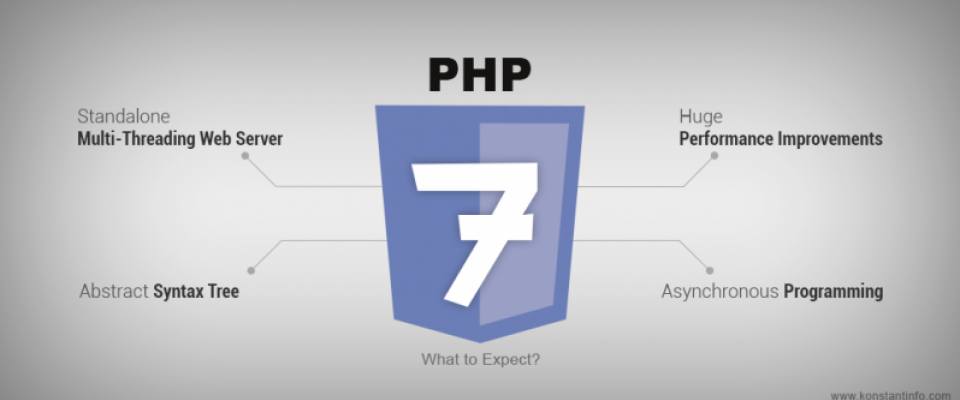
PHP has a cool function that automatically highlights PHP code called highlight_string(); Theoretically this could be used to roll your own code highlighting on a site, rather than rely on JavaScript or some kind of external service to do it. In this article I'll show you the basics of how it works, then extended it with a few tricks. Since JavaScript is so similar to PHP in syntax, we can trick the function into highlighting JavaScript code as well. Then finally how we can bust out some smarts to auto-tab the code.
Basic Usage of the highlight_string function
The highlight_string() function just accepts a string, which must begin with . by default it echos/prints the line.
<?php highlight_string('<?php
$i = 1;
function rockOut() {
alert("wah wah wah");
}
?>'); ?>
The resulting HTML is:
<pre id="code_highlighted"><code><span style="color: #0000BB">
<span style="color: #0000BB"><script type="text/javascript">
</span><span style="color: #007700">if (</span><span style="color: #0000BB">true</span><span style="color: #007700">) {
echo </span><span style="color: #DD0000">'The value is true'</span><span style="color: #007700">;
} else {
echo </span><span style="color: #DD0000">'The value is false'</span><span style="color: #007700">;
}
</span><span style="color: #0000BB"></script></span>
</span>
If you'd rather have that string returned rather than printed, just pass TRUE as a second parameter.
Trick it into highlighting JavaScript
Benjamin Mayo (Darren Beige) put together a PHP function that would trick PHP into highlighting JavaScript code instead of exclusively PHP. Beyond that, it also applies proper tab indentation of code, despite what is present in the file. For example, even if the original code was completely flush left, the output will be nicely indented.
How it works
The indentation occurs by adding line breaks after every brace and semicolon if they are not already there. This puts each statement on it's own line, priming the code. However, the main bulk of the code for indentation happens in the loop itself.
$lineecho = $line;
if (substr_count($line, "\t") != $tab) {
$lineecho = str_replace("\t", "", trim($lineecho));
$lineecho = str_repeat("\t", $tab) . $lineecho;
}
$tab = $tab + substr_count($line, "{") - substr_count($line, "}");
The block works by keeping a count (in the variable $tab) of how many tab characters ("\t") there are on the previous line. The current line is counted for tabs using the substr_count() function. If the two values do not match, the echoed line is padded by the $tab value. This now means that the number of tab characters at the start of the line matches the number in the $tab variable. After this procedure, the new $tab value is calculated by taking the current $tab and adding on the number of opening braces found subtracting the number of closing braces.
The output code is in
tags so the tabs display properly.
Usage of the extended highlight function
Let's say you wanted to highlight a big chunk of JavaScript code that lived in a file. Easy, just include the PHP file/function, grab the contents of that file, and run the function on it.
<?php
include_once('format_javascript.php');
$BigJavaScriptString = file_get_contents('path/to/javascript.js');
echo format_javascript($testBigJS);
?>
So if you want to highlight code this way, you need to get it into a string variable. If you wanted to use this in a CMS, you would need to be able to save and run PHP inside the saved content areas. Or, you'd need to write some fancy regex stuff to parse content and look for particular tags and be able to extract the innards into a variable for highlighting.
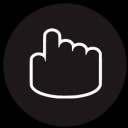
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Examine the 10 key PHP functions I use frequently
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating. 1. Levenshtein This function uses the Levenshtein algorithm to calculate the…
How to upload files to the server using JavaScript
In this tutorial we are going to see how you can upload files to a server using Node.js using JavaScript, which is very common. For example, you might want to…
How to combine multiple objects in JavaScript
In JavaScript you can merge multiple objects in a variety of ways. The most commonly used methods are the spread operator ... and the Object.assign() function. How to copy objects with…
How to Track Flight Status in real-time using the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your…
The Payment Request API: Revolutionizing Online Payments (Part 2)
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features…
The Payment Request API: Revolutionizing Online Payments (Part 1)
The Payment Request API has emerged as the new standard for online payments, transforming the way transactions are conducted on the internet. In this two-part series, we will delve into…
Let's create a Color Picker from scratch with HTML5 Canvas, Javascript and CSS3
HTML5 Canvas is a technology that allows developers to generate real-time graphics and animations using JavaScript. It provides a blank canvas on which graphical elements, such as lines, shapes, images…
How do you stop JavaScript execution for a while: sleep()
A sleep()function is a function that allows you to stop the execution of code for a certain amount of time. Using a function similar to this can be interesting for…
Mastering array sorting in JavaScript: a guide to the sort() function
In this article, I will explain the usage and potential of the sort() function in JavaScript. What does the sort() function do? The sort() function allows you to sort the elements of…
Infinite scrolling with native JavaScript using the Fetch API
I have long wanted to talk about how infinite scroll functionality can be implemented in a list of items that might be on any Web page. Infinite scroll is a technique…
Sorting elements with SortableJS and storing them in localStorage
SortableJS is a JavaScript extension that you will be able to use in your developments to offer your users the possibility to drag and drop elements in order to change…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
