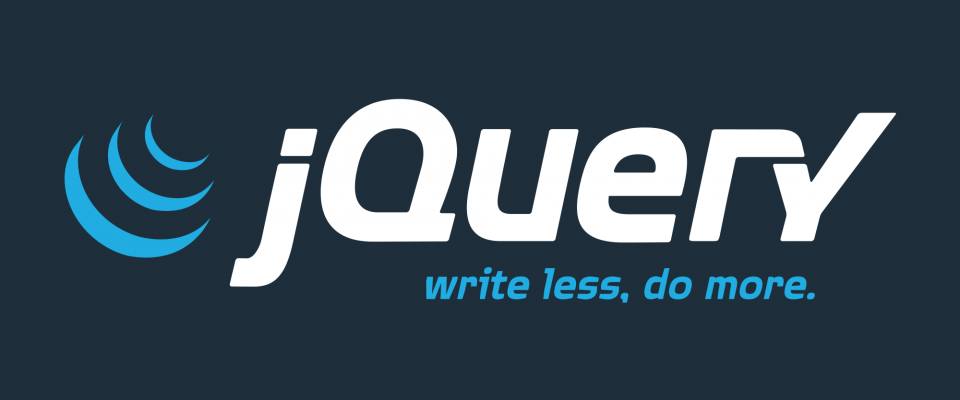
When you have to manage a web project, jQuery can be of great help. In this article, we have compiled some jQuery tips and tricks for making and enhancing responsive sites.
Detect viewport size
CSS media-queries allow you to detect the viewport size and apply different CSS style to elements depending on the viewport width.
This can also be done in jQuery, and it can be very useful to achieve results you couldn’t using CSS alone. The following example shows how to detect the viewport width, and subsequently add an element to a list.
if ($(window).width() < 960) { $( "ul.mylist").append("<li>One more list element</li>"); }
Source: CSS Tricks
Animate Height/Width to “Auto”
If you have tried using thing.animate({ "height": "auto" }); on an element, you've noticed that it didn't work. Happily, there's a quick and efficient fix to this problem.
Here's the function:
jQuery.fn.animateAuto = function(prop, speed, callback){ var elem, height, width;return this.each(function(i, el){ el = jQuery(el), elem = el.clone().css({"height":"auto","width":"auto"}).appendTo("body"); height = elem.css("height"), width = elem.css("width"), elem.remove(); if(prop === "height") el.animate({"height":height}, speed, callback); else if(prop === "width") el.animate({"width":width}, speed, callback);else if(prop === "both") el.animate({"width":width,"height":height}, speed, callback); });
And how to use it:
$(".animateHeight").bind("click", function(e){$(".test").animateAuto("height", 1000); }); $(".animateWidth").bind("click", function(e){$(".test").animateAuto("width", 1000); }); $(".animateBoth").bind("click", function(e){$(".test").animateAuto("both", 1000); });
Source: CSS Tricks
Scroll to an element
Scrolling endlessly on a smartphone isn’t the most fun thing ever. This is why it can be very useful to set scrolls so your visitors won’t need to take 10 seconds to reach the info they’re looking for.
Let start with an auto-scroll: The code below will programmatically scroll to a specific element on the page:
$('html, body').animate({scrollTop: $("#target").offset().top }, 1000);
Now, let’s set a scroll that the user will activate by clicking on a link:
$('a#source').on('click', function(event) {$('body').scrollTo('#target'); });
Lazy load images
Lazy loading is a technique that forces a page to only load images which are visible on the clients' screen. It has proven to be very effective for improving your website loading speed, which is extremely important in terms of user experience and SEO.
There are lots of jQuery plugins dedicated to implement lazy loading on your site. If you're using WordPress, I definitely recommend this one.
As for jQuery lazyload plugins, I've been using this one, simply called Lazy Load, on a few sites. It's usage is very easy. The first step is to import the plugin into your HTML page:
<script src="https://cdn.jsdelivr.net/npm/[email protected]/lazyload.js"></script>
Now the HTML code: By default Lazy Load assumes the URL of the original high resolution image can be found in data-src attribute. You can also include an optional low resolution placeholder in the srcattribute.
<img class="lazyload" data-src="img/example.jpg" width="765" height="574"> <img class="lazyload" src="img/example-thumb.jpg" data-src="img/example.jpg" width="765" height="574">
It's now time to activate the lazy loading. The following will lazy load all images with the .lazyload class:
lazyload();
Turn navigation menu into a dropdown
When your website features many menu items, it can be tricky to display on small screens. Therefore, an easy fix to this problem is to turn a navigation into a dropdown menu.
The code below will get items from nav and append them to a select dropdown:
// Create the dropdown base $("<select />").appendTo("nav"); // Create default option "Go to..." $("<option />", { "selected": "selected","value" : "","text" : "Go to..." }).appendTo("nav select"); // Populate dropdown with menu items $("nav a").each(function() { var el = $(this); $("<option />", { "value" : el.attr("href"), "text" : el.text() }).appendTo("nav select"); }); $("nav select").change(function() { window.location = $(this).find("option:selected").val(); });
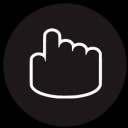
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
JavaScript Formatting Date Tips
Something that seems simple as the treatment of dates can become complex if we don't take into account how to treat them when presenting them to the user. That is…
A Java approach: While loop
Hello everyone and welcome back! After having made a short, but full-bodied, introduction about cycles, today we are finally going to see the first implementations that use what we have called…
A Java approach: The Cycles - Introduction
Hello everyone and welcome back! Until now, we have been talking about variables and selection structures, going to consider some of the fundamental aspects of these two concepts. Theoretically, to…
Javascript: what are callbacks and how to use them.
Today we are going to learn about a concept that is widely used in javascript and that is used quite a lot by today's frameworks, libraries, especially NodeJS. This is…
TypeScript: The evolution of JavaScript
When you're involved in the development of a large project, programming languages like JavaScript don't seem to be the best solution. Their lack of elements such as Language Aids has…
JavaScript. What's new in ES2020?
As we discussed in our article about the ES2019 features you should try, ECMAScript's proposals will continue to grow and give rise to new implementations. Therefore, you can already access the…
Vanilla JavaScript equivalent commands to JQuery
JQuery is still a useful and pragmatic library, but chances are increasingly that you’re not dependent on using it in your projects to accomplish basic tasks like selecting elements, styling…
What's new in Angular?
In version 8.0.3 of Angular we can find great changes and say goodbye to our beloved companion the home page of Angular which has been re-designed with a fresh attractive…
React: 4 types of components to rule them all
If you have already worked with React, you will probably have found terms like "dumb components", "stateless components" or "high order components" which serve to describe different ways of defining…
Is jQuery going to die in 2019?
For a while, JQuery's relevance has been a topic of debate among web developers. We were curious as web developers interested in Javascript to know what others have to say…
Best 5 Javascript NewsTicker Plugins
Not all developers know the marquee tag of HTML, that allows you to create a scrolling piece of text or image displayed that is shown horizontally or vertically on the DOM.…
A roadmap to becoming a web developer in 2019
There are plenty of tutorials online, which won't cost you a cent. If you are sufficiently self-driven and interested, you have no difficulty training yourself. The point to learn coding…