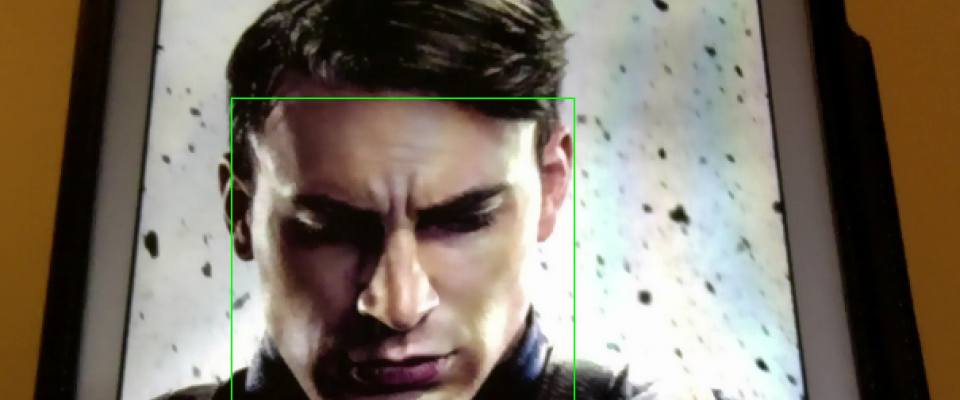
Face detection is a computer technology that determines the locations and sizes of human faces in arbitrary (digital) images. It detects facial features and ignores anything else, such as buildings, trees and bodies.
There is so much to say about face detection and all its algorithms, but first we want to start publishing the Maurice Svay's PHP class, he explains that he was looking for a face detection script for PHP, but wasn't able to find one working without OpenCV (Open Source Computer Vision), an opensource lib that was originally developed by Intel.
OpenCV seems to perform well but you need to be able to install it on your server, so he coded his own pure PHP solution, that does not require any library to be installed on the server.
The class itself has been translated from a javascript code.
The source code of the class:
<?php // // This program is free software; you can redistribute it and/or // modify it under the terms of the GNU General Public License // as published by the Free Software Foundation; either version 2 // of the License, or (at your option) any later version. // // This program is distributed in the hope that it will be useful, // but WITHOUT ANY WARRANTY; without even the implied warranty of // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the // GNU General Public License for more details. // // You should have received a copy of the GNU General Public License // along with this program; if not, write to the Free Software // Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. // // @Author Karthik Tharavaad // [email protected] // @Contributor Maurice Svay // [email protected] class Face_Detector { protected $detection_data; protected $canvas; protected $face; private $reduced_canvas; public function __construct($detection_file = 'detection.dat') { if (is_file($detection_file)) { $this->detection_data = unserialize(file_get_contents($detection_file)); } else { throw new Exception("Couldn't load detection data"); } //$this->detection_data = json_decode(file_get_contents('data.js')); } public function face_detect($file) { if (!is_file($file)) { throw new Exception("Can not load $file"); } $this->canvas = imagecreatefromjpeg($file); $im_width = imagesx($this->canvas); $im_height = imagesy($this->canvas); //Resample before detection? $ratio = 0; $diff_width = 320 - $im_width; $diff_height = 240 - $im_height; if ($diff_width > $diff_height) { $ratio = $im_width / 320; } else { $ratio = $im_height / 240; } if ($ratio != 0) { $this->reduced_canvas = imagecreatetruecolor($im_width / $ratio, $im_height / $ratio); imagecopyresampled($this->reduced_canvas, $this->canvas, 0, 0, 0, 0, $im_width / $ratio, $im_height / $ratio, $im_width, $im_height); $stats = $this->get_img_stats($this->reduced_canvas); $this->face = $this->do_detect_greedy_big_to_small($stats['ii'], $stats['ii2'], $stats['width'], $stats['height']); $this->face['x'] *= $ratio; $this->face['y'] *= $ratio; $this->face['w'] *= $ratio; } else { $stats = $this->get_img_stats($this->canvas); $this->face = $this->do_detect_greedy_big_to_small($stats['ii'], $stats['ii2'], $stats['width'], $stats['height']); } return ($this->face['w'] > 0); } public function toJpeg() { $color = imagecolorallocate($this->canvas, 255, 0, 0); //red imagerectangle($this->canvas, $this->face['x'], $this->face['y'], $this->face['x']+$this->face['w'], $this->face['y']+ $this->face['w'], $color); header('Content-type: image/jpeg'); imagejpeg($this->canvas); } public function toJson() { return "{'x':" . $this->face['x'] . ", 'y':" . $this->face['y'] . ", 'w':" . $this->face['w'] . "}"; } public function getFace() { return $this->face; } protected function get_img_stats($canvas){ $image_width = imagesx($canvas); $image_height = imagesy($canvas); $iis = $this->compute_ii($canvas, $image_width, $image_height); return array( 'width' => $image_width, 'height' => $image_height, 'ii' => $iis['ii'], 'ii2' => $iis['ii2'] ); } protected function compute_ii($canvas, $image_width, $image_height ){ $ii_w = $image_width+1; $ii_h = $image_height+1; $ii = array(); $ii2 = array(); for($i=0; $i<$ii_w; $i++ ){ $ii[$i] = 0; $ii2[$i] = 0; } for($i=1; $i<$ii_w; $i++ ){ $ii[$i*$ii_w] = 0; $ii2[$i*$ii_w] = 0; $rowsum = 0; $rowsum2 = 0; for($j=1; $j<$ii_h; $j++ ){ $rgb = ImageColorAt($canvas, $j, $i); $red = ($rgb >> 16) & 0xFF; $green = ($rgb >> 8) & 0xFF; $blue = $rgb & 0xFF; $grey = ( 0.2989*$red + 0.587*$green + 0.114*$blue )>>0; // this is what matlab uses $rowsum += $grey; $rowsum2 += $grey*$grey; $ii_above = ($i-1)*$ii_w + $j; $ii_this = $i*$ii_w + $j; $ii[$ii_this] = $ii[$ii_above] + $rowsum; $ii2[$ii_this] = $ii2[$ii_above] + $rowsum2; } } return array('ii'=>$ii, 'ii2' => $ii2); } protected function do_detect_greedy_big_to_small( $ii, $ii2, $width, $height ){ $s_w = $width/20.0; $s_h = $height/20.0; $start_scale = $s_h < $s_w ? $s_h : $s_w; $scale_update = 1 / 1.2; for($scale = $start_scale; $scale > 1; $scale *= $scale_update ){ $w = (20*$scale) >> 0; $endx = $width - $w - 1; $endy = $height - $w - 1; $step = max( $scale, 2 ) >> 0; $inv_area = 1 / ($w*$w); for($y = 0; $y < $endy ; $y += $step ){ for($x = 0; $x < $endx ; $x += $step ){ $passed = $this->detect_on_sub_image( $x, $y, $scale, $ii, $ii2, $w, $width+1, $inv_area); if( $passed ) { return array('x'=>$x, 'y'=>$y, 'w'=>$w); } } // end x } // end y } // end scale return null; } protected function detect_on_sub_image( $x, $y, $scale, $ii, $ii2, $w, $iiw, $inv_area){ $mean = ( $ii[($y+$w)*$iiw + $x + $w] + $ii[$y*$iiw+$x] - $ii[($y+$w)*$iiw+$x] - $ii[$y*$iiw+$x+$w] )*$inv_area; $vnorm = ( $ii2[($y+$w)*$iiw + $x + $w] + $ii2[$y*$iiw+$x] - $ii2[($y+$w)*$iiw+$x] - $ii2[$y*$iiw+$x+$w] )*$inv_area - ($mean*$mean); $vnorm = $vnorm > 1 ? sqrt($vnorm) : 1; $passed = true; for($i_stage = 0; $i_stage < count($this->detection_data); $i_stage++ ){ $stage = $this->detection_data[$i_stage]; $trees = $stage[0]; $stage_thresh = $stage[1]; $stage_sum = 0; for($i_tree = 0; $i_tree < count($trees); $i_tree++ ){ $tree = $trees[$i_tree]; $current_node = $tree[0]; $tree_sum = 0; while( $current_node != null ){ $vals = $current_node[0]; $node_thresh = $vals[0]; $leftval = $vals[1]; $rightval = $vals[2]; $leftidx = $vals[3]; $rightidx = $vals[4]; $rects = $current_node[1]; $rect_sum = 0; for( $i_rect = 0; $i_rect < count($rects); $i_rect++ ){ $s = $scale; $rect = $rects[$i_rect]; $rx = ($rect[0]*$s+$x)>>0; $ry = ($rect[1]*$s+$y)>>0; $rw = ($rect[2]*$s)>>0; $rh = ($rect[3]*$s)>>0; $wt = $rect[4]; $r_sum = ( $ii[($ry+$rh)*$iiw + $rx + $rw] + $ii[$ry*$iiw+$rx] - $ii[($ry+$rh)*$iiw+$rx] - $ii[$ry*$iiw+$rx+$rw] )*$wt; $rect_sum += $r_sum; } $rect_sum *= $inv_area; $current_node = null; if( $rect_sum >= $node_thresh*$vnorm ){ if( $rightidx == -1 ) $tree_sum = $rightval; else $current_node = $tree[$rightidx]; } else { if( $leftidx == -1 ) $tree_sum = $leftval; else $current_node = $tree[$leftidx]; } } $stage_sum += $tree_sum; } if( $stage_sum < $stage_thresh ){ return false; } } return true; } }
Download the file detection.dat from here
Using it is very easy, in PHP just write
$detector = new Face_Detector('detection.dat'); $detector->face_detect('your_file.jpg'); $detector->toJpeg();
Here is the result:
This was generated by the script, just changing the frame color from red to green, to make it more visible.
The class does not work that well on every photo, but it's a good start for a journey into face detection
Another solution is Face Detector Class pure 100% PHP from Felix Koch
This is a port of JViolaJones (http://code.google.com/p/jviolajones/) to PHP. The author did a great job writing understandable code. Both thumbs up!
Viola Jones is an object detection method named after Paul Viola and Michael Jones. (See their paper: http://research.microsoft.com/en-us/um/people/viola/Pubs/Detect/violaJones_CVPR2001.pdf)
Viola Jones is also the object detector from OpenCV (http://sourceforge.net/projects/opencvlibrary/). Technically a classifier (namely a cascade of boosted classifiers working with haar-like features) is trained with a few hundreds of sample views of a particular object (i.e., a face or a car), called positive examples, that are scaled to the same size (say, 20x20), and negative examples - arbitrary images of the same size.
.
<?php include("FaceDetector.php"); $detector = new FaceDetector(); $detector->scan("test.jpg"); $faces = $detector->getFaces(); foreach($faces as $face) { echo "Face found at x: {$face['x']}, y: {$face['y']}, width: {$face['width']}, height: {$face['height']}<br />\n"; } ?>
PHP FaceDetector as well as JViolaJones can handle the classifiers shipped with OpenCV or trained with OpenCV. One classifier (haarcascade_frontalface_default.xml) is shipped with this software, so you don't need to download or install OpenCV, although it is a great tool too
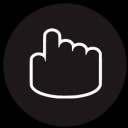
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Open source web design tools alternatives
There are many prototyping tools, user interface design tools or vector graphics applications. But most of them are paid or closed source. So here I will show you several open…
Deepfakes Detection – An Emerging Technological Challenge
It is highly probable that while browsing the Internet, everyone of us has at some point stumbled upon a deepfake video. Deepfakes usually depict well-known people doing really improbable things…
Small and medium enterprises can now earn money by holding online events on Facebook
Facebook has launched a new feature that allows small and medium enterprises, large companies, content creators, educators, media... to earn money by holding online events on the social network. Corporate…
Facebook, three questions to recognize fake news (and not share it)
Where's it coming from? What's missing? How do you feel? These are the three questions that Facebook recommends to all users to ask themselves before sharing news. The initiative is…
Facebook: how to remove hidden data and personal information
Facebook is a great social network that allows us to be always updated on all the news of our friends or family or even the most relevant news of the…
Easy Face and hand tracking browser detection with TensorFlow.js AI and MediaPipe
In March the TensorFlow team has released two new packages: facemesh and handpose for tracking key landmarks on faces and hands respectively. This release has been a collaborative effort between…
There are 470,000 WhatsApp groups indexed on Google and Facebook says it's not his fault
Want to find new WhatsApp groups? Google it. Reporter Jordan Wildon has discovered hundreds of thousands of private groups through the search engine thanks to public invitation links. Not all were…
Whatsapp is working to a new sales system integrated in the app
We already know Whatsapp is about to start displaying advertising on the platform, but there are other ways of doing business, and apparently selling using Whatsapp is very close to…
Facebook Prepack, The Next Big Thing In The JavaScript World
In the last days, the social networks were crazy about Prepack. Probably you still haven’t heard about it. It’s reasonable! It has been an open source only a few days…
8 Social Media Monitoring Tools
We think that a combination of quick response, easy use and functionality is what a company need in order to effectively run a social media campaigns. There are many tools that…
Best Days to Post on Facebook [Infographic]
Among the greatest challenges is determining exactly when to post a given piece of content so it attracts the most “likes,” comments, and retweets. A recent infographic from LinchpinSEO could help…
Facebook 10th anniversary
There are ten candles on the Facebook birthday cake. A phenomenal decade. Ten years ago it did not exist now, it has 1.23 billion users; to put that in perspective it’s something…