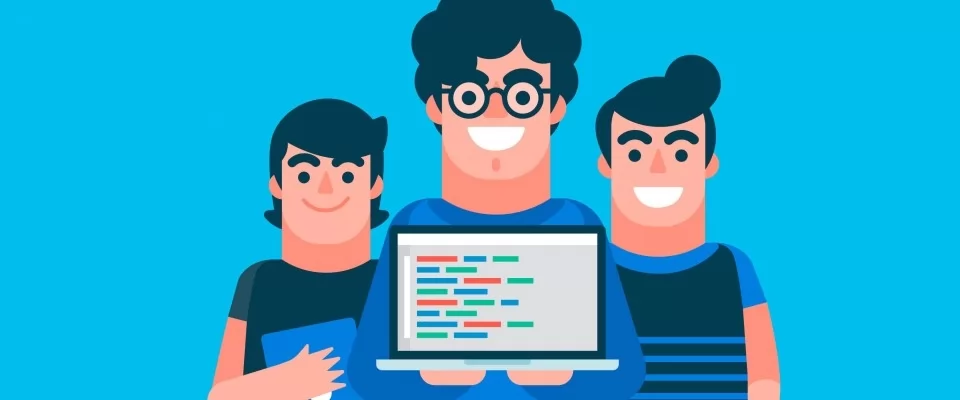
When we started writing about JavaScript, we created a list of every time-saving trick we discovered in the code of other people, on websites, and anywhere other than the tutorials we used.
Since then, we've been contributing to this list and now we are going to share 11 triks that strike us as particularly smart or helpful. This post is meant to be helpful for beginners, but we hope that even intermediate designers of JavaScript will discover something fresh in this list.
Although many of these tricks are useful in any situation, some of them may not be suitable for production-level code, where consistency is often more crucial than concision; we are going to let you judge that!
.
1. Use JSON.stringify
to format JSON Code
You may have used JSON.stringify
before, but did you realize that it can also help to indent your JSON for you?
The stringify() method takes two optional parameters: a replacing function, which you can use to filter the JSON that is displayed, and a space value.
The space
value takes an integer for the number of spaces you want or a string (such as 't
' to insert tabs), and it can make it a lot easier to read fetched JSON data.
console.log(JSON.stringify({ fruit: 'apple', vegetable: 'broccoli' }, null, 't')); // Result: // '{ // "fruit": apple, // "vegetable": broccoli // }'
2. How to Get the Last Item(s) in an Array
The slice() array method can take negative integers and, if so, will take values from the end of the array rather than from the beginning.
let array = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]; console.log(array.slice(-1)); // Result: [9] console.log(array.slice(-2)); // Result: [8, 9] console.log(array.slice(-3)); // Result: [7, 8, 9]
3. How to truncate an Array
If you want to remove values from the end of an array, there are faster options than using splice()
. For instance, if you know the size of the original array, you can redefine its length property as follows:
let array = [a, b, c, d, e, f, g, h, i, l]; array.length = 4; console.log(array); // Result: [a, b, c, d]
4. How to filter unique values
The set
object type introduced in ES6 along with the spread
operator, can be used it to create a new array with only the unique values.
const array = [1, 1, 2, 3, 5, 5, 1] const uniqueArray = [...new Set(array)]; console.log(uniqueArray); // Result: [1, 2, 3, 5]
Thanks to ES6, isolating unique values no longer requires much code!
This trick operates for primitive types of arrays: undefined, null, boolean, string and number. You would need a distinct strategy if you had an array containing items, features or extra arrays.
5. Convert to Boolean
Apart from the normal true and false Boolean values, JavaScript also treats all other values as "true" or "false".
With the exception of 0, "", null, undefined, NaN
and certainly false
, which are 'falsy', all values in JavaScript are 'true', unless otherwise defined.
By using the negative operator !, we can quickly switch between true and false, this will also convert the type to "boolean" .
const isTrue = !0; const isFalse = !1; const alsoFalse = !!0; console.log(isTrue); // Result: true console.log(typeof true); // Result: "boolean"
In conditional statements, this type of transformation can be useful, but, as we have said, in production can become counterproductive.
6. Convert to String
To quickly convert a number to a string, we can use the concatenation operator + followed by an empty set of quotation marks "" .
const val = 23 + ""; console.log(val); // Result: "23" console.log(typeof val); // Result: "string"
7. How to convert a string to a number
The inverse operation can be implemented rapidly by using the +
(addition operator).
let int = "23"; int = +int; console.log(int); // Result: 23 console.log(typeof int); Result: "number"
This can also be used to transform booleans into numbers as shown below:
console.log(+true); // Return: 1 console.log(+false); // Return: 0
By the way, there are many ways to convert a String to a Number. we can think of at least 5 ways to convert a string into a number, including the methods we've already seen.
parseInt(num); // default way (no radix) parseInt(num, 10); // parseInt with radix (decimal) parseFloat(num) // floating point Number(num); // Number constructor ~~num //bitwise not num / 1 // diving by one num * 1 // multiplying by one num - 0 // minus 0 +num // unary operator "+"
The best one in our opinion is to use the Number object, in a non-constructor context (without the new keyword):
const count = Number('1234') //1234
In the case you need to parse a string with decimal separators, use Intl.NumberFormat
instead.
Another good alternative for integers is to call the parseInt()
function:
const count = parseInt('1234', 10) //1234
Try not to forget the second parameter, which is the radix, always 10 for decimal numbers, or the conversion may try to guess the radix and give unexpected results.
parseInt()
tries to get a number from a string that does not only contain a number:
parseInt('10 cats', 10) //10
Warning: if the string does not start with a number, you get NaN (Not a Number):
parseInt("mouse 10", 10) //NaN
In addition, it is not reliable with separators between digits just like Number:
parseInt('10,000', 10) //10 ❌ parseInt('10.00', 10) //10 ✅ (considered decimals, cut) parseInt('10.000', 10) //10 ✅ (considered decimals, cut) parseInt('10.20', 10) //10 ✅ (considered decimals, cut) parseInt('10.81', 10) //10 ✅ (considered decimals, cut) parseInt('10000', 10) //10000 ✅
If you want to play with decimals, use parseFloat()
:
parseFloat('10,000', 10) //10 ❌ parseFloat('10.00', 10) //10 ✅ (considered decimals, cut) parseFloat('10.000', 10) //10 ✅ (considered decimals, cut) parseFloat('10.20', 10) //10.2 ✅ (considered decimals) parseFloat('10.81', 10) //10.81 ✅ (considered decimals) parseFloat('10000', 10) //10000 ✅
Similar to the + , but returns the integer part, is the Math.floor():
Math.floor('10,000') //NaN ✅ Math.floor('10.000') //10 ✅ Math.floor('10.00') //10 ✅ Math.floor('10.20') //10 ✅ Math.floor('10.81') //10 ✅ Math.floor('10000') //10000 ✅
The following is one of the fastest options, behaves like the operator + unary, so it does not perform conversion to an integer if the number is a float.:
'10,000' * 1 //NaN ✅ '10.000' * 1 //10 ✅ '10.00' * 1 //10 ✅ '10.20' * 1 //10.2 ✅ '10.81' * 1 //10.81 ✅ '10000' * 1 //10000 ✅
Business vector created by freepik - www.freepik.com
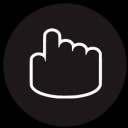
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.