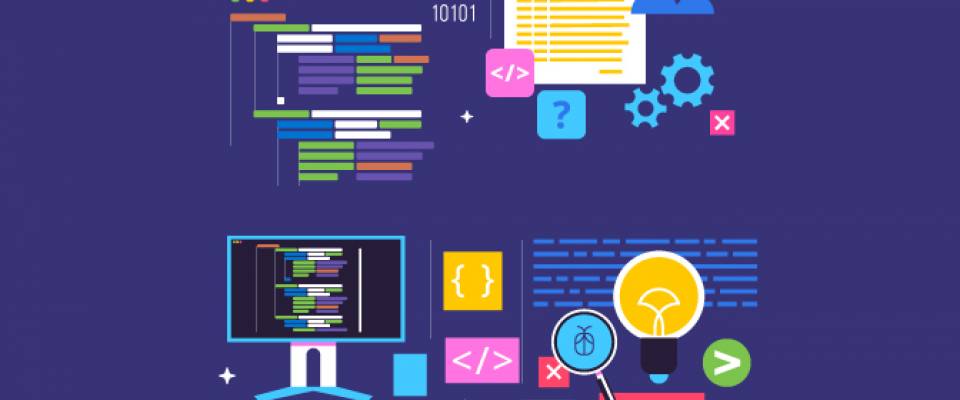
For anyone who is not already acquainted with it, Google provides a style guide for writing JavaScript that gives the best stylistic practices for writing clean, understandable code (what Google believes to be).
The guide does not present difficult rules for writing valid JavaScript, but only tips to keep the source files consistent and attractive.
This is extremely interesting for JavaScript, a flexible language that enables a wide range of stylistic choices.
Two of the most popular style guides are currently available from Google and Airbnb. If you spend a lot of time writing JS, we definitely recommend that you check it out.
The following is an analysis of the points of the Google JS Style Guide that we consider to be fundamental
They deal with everything from heavily contested issues (tabs versus spaces and the controversial question of how to use semicolons) to some more arcane specifications that impressed us.
We will provide a summary of the specification for each rule, followed by a supporting quote from the style guide that details the rule. We will also give an example of the style in practice where applicable and contrast it with code that does not follow the rules.
Use spaces, not tabs
The ASCII horizontal space character (0x20) is the only whitespace character to appear anywhere in a source file, apart from the line terminator sequence. This means that the characters on the tab are not used for indentation.
The guide indicates that two spaces (not four) should be used for indentation.
// bad function foo() { ∙∙∙∙let name; } // bad function bar() { ∙let name; } // good function baz() { ∙∙let name; }
Semicolons ARE required
Every statement must be terminated with a semicolon. Relying on automatic semicolon insertion is forbidden.
While we can't imagine why anyone opposes this idea, the consistent use of semicolons in JS is becoming a new debate about 'spaces versus tabs.' In defense of the semicolon, Google is coming out firmly here.
// bad let luke = {} let leia = {} [luke, leia].forEach(jedi => jedi.father = 'vader') // good let luke = {}; let leia = {}; [luke, leia].forEach((jedi) => { jedi.father = 'vader'; });
Don’t use ES6 modules (yet)
Do not use ES6 modules yet (i.e. the export and importkeywords), as their semantics are not yet finalized. Note that this policy will be revisited once the semantics are fully-standard.
// Don't do this kind of thing yet: //------ lib.js ------ export function square(x) { return x * x; } export function diag(x, y) { return sqrt(square(x) + square(y)); } //------ main.js ------ import { square, diag } from 'lib';
Horizontal alignment is discouraged (but not forbidden)
This practice is allowed, but Google Style generally discourages it. Horizontal alignment in places where it has already been used is not even required.
Horizontal alignment is the practice of adding a variable number of additional spaces in your code to make certain tokens appear on previous lines directly below certain other tokens.
// bad { small: 42, big: 435, }; // good { small 42, big: 435, };
Don’t use var anymore
With either const or let, declare all local variables. Unless a variable needs to be reassigned, use const by default. The keyword of var should not be used.
We still see people on StackOverflow and elsewhere using var in code samples. If people argue for it, or if it's just a case of old habits dying hard, we can't say.
// bad var example = 42; // good let example = 42;
Arrow functions are preferred
Arrow functions provide a concise syntax and resolve a number of problems. Prefer arrow functions over the keyword function, especially for nested functions
We'll be honest, we just thought arrow functions were great because they were more concise and more pretty to look at. It also turns out that they serve a very useful function.
// bad [1, 2, 3].map(function (x) { const y = x + 1; return x * y; }); // good [1, 2, 3].map((x) => { const y = x + 1; return x * y; });
Use template strings instead of concatenation
Use template strings (delimited with `) over complex string concatenation, particularly if multiple string literals are involved. Template strings may span multiple lines.
// bad function sayHi(name) { return 'How are you, ' + name + '?'; } // bad function sayHi(name) { return ['How are you, ', name, '?'].join(); } // bad function sayHi(name) { return `How are you, ${ name }?`; } // good function sayHi(name) { return `How are you, ${name}?`; }
Don’t use line continuations for long strings
Do not use line continuations in ordinary or template string literals (i.e. ending a line in a string literal with a backslash). Although ES5 allows this, if any trailing whitespace comes after the slash, it can lead to difficult errors and is less obvious to readers.
Interestingly enough, Google and Airbnb disagree with this rule (the spec of Airbnb is here).
While Google suggests connecting longer strings (as appeared as follows) Airbnb's style control prescribes basically doing nothing, and enabling long strings to go on as long as they have to.
// bad (sorry, this doesn't show up well on mobile) const longString = 'This is a very long string that \ far exceeds the 80 column limit. It unfortunately \ contains long stretches of spaces due to how the \ continued lines are indented.'; // good const longString = 'This is a very long string that ' + 'far exceeds the 80 column limit. It does not contain ' + 'long stretches of spaces since the concatenated ' + 'strings are cleaner.';
“for… of” is the preferred type of ‘for loop’
With ES6, the language now has three different kinds of forloops. All may be used, though for-of loops should be preferred when possible.
If you ask us, this is a strange one, but we thought we'd include it because Google considers a preferred type of for loop.
We've always been under the impression that for... in loops were better for objects, while for... of were better suited to arrays. A ‘right tool for the right job’ type situation.
While Google's detail here doesn't really negate that thought, it is as yet intriguing to realize they have an inclination specifically for this loop.
Don’t use eval()
Do not use eval or the Function(...string) constructor (except for code loaders). These features are potentially dangerous and simply do not work in CSP environments.
The MDN page for eval() has a section called “Don’t use eval!”
// bad let obj = { a: 20, b: 30 }; let propName = getPropName(); // returns "a" or "b" eval( 'var result = obj.' + propName ); // good let obj = { a: 20, b: 30 }; let propName = getPropName(); // returns "a" or "b" let result = obj[ propName ]; // obj[ "a" ] is the same as obj.a
Constants should be named in ALL_UPPERCASE separated by underscores
Constant names use CONSTANT_CASE: all uppercase letters, with words separated by underscores.
In case you're certain beyond a shadow of a doubt that a variable shouldn't change, you can demonstrate this by capitalizing the name of the constant. This makes the constant’s immutability obvious as it gets used throughout your code.
If the constant is function - scoped, it provoques a notable exception to this rule. It must be written in camelCase in this case.
// bad const number = 5; // good const NUMBER = 5;
One variable per declaration
Every local variable declaration declares only one variable: declarations such as let
// bad let a = 1, b = 2, c = 3; // good let a = 1; let b = 2; let c = 3;
Use single quotes, not double quotes
Ordinary string literals are delimited with single quotes ('), rather than double quotes (").
Tip: if a string contains a single quote character, consider using a template string to avoid having to escape the quote.
// bad let directive = "No identification of self or mission." // bad let saying = 'Say it ain\u0027t so.'; // good let directive = 'No identification of self or mission.'; // good let saying = `Say it ain't so`;
Conclusions
These are not directives, as we said at the beginning.
Google is one of the many technology giants, and these are only guidelines. All of that said, of it's fascinating to look at a company like Google's which employ a lot of brilliant developers who spend a lot of time writing great code, giving style recommendations.
If you want you can follow the "Google Compliant Source Code" guidelines", but, of course, several people disagree, and you can brush off any or all of this.
We definitely think there are several cases where the specifications of Airbnb are more attractive than those of Google. Regardless of the position you take on these specific rules, it is still important to bear in mind the stylistic consistency when writing any code.
Business vector created by freepik - www.freepik.com
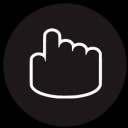
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Template Literals in JavaScript
Template literals, also known as template literals, appeared in JavaScript in its ES6 version, providing a new method of declaring strings using inverted quotes, offering several new and improved possibilities. About…
How to use the endsWith method in JavaScript
In this short tutorial, we are going to see what the endsWith method, introduced in JavaScript ES6, is and how it is used with strings in JavaScript. The endsWith method is…