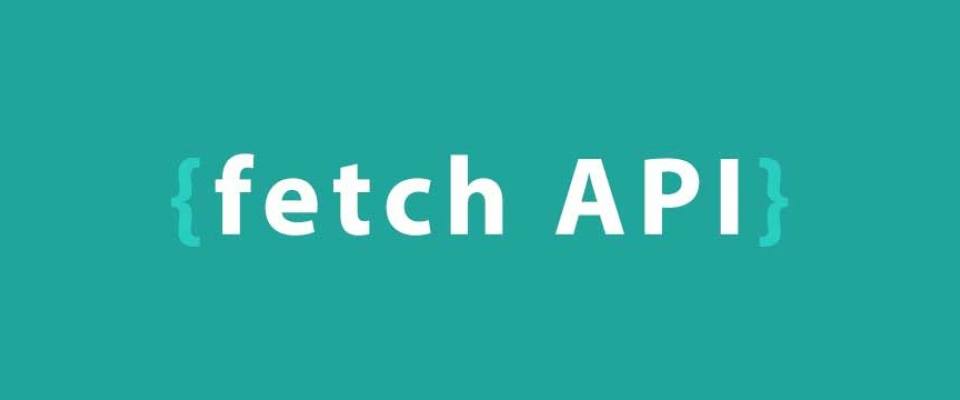
When AJAX came to the modern web, it changed the definition of how web works. We all are using ajax for a long time but not with Fetch API. To load a new content in a web page, we do not need a full page reload. Using AJAX, we can post or pull data from a web server asynchronously.
Almost every web application nowadays use ajax. It was all possible because of the XMLHttpRequest. It is a browser API in the form of an object whose methods transfer data between a web browser and a web server. The object is provided by the browser’s JavaScript environment.
Our one of the most popular JavaScript libraries, jQuery uses XMLHttpRequest to make HTTP calls. If we talk about any networking library in any modern JavaScript ecosystem uses XMLHttpRequest like axios (promise-based), superagent (callback-based).
The issues with XMLHttpRequest
Making HTTP request with raw XMLHttpRequest really sucks. You need to take care a lot of things to get some data from the server. To make a get request with XMLHttpRequest, we need to write the code like
var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (xhr.readyState == XMLHttpRequest.DONE) { alert(xhr.responseText); } } xhr.open('GET', 'http://example.com', true); xhr.send(null);
We tend to use jQuery’s Ajax (84 KB). If in a web application, we are adding jQuery to make only a few requests, then It is not at all bandwidth friendly. We are loading a script of 84kb to use only one or two methods. The problem can be solved using the Fetch API which is already present in our browser.
Introduction to Fetch API
Fetch is a promise based HTTP networking API provided by the browser. It is here from the chrome 42. Firefox also supports it. It is now almost standardised. It saves you from remembering complex API of XMLHttpRequest. Look at one example of Fetch.
fetch('some/api/getData.json') .then( function(response) { if (response.status !== 200) { console.log('Status Code: ' + response.status); return; } response.json().then(function(data) { console.log(data); }); } ) .catch(function(err) { console.log(err); });
It is clean. It is excellent. Here we are making a simple get request. Fetch always returns a promise. Promise resolves to the response object. This response object has different helper methods like response.json(), response.text(), response.blob() etc.
Usage of Fetch
It can fully replace old XMLHttpRequest. The first parameter of the fetch is the requested URL. We can also pass a second parameter which is essentially a configuration object. In this configuration object, we can specify different options like HTTP Method Type, Headers of the Request, body of the request etc. Let’s look at how to make a post request with Fetch.
Post Request using Fetch API
function json(response) { return response.json() } var url = '/api/saveData'; fetch(url, { method: 'post', headers: { "Content-type": "application/x-www-form-urlencoded" }, body: 'foo=bar&lorem=ipsum&[email protected]' }) .then(json) .then(function (data) { console.log(data); }) .catch(function (error) { console.log('Failed', error); });
Here we are chaining our response through our json method. The code is self-explanatory.
If you are yet not convinced to use Fetch. There is an excellent fetch polyfill library that you can try.
We will encourage you to use fetch over XMLHttpRequest in your next vanilla JavaScript project.
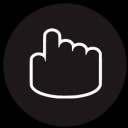
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
Why businesses need to be familiar with APIs
APIs serve as intermediaries between software, allowing them to communicate with each other and perform various functions like data sharing or processing. APIs provide the protocols, definitions, tools, and other…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
