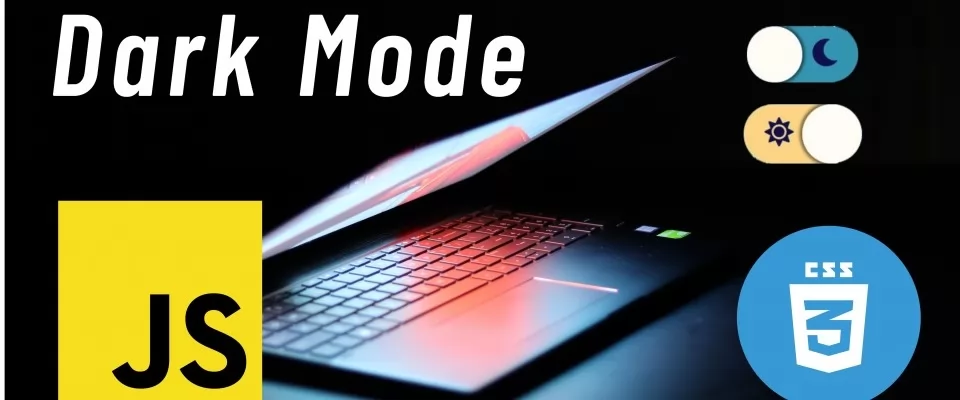
How to do an alternative color switch between dark and light theme on your website
In today’s article we are going to learn how to build pretty much standard these days on the web pages and that is the alternative color mode and switching between them. This is very useful when during night you want the user to be able to NOT suffer by staring at very bright colors, and vice versa during the day it's better not having colors so dark on your page.
Continue reading to discover how to easily make a switchable dark theme for your page and how to implement a button for it.
Why Dark Mode?
Alternative mode to display web apps or ordinary sites is getting a lot of attention in recent years. Browsers have it, Google, Youtube offers dark mode, Apple added it to its iOS, even Windows has done the same in version 10.
The dark mode is mainly used as a supplemental mode to a light theme. It allows to lessen the strain on your eyes and normally it should be easy to switch on and off.
Using JavaScript and CSS
So the first thing we need is a web page where we want to add the Dark Mode. Let's open HTML code in a code editor like Visual Studio Code, or some other you like. The first thing to do is to find the place where we want to put the icon or button to activate or deactivate the mode.
Now here we have our header and behind ‘Login’ and ‘Register’ links we will place our button, with id so we can build the functionality with JavaScript and class to be able to manipulate its style with CSS. Inside the code for the button we will place two icons, and we're going to be using FontAwesome - the icon source, where you can find most of the icons you desire.
The icons would be placed into etiquette <span>
, but first we need to link the file with icons. On our page, in the head etiquette we had already downloaded FontAwesome4 and linked to it like we can see here.
<head> <link rel="stylesheet" href="css/font-awesome.min.css"> <!-- FontAwesome 4--> </head>
The code for the menu:
<nav class="menu"> <a href="#about">About</a> <!--ES> Acerca de--> <a href="#work">My work</a> <!--ES> Trabajos--> <a href="#contact">Contact</a> <!--ES> Contacto--> <button class="darkModeSwitch" id="switch">Dark mode switch <span><i class="fa fa-sun-o"></i></span> <span><i class="fa fa-moon-o"></i></span> </nav>
If you like to use new-ish FontAwesome 5 icons, you need to have an account.
There are two ways to implement custom icons, by downloading and linking .css sheet or linking the styles to the internet. The latter we use here, we go to the FontAwesome, select ‘Start a new project with a kit’, select ‘Your Kits’, choose your kit, likely named weirdly with numbers and letters, and from there you click on the name, click on button ‘Copy Kit Code’ and that paste into your HTML code into etiquette head.
<script src="https://kit.fontawesome.com/f09689b89n.js" crossorigin="anonymous"></script> <!--FontAwesome 5-->
Now you can happily use the icons in your HTML code. So we find icons we like, and copy the icon HTML code.
Now change the icon names or paste a HTML code previously copied according to the version of icons. Later we can easily change the style of the icons with CSS, like color and size to transform it into whatever you’d like.
<span><i class="fas fa-sun"></i></span> <span><i class="fas fa-moon"></i></span>
We give it a style right away in a .css file. Cursor we want to be a pointer, that's when you hold the mouse over it, it will look like a mini hand and the rest like this. Position will be relative for having another element sitting on this.
.darkModeSwitch{ background: #3494b1; display:flex; position: relative; cursor: pointer; outline: none; border: none; border-radius: 1rem; align-items: center; justify-content: space-between; }
With this done, we want to change the style of the icons, so they are inside <span>
.darkModeSwitch span{ width:30px; height:30px; line-height:30px; display:block; color:#fff; background: none; }
So far we have something like this:
Now we have to do a small circle which will hide one or another option. We use the pseudoselector ‘After’, which will add anything behind the selector class. With property absolute we can play with the position of the circle in the box we made earlier - in which it is located(.darkModeSwitch) - by telling its position should be top,left,right: X px, we can define its starting position in the element. Top:0 and Left:0 would set the starting point or coordinate of the button to be top and left. If we set right:0 and left:unset, the button’s position would be on the right side. Later we will make it move left and right by alternating the position of the button. Then, content must be empty so that the other styles could work. Notice the transition, with this set to 0.5s the change of the colors won't be so drastic and sudden.
.darkModeSwitch::after{ display:block; background: #fffbf5; width: 2.4rem; height: 2.4rem; position: absolute; top:0; left:0; right:unset; border-radius: 3rem; transition: all .5s ease; box-shadow: 0 0 2px 2px rgba(0, 0, 0, .5); content: ""; }
So with this we have the visual part done. Now we want also the button to change the color of its background so the light mode would be more visible when the screen is very dark. When for example this button would have another class, let's call it ‘active’ to express the state of it, the button will change colors. And furthermore, when this will happen, also we change the attributes of the pseudoelement ‘after’ so it will be on the right side by overwriting values ‘left’ to unset and ‘right’ to value 0. This functionality we’d do like this:
.darkModeSwitch.active{ background: #fdd384; } .darkModeSwitch.active::after{ left:unset; right:0; }
Toggling Themes - JavaScript Part
So the rest of the work will be to actually add the class ‘active’ when we click on the button. This we’d achieve with JavaScript. Navigate yourself into the javascript file, if you don't have one, create and link it with this line in the index.html, for example right before closing etiquette of the body.
</footer> <script src="js/main.js"></script> </body>
In the JS file we put this code which will do this: We would manipulate the element called ‘switch’(remember that one in HTML code) and on the element we place the event listener - the method which expects 2 parameters. First is on what type of event should be executing the code. Second is what code should be running. This will be a function that adds or removes class with attribute ‘dark’ on the HTML element ‘body’. See the inspector in the browser and watch the body obtains our dark class upon the button is clicked. This way we would make a toggle between two states, one that has the class ‘dark’ and the one that hasn't. Also we want to do when the button is clicked, the ‘active’ class would be added to the button and by this we change the appearance of the button itself.
const switchButton = document.getElementById('switch'); switchButton.addEventListener('click', () => { document.body.classList.toggle('dark'); //toggle the HTML body the class 'dark' switchButton.classList.toggle('active');//toggle the HTML button with the id='switch' with the class 'active' });
Hello dark mode my old friend
Now we want to actually have some different color to our page. As we have set in the CSS code here, background color is set to white-ish. Now add an alternate color you pick to the class ‘dark’ of the body.
body { background: #f2f2f2; font-family: "Lato", Helvetica, sans-serif; } body.dark{ background: #5436; }
As you could see, it's fairly easy to create a website with HTML, CSS and a bit of JavaScript which has two color modes. The problem we could be facing is that with every load of the page, that is even if you hit F5 to refresh the page or you have a multi page site, the code which will be loaded will be the same every time. The result will be the user will always see the light mode at every load of the page.
What is missing to this code is some memory to the settings we choose. If we have for example implemented code for storing information like cookies or session storage, the user data would be stored in the client’s browser and at the next visit of your page it would be recognized which settings the user had and the correct display mode would be loaded. We wrote how to set up cookies last month, here is the link to our article about cookies. In the next article we will explain how to complete the dark mode with storing the user's preferences to address this problem.
Conclusion
Hopefully this article showed you a few tips you can use when you’re designing a website or web app. We explained how to create CSS code for the alternative theme and by the switching of a button it would give a totally different feel to your site. And with the benefit of more comfort for the user and that is what we all desire.
Image base from Unsplash