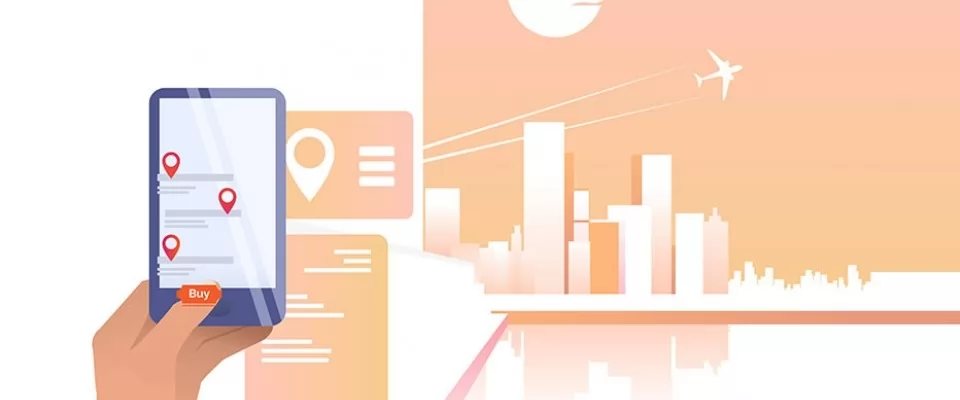
A Guide to utilizing the Flight Tracker API
The Flight Tracker API provides developers with the ability to access real-time flight status, which is extremely useful for integrating historical tracking or live queries of air traffic into your website.
With this API, you can easily track the status of a flight and access airport schedules.
There are several Flight Tracker APIs available to retrieve flight status, and one of the best options is aviationstack. This API offers a simple way to access aviation data globally, including real-time flight status and airport schedules.
Aviationstack tracks every flight worldwide at all times, storing the information in its database and providing real-time flight status through its API. It is a user-friendly REST API that returns responses in JSON format and is compatible with various programming languages such as PHP, Python, Ruby, Node.js, jQuery, Go, and more.
In this tutorial, we will show you how to obtain real-time flight status using the aviationstack Flight Tracker API with PHP.
Obtaining API Credentials
To get started, you need to create an account on aviationstack. Once you are in your dashboard, you can copy the API access key from the "Your API Access Key" section.
API Configuration
We will need the access key to authenticate and access the aviationstack API.
Next, we will build the query using the http_build_query()
function to pass the necessary parameters to the aviationstack API.
Define the access key in the access_key
parameter.
$queryString = http_build_query([ 'access_key' => 'YOUR_ACCESS_KEY' ]);
HTTP GET Request
To retrieve flight data, we will make an HTTP GET
request to the aviationstack API using cURL.
// API URL with the query string $apiURL = sprintf('%s?%s', 'https://api.aviationstack.com/v1/flights', $queryString); // Initialize cURL $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $apiURL); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the API request $api_response = curl_exec($ch); // Close cURL curl_close($ch);
It is important to ensure that the API call is secure, so we should use the URL with https
:
Flight Status and General Information
After making the API call, we will receive the current flight status and related information in JSON format. Initially, the aviationstack API provides the following geolocation data:
- Flight date (flight_date)
- Flight status (flight_status)
- Departure and arrival information (departure/arrival)
- airport
- timezone
- iata
- icao
- terminal
- gate
- delay
- scheduled
- estimated
- actual
- estimated_runway
- actual_runway
- Airline information (airline)
- name
- iata
- icao
- Flight information (flight)
- number
- iata
- icao
- codeshared
- Aircraft information (aircraft)
- registration
- iata
- icao
- icao24
- Live data (live)
- updated
- latitude
- longitude
- altitude
- direction
- speed_horizontal
- speed_vertical
- is_ground
By using the json_decode()
function, we can convert the obtained JSON data into a PHP array.
Here's an example of how to extract flight information using the aviationstack API:
$api_result = json_decode($api_response, true);
Below is the complete code to retrieve global flight information using the aviationstack API with PHP:
// Define the API access key $queryString = http_build_query([ 'access_key' => 'YOUR_ACCESS_KEY', 'limit' => 10 ]); // API URL with the query string $apiURL = sprintf('%s?%s', 'https://api.aviationstack.com/v1/flights', $queryString); // Initialize cURL $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $apiURL); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // Execute the API request $api_response = curl_exec($ch); // Close cURL curl_close($ch); // Convert the JSON into an array $api_result = json_decode($api_response, true); // Display flight data foreach ($api_result['data'] as $flight) { if (!$flight['live']['is_ground']) { echo sprintf("%s flight %s from %s (%s) to %s (%s) is in the air.", $flight['airline']['name'], $flight['flight']['iata'], $flight['departure']['airport'], $flight['departure']['iata'], $flight['arrival']['airport'], $flight['arrival']['iata'] ), PHP_EOL; echo '<br/>'; } } ?>
This code allows you to retrieve updated flight information using the aviationstack API. Make sure to replace ' YOUR_ACCESS_KEY
' with your provided personal access key from aviationstack.
You can integrate this code into your PHP application to display real-time flight status and related details on your website.
Note: It's important to handle error cases, such as when the API response is not successful or when there are no flight data available. You can add error handling and additional logic as per your application requirements.
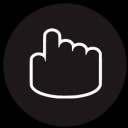
Janeth Kent
Licenciada en Bellas Artes y programadora por pasión. Cuando tengo un rato retoco fotos, edito vídeos y diseño cosas. El resto del tiempo escribo en MA-NO WEB DESIGN AND DEVELOPMENT.
Related Posts
Examine the 10 key PHP functions I use frequently
PHP never ceases to surprise me with its built-in capabilities. These are a few of the functions I find most fascinating. 1. Levenshtein This function uses the Levenshtein algorithm to calculate the…
What is a JWT token and how does it work?
JWT tokens are a standard used to create application access tokens, enabling user authentication in web applications. Specifically, it follows the RFC 7519 standard. What is a JWT token A JWT token…
PHP - The Singleton Pattern
The Singleton Pattern is one of the GoF (Gang of Four) Patterns. This particular pattern provides a method for limiting the number of instances of an object to just one.…
How to Send Email from an HTML Contact Form
In today’s article we will write about how to make a working form that upon hitting that submit button will be functional and send the email (to you as a…
The State of PHP 8: new features and changes
PHP 8.0 has been released last November 26: let's discover together the main innovations that the new version introduces in this language. PHP is one of the most popular programming languages…
HTTP Cookies: how they work and how to use them
Today we are going to write about the way to store data in a browser, why websites use cookies and how they work in detail. Continue reading to find out how…
The most popular Array Sorting Algorithms In PHP
There are many ways to sort an array in PHP, the easiest being to use the sort() function built into PHP. This sort function is quick but has it's limitations,…
MySQL 8.0 is now fully supported in PHP 7.4
MySQL and PHP is a love story that started long time ago. However the love story with MySQL 8.0 was a bit slower to start… but don’t worry it rules…
A roadmap to becoming a web developer in 2019
There are plenty of tutorials online, which won't cost you a cent. If you are sufficiently self-driven and interested, you have no difficulty training yourself. The point to learn coding…
Working with JSON in JavaScript
JSON is short for JavaScript Object Notation and an easy - to - access way to store information. In short, it gives us a collection of human - readable data…
10 PHP code snippets to work with dates
Here we have some set of Useful PHP Snippets, which are useful for PHP Developers. In this tutorial we'll show you the 10 PHP date snippets you can use on…
8 Free PHP Books to Read in Summer 2018
In this article, we've listed 8 free PHP books that can help you to learn new approaches to solving problems and keep your skill up to date. Practical PHP Testing This book…
