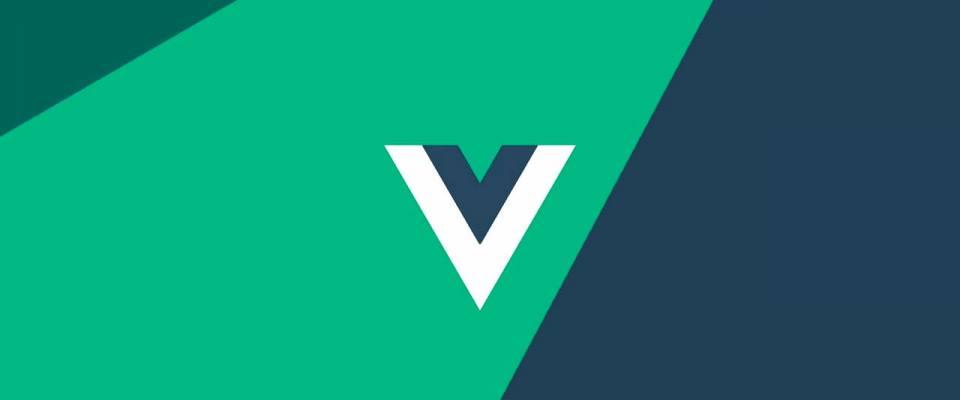
Vue.js seems to be another JavaScript UI library with a strong community support. If you've got a project coming up and you're wondering which tool to choose from, you can try Vue. View is very easy to learn and in just 10 minutes you can get started with it (actually that's what this article will help you do).
But first let's look at why you should even try to give Vue a quick look.
Why use Vue?
The idea behind this post is not to try to persuade you whether or not to use Vue. Nor should it start an argument about whether it's better than other tools out there or not. Rather, the purpose is to show you how easy and simple it is to get started with Vue.js and perhaps it could save a deadline for you.
When compared to the alternatives, including Angular, React, Ember, Aurelia, etc., Vue brags in some aspects of having beaten some of them. Simple API, size, overall performance, curve of learning, etc. On the official documentation., you can look at these comparisons.
But we already know, that these tools are not the most important of all — it's just about you, your customer, your team, and the project. Choosing what blends in well with all those factors is recommended, and if Vue does, read on!
Requirements
View requires to experience its awesomeness as little as a single script. And we can use Fiddle for this tutorial and we don't need to procure the priviledge of tooling to keep building on our machine. This will also help us to instantly see some of our code coming to life.
Simply create an empty Fiddle and add to the HTML the following script:
<script src="https://unpkg.com/vue@next/dist/vue.js"> </script>
The script loads Vue into the Fiddle ecosystem and we can use whatever Fiddle API can offer .
The Vue Instance
Each Vue application's entry point is created through an instance. Then the instance configures the rest of the application and can drill into children as your application scales up. That's how it looks like:
// The Vue instance new Vue({ // Element to attach the app // on the DOM el: '#myapp', // Bindable data data: { message: 'Hello World! Welcome to Ma-No', } })
The instance is coded by passing information about the app into an object and where it should be loaded. The el
property explicitly states which element to mount on and the value is the ID of the element prefixed with a hash (#).
The data to be linked to the view is clearly stated with the data property, which also has a value object that can be accessed through the template.
Back to our HTML, using what is called reactive binding, we can display the data:
<div id="myapp"> <p>{{ message }}</p> </div>
Two things that are worth mentioning are :
- Where we mount the application (the ID of our DIV)
- The double curly braces are used to bind the data to the template.
We bind the message value specified during configuration in the data object. It's really simple to get started and we already have a working app:
Data-Binding
We've already seen a clear example of binding data to view, however in a real application there's more to it. Let's start drilling it further and see what Vue can do for us:
The Conditions
The ability to bind data to view before a decision is a very useful feature in JS frameworks. Only if a value resolves to true can we tell Vue to make a binding. Let's start to look how:
new Vue({ el: '#myapp', data: { message: 'Hello World! Welcome to Ma-No', //We check if a user is a new User or not newUser: true } })
We just added additional data to check whether or not the user is new. If the user has just entered, he / she will not be a new User. Use the flag to display various messages to users of Ma-No:
<div id="myapp"> <!-- Display new user--> <h2 v-if="newUser">{{ message }}</h2> <!-- Display old user --> <h2 v-else="newUser">Welcome back! Choose a tutor</h2> </div>
v - if
and v - else
are liable for displaying data. If the value being examined (which is newUser) resolves to be true, the v - if
directive template wins, if false, v - else
wins.
The Loops
Vue is amazing enough to provide us with a simple API for looping through a range of bound data. For this purpose, the v - for
directive takes its use and all we need is a set of data:
<ol> <li v-for="tutor in tutors">{{tutor.fullname}}</li> </ol>
V - for embraces a loop expression that generates a tutor variable and utilizes the variable to iterate over a tutor array. The array actually contains the object so that the properties can then be accessed:
new Vue({ el: '#myapp', data: { message: 'Hello World! Welcome to Ma-No', newUser: false, // Array of tutors tutors: [ {id: "ABC", fullname: "Mario Rossi"}, {id: "DEF", fullname: "Luigi Bianchi"}, {id: "GHI", fullname: "Joana Doe"}, {id: "JKL", fullname: "Paul Blu"}, {id: "MNO", fullname: "Mary White"}, {id: "PQR", fullname: "Sam Green"} ] } })
You can notice the array that contains a list of tutors which the v-for directive is iterating over.
Two-Way Binding
Two - way binding in Vue is quite straight forward and can be accomplished with a v - model directive. By attaching the v - model directive to a text input and displaying the data at the same time, let's see two - way binding in play:
<p> Add tutor: <input type="text" v-model="tutor"> {{tutor}} </p>
The directive's value is used for data binding, and that is why the interpolation content ({{}}) is the same as the directive's. In our Vue configuration, we now need to define the data:
new Vue({ el: '#myapp', data: { message: ''Hello World! Welcome to Ma-No!', // For the two-way binding tutor: '', newUser: false, tutors: [ // Truncated for brevity ] } })
Events and Handlers
Event is the narrative behind any web application's user interaction. Because of that, for generating and handling events, Vue has a very declarative syntax that is easy to understand and reUser.
Let's add more controls to our view to collect the full name and ID of the tutor.
<p> ID: <input type="text" v-model="tutor.id"> {{tutor.id}} </p> <p> Fullname: <input type="text" v-model="tutor.fullname"> {{tutor.fullname}} </p> <p> <button v-on:click="addtutor">Add tutor</button> </p>
It's just a simple binding, however this time we bind to an object's values and not just a primitive direct value.
We also added a button to an event as well. Using the v-on directive, events in Vue are created while attaching the type of event using:. Eg: v-on: click, v-on:mouseover, etc. The click event takes a handler, addTutor that we still have to implement. Before this event is implemented, update the Vue config Tutor data to an object instead of a primitive:
tutor: {id:'',fullname:''},
Event handlers are specified in the same way as bound data, but not directly within the data object, but in a methods object:
new Vue({ el: '#myapp', data:{ message: 'Hello World! Welcome to Ma-No!', // No longer a primitive tutor:{id:'',fullname:''}, newUser: false, tutors:[ // Truncated for brevity]}, // Methods methods:{ // Add tutor handler addtutor: function(){ // Add new value from the inputs // above the existing array this.tutors.unshift(this.tutor)}}})
The function value of the addtutor property tells our app what to do when clicking on the button. All it does is update the tutor array by dropping our new tutor over the array with unshift
Meet Components
You may stop worrying because you can can enjoy components like you are doing in other frameworks or libraries. Components are recommended in Vue projects, because we can already see that our app is expanding.
Let us build a tutor-user component that only gets data from our application and shows the information:
// tutor-user component Vue.component('tutor-user', { // acceptable props props: ['tutor'], // renderable templates template: '<li>{{tutor.fullname}}</li>' });
Components are built with a Vue.component that takes two arguments : name and configuration. The object config establishes the template that the component will inject to the DOM and the props expected from the parent of the component. The parent himself is that, so let's see how the parent calls it:
<ol> <tutor-user v-for="tutor in tutors" v-bind:tutor="tutor"></tutor-user> </ol>
Using the identifier specified during component creation, we render the component. Using v - bind, we also pass in the props:[PROP NAME ] where PROP NAME is the property name and mentor in our case. The property's value is obtained from the loop.
This may not be useful for our application, but once an app has scaled, more and more DOM items may be necessary for the part taken care of by the component.
Conclusions
As you saw, starting with Vue is very easy and Vue actually boasts simplicity. Vue looked at other frameworks ' disadvantages and tried to improve things that make it worthy of being shot or even considered.
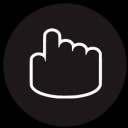
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…
