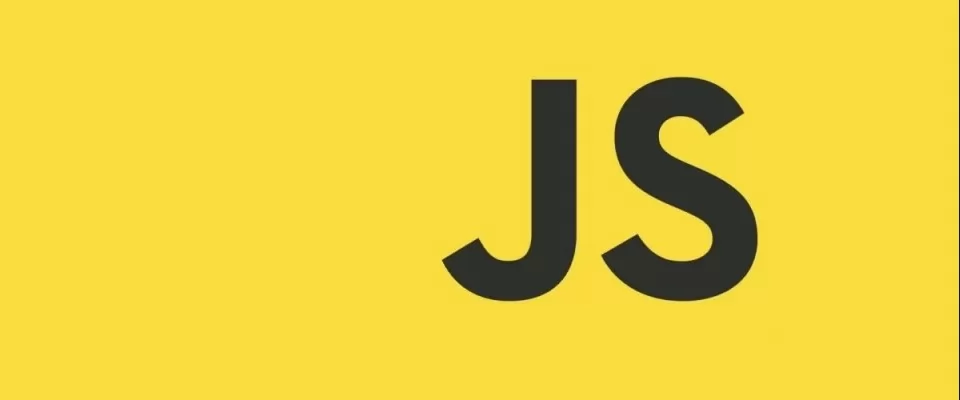
We were already talking about how to multiply arrays in JavaScript and we realised that we had not explained something as simple as traversing an array with Javascript. So we couldn't let the time pass and we have started working to explain you how to do this task.
The first thing to do is to define the array in Javascript. To do this we instantiate it directly on a constant.
const matrix = [[1,2,3],[4,5,6],[7,8,9]];
Here we see that the elements of the array are themselves arrays of elements, which ends up forming the matrix.
Being an array of arrays, we will need two for loops to traverse the matrix. The first one will traverse the main array and the second one will traverse each of the secondary arrays. The arrays will go from the initial position to the length of the array, which we get through the .length
property.
In this way the two concatenated loops are as follows:
for (x=0;x<matrix.length;x++) { for (y=0;y<matrix[x].length;y++) { // Acceder al elemento } }
Inside the second loop, we can access the element via the x and y variables of the matrix.
for (x=0;x<matrix.length;x++) { for (y=0;y<matrix[x].length;y++) { console.log(matrix[x][y]); } }
This code returns the list of the elements we have traversed in the matrix. So, in order not to leave it without formatting, we are going to add a little bit of formatting in the generation of the array information. The final code will look like this:
for (x=0;x<matrix.length;x++) { text = "" for (y=0;y<matrix[x].length;y++) { text += matrix[x][y] + "t"; } console.log(text) }
I hope you found useful this simple code that explains how to traverse a matrix with JavaScript.
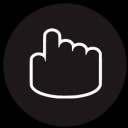
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…