an element's style property to directly modify its styles.
Working with attributes
It seems like a logical thing to want to be able to add or modify an element's attribute, and it is fairly easy to do so.We just need to make ourselves familiar with a few methods, with really self-explanatory names:
The setAttribute() method, where we pass two arguments - the name of the attribute we want to set and its value. The method then adds the specified attribute to an element, and gives it the specified value. In this way, we can change for example the element's ID, but also class, style, etc. Here's an example:
myElement.setAttribute("id","#01");
or
document.getElementById("myLink").setAttribute("href", "https://www.ma-no.org");
The removeAttribute() method removes the specified attribute from an element. Use the getAttribute() method to return the value of an attribute of an element. If the specified attribute already exists, only the value is set/changed.
Maybe are some of you wondering, if we can modify attributes with those methods, why not use them to also change the "style" attributes. Although it is possible, it is not recommended.
Instead we use properties of the Style object as we described earlier, because in this way we make sure that other CSS properties that may be specified in the style attribute will not be overwritten.
Adding new elements
We have to follow these steps to create and append new elements to our page:
- first we create the element we need by calling the createElement DOM method, like this:
let mySection = document.createElement('section');
- now we have our new section created, but it is empty, we might want to append some elements to it. Those elements first need to be created too, so again, we use the createElement method and store these newly build elements in variables:
let myArticle = document.createElement('article');
let myParagraph = document.createElement('p');
- Even though these variables are so far not visible to us, they hold references to DOM elements and because of this, we are able to use the methods we've mentioned before to add content:
myParagraph.textContent = "whatever";
- Nice! Now the elements exist somewhere out there, in the DOM. Let's put them together, using the appendChild method. First we append the paragraph to the article and the the article to the section:
mySection.appendChild(myArticle);
- Finally, we need to append our section to some element which already exists in the DOM, like for example 'main'. Remember, to access the 'main' element, we use for example the getElementByTagName method:
document.getElementByTagName('main').appendChild('mySection);
- And what if we need to replace or remove an element?
We use either the replaceChild() or the removeChild() method, according to our needs. Notice that both methods call on the parent of the element you wish to remove. So to remove an element when we do not have direct access to the parent element can be done with this little trick:
myElement.parentElement.removeChild(myElement);
A short recap:
After gaining access to our DOM elements, we want to modify them. This is done by either using their properties or methods. We can create and add new elements to the DOM, modify the elements' classes and style and modify their attributes.
And that's it! These are the basics you need to know so that we can move up a level and learn how to make more magical things happen, like reacting to events (such as mouse clicks or form inputs).
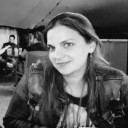
Iveta Karailievova
Originally coming from a marketing background, decided to turn her life around and immerse herself into the wonderful exciting and most importantly – never boring world of technology and web development. Proud employee at MA-NO . Easily loses track of time when enjoying working on code. Big fan of Placebo, cats and pizza.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
