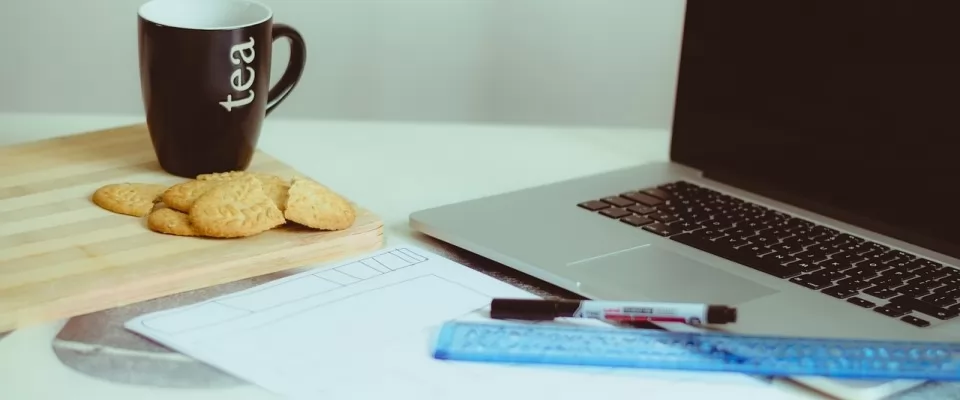
Today we are going to write about the way to store data in a browser, why websites use cookies and how they work in detail.
Continue reading to find out how to implement and manage them - if you're looking for code!
Understanding HTTP COOKIES - What are they?
Cookies and other types like local storage and session storage were invented to make your online experience easier by saving browsing information. Since web browsers and servers use HTTP protocol which is stateless, that was necessary for the website to “remember” stateful information such as logging in, buttons clicked by user, site preferences, items added into shopping cart, previously entered form fields, etc.
HTTP Cookie, also called web cookie, internet cookie, browser cookie or simply cookie is data stored on the user’s computer by the browser. They do most essential functions in modern websites, the functions were all used to, like automatic authentication - the server will request that state from the cookie. No need to require the user to get logged in every time, this is perhaps the most common and useful function of cookies.
How do cookies work?
Cookies are files created by websites, the server sends some data to the visitor’s browser, then the browser may accept the cookie. If it does, it is stored as a plain text record on the visitors computer in a folder like this for example (on windows)
C:/Users/YourUsername/Directory/App/Data/Roaming/Microsoft/Windows/Cookies, but it may be another directory too.
Later if a visitor arrives on the same server (let's say another page of the site), the browser sends the cookie back and the server retrieves cookie values.
They typically contain two bits of data. A unique ID and a site name.
Cookies are browser related, that means that they are being stored by browser used and are not available on another browser. If a user is browsing in Google Chrome, then suddenly the user switches to Firefox, they will not be used by Firefox, since any other browser will store their own cookies. Also, cookies can be perceived as temporary (session cookies) or persistent (virtually permanent cookies).
You could say temporary cookies are set to expire when the user closes the browser or leaves the site, to the opposite of persistent cookies, which remain stored on the user hard drive, until they get deleted.
Are cookies dangerous?
If you’re worried or you often say “ I'm not saying aliens, but.. ”, the best way to resolve your fears is to learn more about the topic.
Well cookies can't retrieve any other data that they have not stored on your computer, they cannot access any other files.
Cookies are files you can delete. You can also disallow cookies in your browser, but you probably do not want to, because that would really limit the quality of your Internet experience. You can set your browser to ask your permission before accepting a cookie though, and only accept them from websites you trust. Since the data in cookies doesn't change, cookies themselves aren't harmful. They can't infect computers with viruses or other malware. However, some cyberattacks can hijack cookies and enable access to your browsing sessions. The danger lies in their ability to track individuals' browsing histories.
Because persistent cookies can log your uniquely identifiable movements online over a long period, they are sometimes called tracking cookies.
Third-party tracking is frequently used by advertisers to find out what websites you visit and the content you view, as well as other information. They are set when you visit a site that contains an embedded ad from another (third-party) website. Advertisers can embed ads in a large number of sites, collate the information their cookies gather and use it to send you ads tailored to your interests.
For example European law requires that all websites targeting European Union member states gain "informed consent" from users before storing non-essential cookies on their device. That's why it all pops out on you that you almost can't see any content at first visit on the websites.
Cookie theft is also a risk to be aware of. If you sign into a site while browsing on public WiFi, as session cookies are not encrypted. A hacker could copy the cookie data and use it to impersonate you and get into your account.
Upsides and Downsides
Cookies are sent with every request, so they can worsen performance (especially for mobile data connections), so if you have a lot of cookies that are really large it'll slow down your request to and from the server. Modern APIs for client storage are the Web Storage API (localStorage and sessionStorage) and IndexedDB, which are not sent with every request.
Cookies can store only a much smaller amount of information than the other two - the capacity is 4kb, but for example authentication purposes to transport tokens it’s enough.
Local storage and session storage can hold 10 megabytes and 5 megabytes respectively.
Cookies and local storage are available for any window inside the browser - that means from different tabs, while session storage is only for a single tab.
Another advantage for cookies is that you have complete control over the expiration date of the cookie.
Downside of cookies is that it's stored in plain text, so it can be read and tampered with easily - not much suitable to store sensitive data.
Because particularly third-party tracking cookies can be used without your knowledge or permission to put together a detailed profile of you, you may consider this tracking to be an invasion of privacy. You may also object to being sent targeted adverts. Other people might find such ads delightful. These days the user or visitor of the site is usually informed about such procedures.
Cookie management in browser
Most of the browsers allow you to control your cookie settings, enable or disable cookies, see what you have stored and for how long. This of course according to the browser used.
First how to see existing stored cookies (and other storage that a web page can use) on your browser: you can enable the Storage Inspector in Developer Tools and select Cookies from the storage tree.
You can open the Storage Inspector by selecting Storage Inspector
from the Web Developer submenu in the Firefox Menu Panel /alternatively press
F12
and locate storage tab
or by pressing its
Shift + F9
keyboard shortcut (or Tools menu if you display the menu bar or are on macOS).
Let's do it and create some cookies(Taming the cookie monster)
Cookies are saved and retrieved in name-value pairs like:
name = “user”
(at least 1 parameter is required - the name of the cookie, make sense right?) -The name of your cookie. You will use this name to later retrieve it.value = “John Smith”
-The value that is stored in your cookie. Common values are username(string) and last visit(date).
There can be more fields or flags :
- Expiration - the date the cookie will expire. If this is blank, it will happen when the visitor quits the browser (session cookie).
- Domain - the domain name of the site
- Path - the path to the directory or web page that set the cookie. This may be blank if you want to retrieve the cookie from any directory or page.
- Secure - if this field contains the word secure, the cookie may only be retrieved with a secure server - it cannot be transmitted over unencrypted connections. It is made by adding the Secure flag.
- HttpOnly - with this flag, it cannot be accessed by client-side APIs.
Server side PHP Cookies
To create a cookie, use the function - setcookie()
with only the name parameter required. All other parameters are optional.
Php.net has a more detailed description.
We can store only primitive information in a cookie, not Objects or Arrays.
Important note: the setcookie function must appear before the <html>
tag.
Creating a cookie
setcookie(name, value, expiration, path, domain, security, httponly);
<?php $cookie_name = "user"; $cookie_value = "John Smith"; setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); // 86400 = 1 day ?>
With this we have created a cookie with the name “user” that holds the value “John Smith”, will expire after 30 days and is available on the entire website. If the domain would be set to '/foo/', the cookie will only be available within the /foo/ directory and all sub-directories such as /foo/bar/ of domain.
The value of the cookie is automatically URLencoded when sending the cookie, and automatically decoded when received (to prevent URLencoding, use setrawcookie()
instead. This won't accept value.
<?php $cookie_raw_name = "raw_user"; setrawcookie($cookie_raw_name, time() + (86400 * 30), "/"); ?>
To see an actual data cookie stored by Firefox browser, they are in cookie.sqlite, located in your Mozilla User Profile directory, how to open the format is above the scope of this article.
Retrieve a cookie
To find whether the cookie is set or not, use the isset() function.
To retrieve the cookie, use global variable $_COOKIE
<html><body> <?php if(!isset($_COOKIE[$cookie_name])) { echo "Cookie named '" . $cookie_name . "' is not set!"; } else { echo "Cookie '" . $cookie_name . "' is set!<br>"; echo "Value is: " . $_COOKIE[$cookie_name]; echo "<br>"; echo "Raw cookie value is " . $_COOKIE[$cookie_raw_name]; } ?> </body></html>
For the first time when we open this simple html code in a browser, we would see “Cookie named 'user' is not set! “ - that's because the first time that this code runs, there was a response from your browser which didn't contain our cookie, only after refresh (hit F5), it will retrieve it and the code will be different, it will appear “Cookie 'user' is set! Value is: John Smith ”.
We can also see the other cookie - “Raw cookie value is 1604657135“.
Modify a cookie value
To modify it, set again the cookie value. It's just that easy.
<?php $cookie_value = "Luigi"; setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); // 86400 = 1 day ?>
Delete a cookie
To delete a cookie, use the setcookie()
function with an expiration date in the past. One tip : if you had set the path, you also need to set the path when you delete the cookie, otherwise it won't function.
//DELETE COOKIE set the expiration date to one hour ago $cookie_name = "user"; $cookie_value = ""; setcookie($cookie_name, "", 1, "/");
Cookies with JavaScript
Js can manipulate cookies using cookie property of the document object.
Firstly, let's say we use the upper code to set the cookies, in HTML DOM we can obtain cookies associated with the current document by getting document.cookie
, which will return all cookies in one string much like: cookie1=value; cookie2=value; cookie3=value; like this:
<?php $cookie_name = "user"; $cookie_value = "Luigi"; setcookie($cookie_name, $cookie_value, time() + (86400 * 30), "/"); ?> <html><body> <div id="cookies">here will appear cookie data</div> </body></html> <script> var getCookies = document.cookie; document.getElementById("cookies").innerHTML = getCookies; </script>
We will get “user=Luigi”.
As we saw, if the new cookie is added to document.cookie, older cookies are not overwritten, instead the new cookie is added to the end, so if you read document.cookie, you get a chain of strings like “username=John Doe; username=Santa”.
If you want to find the value of one specified cookie, you must write a JavaScript function that searches for the cookie value in the cookie string. There is no easy way to show only specific cookie, but all of them at once.
Creating a cookie with Javascript
The simplest way to create a cookie is to assign a string value to the document.cookie object. Multiple parameters are separated by a semicolon (;)
<html><body> <div id="cookies">here will appear cookie data</div> </body></html> <script> document.cookie = "site = Ma-no.org" var getCookies = document.cookie; document.getElementById("cookies").innerHTML = getCookies; </script>
We will get “site=Ma-no.org;”
Now we add expiry time because we don't want the cookie to be deleted when we close the browser. It needs to be in format UTC.
And with the path parameter, we can set the path the cookie will function. By setting “/” we will tell that cookie will be available to the whole site.
document.cookie = "username = Santa; expires=Thu, 24 Dec 2020 12:00:00 UTC; path=/";
Expiration dates can be set directly or we can use JS date function with UTC string methods on them.
var date=new Date() date.setFullYear(2021,0,1) date.setHours(0 +1) //since we are in GMT+1 zone date.setMinutes(0) date.setSeconds(0) document.cookie = "site = Ma-no.org; expires=" + date.toUTCString();
This cookie now expires at Fri Jan 01 2021 01:00:00 GMT+0100 (Central European Standard Time).
If we want a cookie that never expires, we must set the date much in the future, say 200years.
When an Expires date is set, the time and date set is relative to the client the cookie is being set on, not the server.
Here is an example of a cookie that will expire in 2 days.
var cookieDate = new Date() var expiration_days = 2 cookieDate.setTime(cookieDate.getTime() + (expiration_days*24*60*60*1000)) document.cookie = "username = John Doe; expires=" + cookieDate.toUTCString()
The value of a cookie cannot contain commas, semicolons or whitespaces. If you need to add those characters for some reason, you can use the encodeURIComponent() method, which will encode those characters to a writable format.
This function encodes special characters. In addition, it encodes the following characters: , / ? : @ & = + $ #
document.cookie = "obľúbení = Čert, Vločka a Pán prsteňov"
This can make unpredictable things as you can see here, this cookie is handled as an object :
And now we will obtain the specific cookie with the help of this function, which decodes the cookie string, to handle cookies with special characters. Here you can see the difference in encoding.
<div id="cookies">here will appear cookie data</div> <div id="decodedCookie">decoded data</div> </body></html> <script> document.cookie = "obľúbení = " + encodeURIComponent("Čert, Vločka a Pán prsteňov") var getAllCookies = document.cookie document.getElementById("cookies").innerHTML = getAllCookies document.getElementById("decodedCookie").innerHTML = getCookie('obľúbení'); //returns the value of a specified cookie function getCookie(cookieName) { var nameString = cookieName + "="; var decodedCookie = decodeURIComponent(document.cookie); var cookieArray = decodedCookie.split(';'); //Split document.cookie on semicolons into an array for(var i = 0; i <cookieArray.length; i++) { //Loop through the array var cookie = cookieArray[i]; while (cookie.charAt(0) == ' ') { cookie = cookie.substring(1); //Read out each value } if (cookie.indexOf(nameString) == 0) { //If the cookie is found, return the value of the cookie return cookie.substring(nameString.length, cookie.length); } } return ""; //If the cookie is not found, return nothing. } </script>
Delete a cookie with Javascript
To delete a cookie, set expires parameter to a passed date:
document.cookie = "username = Santa; expires=Tue, 6 Oct 2020 12:00:00 UTC; path=/"
Some browsers will not let you delete a cookie if you don't specify the path.
Conclusion
Cookies serve mainly for three purposes - Session management, Personalization and Track user behaviour.
There are some alternatives to the cookies already for some time but it seems that cookies are here to stay. If you want to know more about cookies, there's plenty of sources out there.
With cookies, sites can:
- Keep you signed in.
- Remember your site preferences.
- Give you locally relevant content.
And let's face it. They have a cool name.
Image sources:
Difference img from teacher Beau Carnes from freecodecamp
Royalty-Free photo: Broken white ceramic bowl with brown cookies | PickPik
Sally makes her own cookie monster cupcakes | Food | Jose Martinez | Flickr