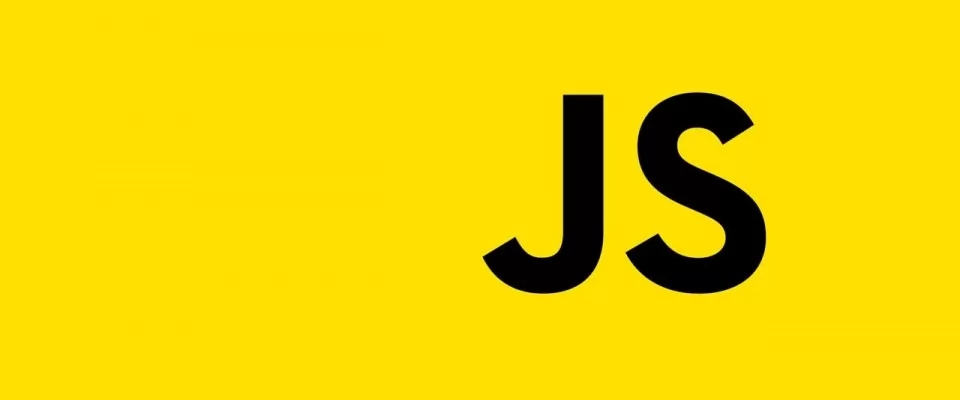
Primitive and referenced values (objects) behave very differently when we want to make a copy of them.
Primitive values
Let's suppose a variable name that has a primitive value associated with it (number, string, boolean, undefined and null). If we make a copy of this variable name to another variable name2, any modification in the original variable will not imply any change in the second variable as they are primitive values.
let name="Silvia"; let name2=""; console.log (name, name2); // returns Silvia, Silvia name="Luigi"; console.log (name,name2); // returns Luigi, Silvia
Example 1
Values by reference
However, if we do the same operation on values by reference, any change we make in one of the variables will also be reflected in the other variable since both variables point to the same object. (see example 2)
Arrays
To clone arrays, the slice()
method is used to create a new copy of the array. This copy can be modified independently without affecting the original array (n3 example 2). If no parameters are passed, it makes an exact copy of the array, but numbers can also be passed as parameters. If only one number is passed, it will determine the value of the index from which we want the copy to be made, while if 2 numbers are passed, we will be marking the beginning and the end.
//n.1 const names= ['Silvia','Luigi','Alex']; const names2= names; console.log (names, names2); // returns name ['Silvia','Luigi','Alex'] // returns names2 ['Silvia','Luigi','Alex'] //n.2 names2[2]="Angelo"; console.log (names,names2); // returns name ['Silvia','Luigi','Angelo'] // returns names2 ['Silvia','Luigi','Angelo'] //n.3 const name2= names.slice(); names2[2]="Anna"; console.log (name2,names2); // returns name ['Silvia','Luigi','Angelo'] // returns names2 ['Silvia','Luigi','Anna']
Example 2
Objects
The same happens when the referenced value is an object, any modification in one of its properties will affect both variables (n.2 Example 3). To clone objects, the Object.assign() method is used, which will copy the values of all enumerable properties of one or more source objects to a target object (n.3 Example 3). But this method is only effective for shallow copies of objects, i.e. when our starting object has no other objects as attributes.
//n.1 const names= { name:'Silvia', surname:'White' } const names2= names; console.log (names, names2); // they are exact copies //n.2 names2.surname='Green'; console.log (names,names2); /* returns const names= { name:'Silvia' surname:'Green' } returns const names2= { name:'Silvia'; surname:'Green'; } */ //n.3 const name2= Object.assign({}, names); names2.surname="Green"; console.log (names,names2); /* returns const names= { name:'Silvia' surname:'White' } returns const names2= { name:'Silvia' surname:'Green' } */
Example 3: Cloning of objects at first level
To make a deep copy of objects other methods are used.
As we said, the Object.assign()
method was only valid when we were trying to make a shallow copy of the object, that is, when our object does not have other objects as attributes. In these cases, a deep copy of the object is necessary.
A deep copy, unlike a shallow copy, also copies each sub-object recursively until all the objects involved are copied.
What methods can we use for a deep copy of Objects?
JSON.parse(JSON.stringify(obj))
This method converts the object to a string with JSON.stringify() and then converts it back to a JSON.parse() object. This method is effective with simple objects but not when one of the properties is a function (Example 4).
//n.1 const names= { name:'Silvia', surname:'White', social: { twitter: '@manoweb', facebook:'ManoWeb' } } const names2= JSON.parse(JSON.stringify(names)); names2.social.facebook='manoagency'; console.log (names, names2); /* returns const names= { name:'Silvia', surname:'White', social:{ twitter:@manoweb facebook:'ManoWeb' } } returns const names2= { name:'Silvia', surname:'White', social:{ twitter:@manoweb facebook:'manoagency' } } */
Example 4
Exploiting recursive functions in Deep Clone
Another very interesting and elegant way to make deep copies of objects is to use recursive functions (Example 5).
We create a function deepClone(object)
to which we pass as an argument the object we want to clone.
Inside the function, a local variable clone is created, which is an empty object where each of the properties that will be cloned from the starting object will be added.
Thanks to a for
we go through each and every one of the properties of the object we want to clone.
- If the property is not an object, it is simply cloned and added to the new clone object.
- If the attribute is an object, the deepClone(value) function is executed again, passing the value of the property (in this case the object) as a parameter, and the same process is repeated.
function deepClone(object) { var clone={}; for (var key in object) { var value = object[key]; if (typeof(value)!='object') { clone[key]=value; } else { clone[key]=deepClone(value);//Here the object property is also an object. //By means of a recursive function, what we do is to clone the object that //is on the second or next level. } } return clone; } deepClone({value1:1,value2:{value3:2}}); //{value1:1,value2:{value3:2}} deepClone({value1:1,value2:{value3:{value3b:3}}}); //{value1:1,value2:{value3:{value3b:3}}}
Example 5
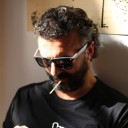
Luigi Nori
He has been working on the Internet since 1994 (practically a mummy), specializing in Web technologies makes his customers happy by juggling large scale and high availability applications, php and js frameworks, web design, data exchange, security, e-commerce, database and server administration, ethical hacking. He happily lives with @salvietta150x40, in his (little) free time he tries to tame a little wild dwarf with a passion for stars.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…