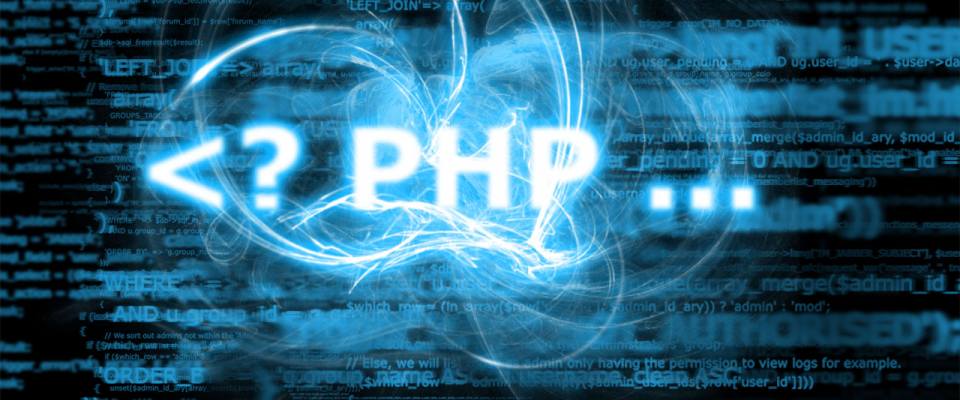
If you are familiar with th MVC language before you certainly know the practice of separating your business logic from your HTML.
The way this works in MVC language is that the framework will allow you to assign a view (HTML) to a controller (class).
In this tutorial we are going to re-create this functionality and send variables that can be used in a HTML file. We will also be able to return the contents of that HTML inside a variable to output this in any place we want within our application.
To achieve this we will use the PHP output controller functions.
The "View File"
A typical view file content just the HTML for the page without any logic part. All the logic in your application should be done in the controller and this will be sent to the view.
Below is a simple example of a view file it contains the title and the content of the page.
<div class="view-file"> <h1><?php echo $title; ?></h1> <p><?php echo $content; ?></p> </div>
Calling the "View File"
When we are calling our view file we are first going to create an array of variables that can be passed to our view.
The key of these array values will be turned into variable names in our view. As we only had a title and content variables we can just pass these into our get View() function.
The getView() function takes two parameters, first will be the location of our view file, the second will be the variables sent to the view file.
<?php $vars = array(); $vars['title'] = 'Page Title'; $vars['content'] = 'This is an example of displaying content in the view file'; return $this->getView('view_file.php', $vars); ?>
Get the "View Function"
We have to use the output control functions built into PHP.
First of all we have to control that the view file exists, if the view file doesn't exist then we can't continue with the function so we just return false at that stage.
Next we can take the array of variables and use the extract() function that will turn the array keys into variables.
// Extract the variables to be used by the view
if(!is_null($vars)) {
extract($vars);
}
We need to include the view file into the script so we can use these variables but as we want to return the content of the view file we need to stop PHP from outputting the content of the file, to do this we start the output buffer using ob_start().
ob_start();
With the output buffer started we include the view file, which means it will have access to the variables we have created using the extract() function.
include_once $viewFile;
The view file is not output because we started the ob_start(), to get the content of what is in the buffer we need to use another output control function ob_get_contents(). This will get what would be output and return the contents of the output buffer.
$view = ob_get_contents();
As we have collected all the output and don't want anything else included in the output of the view file then we need to stop the output buffer by using the function ob_end_clean().
Finally we can return the contents of the view file with the HTML populated with the variables we sent to it.
Below is full getView() method.
<?php /** * Get the view file */ public function getView($viewFile, $vars = NULL) { // Check the view file exists if(!file_exists($viewFile)) { return false; } // Extract the variables to be used by the view if(!is_null($vars)) { extract($vars); } ob_start(); include_once $viewFile; $view = ob_get_contents(); ob_end_clean(); return $view; } ?>
original source: paulund.co.uk
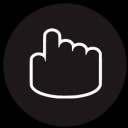
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Examine the 10 key PHP functions I use frequently
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating. 1. Levenshtein This function uses the Levenshtein algorithm to calculate the…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
Loading images by resolution with HTML5
Normally the way to load images in HTML is through the img element to which we pass as a parameter the URL of the image to load. But since HTML5…
PHP - The Singleton Pattern
The Singleton Pattern is one of the GoF (Gang of Four) Patterns. This particular pattern provides a method for limiting the number of instances of an object to just one.…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
Looping through a matrix with JavaScript
We were already talking about how to multiply arrays in JavaScript and we realised that we had not explained something as simple as traversing an array with Javascript. So we…
How to multiply matrices in JavaScript
It may seem strange to want to know how to multiply matrices in JavaScript. But we will see some examples where it is useful to know how to perform this…
JavaScript Formatting Date Tips
Something that seems simple as the treatment of dates can become complex if we don't take into account how to treat them when presenting them to the user. That is…
Validating HTML forms using BULMA and vanilla JavaScript
Today we are going to write about contact forms and how to validate them using JavaScript. The contact form seems to be one of the top features of every basic home…
The State of PHP 8: new features and changes
PHP 8.0 has been released last November 26: let's discover together the main innovations that the new version introduces in this language. PHP is one of the most popular programming languages…